Can there be another implementation of this program, without empty constructor? Can someone write it ? #include #include using namespace std; //class lecturer class lecturer{ //data members string name; string department; public: //empty constructor lecturer(){ } //constructor lecturer(string n, string d){ name = n; department = d; } //setter for name void set_name(string n){ name = n; } //setter for department void set_dep(string d){ department = d; } //getter for name string get_name(){ return name; } //getter for department string get_dep(){ return department; } string toString(){ string des = "Name :" + get_name() + " Department: " + get_dep(); return des; } }; //class lecture class Lecture{ //data members private: string subject; string weekday; int hour; bool online; lecturer l; public: //empty constructor Lecture(){ } //constructor Lecture(string sub, string w, int h, bool o, lecturer le){ subject = sub; weekday = w; hour = h; online = o; l.set_name(le.get_name()); l.set_dep(le.get_dep()); } //setter for subject void set_sub(string sub){ subject = sub; } //setter for weekday void set_weekd(string w){ weekday = w; } //setter for hour void set_hour(int h){ hour = h; } //setter for online void set_on(bool on){ online = on; } //setter for lecturer void set_lec(lecturer le){ l.set_name(le.get_name()); l.set_dep(le.get_dep()); } //getter for subject string get_sub(){ return subject; } //getter for weekday string get_weekd(){ return weekday; } //getter for hour int get_hour(){ return hour; } //getter for online bool get_on(){ return online; } //function to check whether the lecture starts at 7-11 bool isItMorning(){ if (hour >=7 && hour <=11) return true; else return false; } //function to display description string toString(){ string des = l.toString() + " Subject : " + subject + " Day: " + weekday + " Time : " + to_string(hour) + " Online: " + to_string(online); return des; } }; int main(){ //objects of lecturer lecturer l1("xyz", "CS"); lecturer l2("abc", "maths"); //calling toString function cout<
Can there be another implementation of this program, without empty constructor? Can someone write it ?
#include <iostream>
#include <fstream>
using namespace std;
//class lecturer
class lecturer{
//data members
string name;
string department;
public:
//empty constructor
lecturer(){
}
//constructor
lecturer(string n, string d){
name = n;
department = d;
}
//setter for name
void set_name(string n){
name = n;
}
//setter for department
void set_dep(string d){
department = d;
}
//getter for name
string get_name(){
return name;
}
//getter for department
string get_dep(){
return department;
}
string toString(){
string des = "Name :" + get_name() + " Department: " + get_dep();
return des;
}
};
//class lecture
class Lecture{
//data members
private:
string subject;
string weekday;
int hour;
bool online;
lecturer l;
public:
//empty constructor
Lecture(){
}
//constructor
Lecture(string sub, string w, int h, bool o, lecturer le){
subject = sub;
weekday = w;
hour = h;
online = o;
l.set_name(le.get_name());
l.set_dep(le.get_dep());
}
//setter for subject
void set_sub(string sub){
subject = sub;
}
//setter for weekday
void set_weekd(string w){
weekday = w;
}
//setter for hour
void set_hour(int h){
hour = h;
}
//setter for online
void set_on(bool on){
online = on;
}
//setter for lecturer
void set_lec(lecturer le){
l.set_name(le.get_name());
l.set_dep(le.get_dep());
}
//getter for subject
string get_sub(){
return subject;
}
//getter for weekday
string get_weekd(){
return weekday;
}
//getter for hour
int get_hour(){
return hour;
}
//getter for online
bool get_on(){
return online;
}
//function to check whether the lecture starts at 7-11
bool isItMorning(){
if (hour >=7 && hour <=11)
return true;
else
return false;
}
//function to display description
string toString(){
string des = l.toString() + " Subject : " + subject + " Day: " + weekday
+ " Time : " + to_string(hour) + " Online: " + to_string(online);
return des;
}
};
int main(){
//objects of lecturer
lecturer l1("xyz", "CS");
lecturer l2("abc", "maths");
//calling toString function
cout<<l1.toString()<<endl;
cout<<l2.toString()<<endl;
//array of Lecture objects
Lecture L[5];
//initialising the array
L[0] = Lecture("Java","Monday",8,true,l1);
L[1] = Lecture("C++","Tuesday",9,false,l1);
L[2] = Lecture("DS","Monday",10,true,l1);
L[3] = Lecture("Calculus","Monday",7,true,l2);
L[4] = Lecture("Geometry","Wednesday",8,false,l2);
cout<<endl;
//ofstream object
ofstream out;
//open output file
out.open("detail.txt");
//printing the details to the screen and the output file
for(int i=0;i<5;i++)
{
cout<<L[i].toString()<<endl;
out<<L[i].toString()<<endl;
}
//close output file
out.close();
return 0;
}

C++ Empty creator necessity depends upon category style necessities. we all know that C++ category creator is termed after we produce AN object of a category.
If a category isn't needed to initialize its knowledge member or doesn't contain knowledge member, there's no ought to write empty creator expressly. On category object creation, default creator implicitly known as are enough.
Step by step
Solved in 2 steps

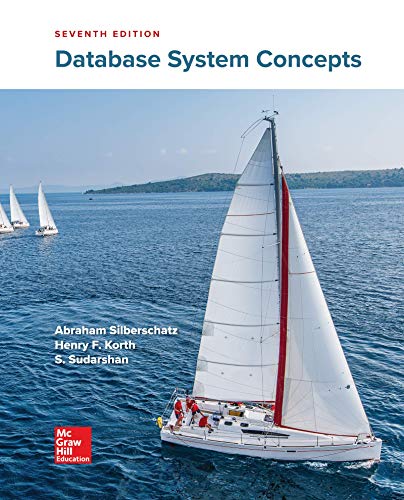
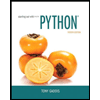
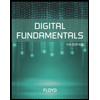
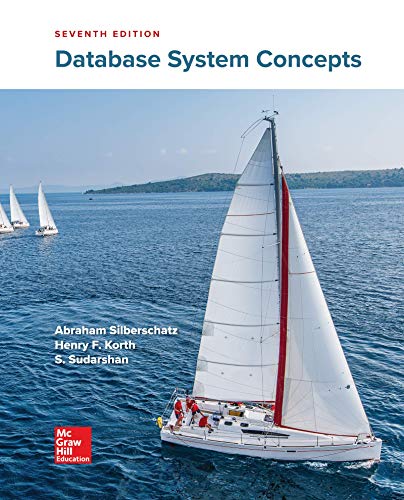
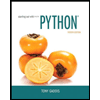
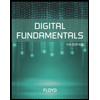
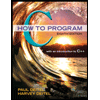
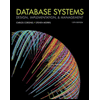
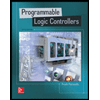