The main function, 1. Prompts the user to enter a capacity, and then declares a pointer groups pointing to a dynamic array of Student with the entered capacity. 2. Prompts the user to enter a student's id and name. If the entered id is not 0, creates a Student object newStudent with the entered id and name values. 3. Adds the object newStudent in the dynamic array groups. You may declare an int variable size to count the number of student objects stored in the dynamic array. 4. Repeats the step 2 and 3 until the entered student id is O. 5. Displays the student information in groups, that is, print out the student id and name in the dynamic array. The expected result: Enter the capacity of dynamic array (int): 20 Enter the student id (int) and name (string): 1101 Taylor Enter the student id (int) and name (string): 1102 Smith Enter the student id (int) and name (string): 1103 Alice Enter the student id (int) and name (string): 1104 Tom Enter the student id (int) and name (string): 0 noname The students are: 1101 Taylor 1102 Smith 1103 Alice 1104 Tom Lab Exercise Write C++ programs that • Implement the class Student in the file Student.cpp #ifndef STUDENT_H #define STUDENT_H #include #include class Student { public: // Default constructor Student():id(0), name("") { } // Creates a student with the specified id and name. Student(int idvalue, const string & namevalue) { } // Returns the student name. string get_name() const { } // Returns the student id. int get_id () const { } // Sets the student name. void set_name(const string& namevalue) { } // Sets the student id. void set_id(int idvalue) } // Prints the student id and name. void print_student () const { cout << id << " <> capacity; // Add your code ... return 0;
The main function, 1. Prompts the user to enter a capacity, and then declares a pointer groups pointing to a dynamic array of Student with the entered capacity. 2. Prompts the user to enter a student's id and name. If the entered id is not 0, creates a Student object newStudent with the entered id and name values. 3. Adds the object newStudent in the dynamic array groups. You may declare an int variable size to count the number of student objects stored in the dynamic array. 4. Repeats the step 2 and 3 until the entered student id is O. 5. Displays the student information in groups, that is, print out the student id and name in the dynamic array. The expected result: Enter the capacity of dynamic array (int): 20 Enter the student id (int) and name (string): 1101 Taylor Enter the student id (int) and name (string): 1102 Smith Enter the student id (int) and name (string): 1103 Alice Enter the student id (int) and name (string): 1104 Tom Enter the student id (int) and name (string): 0 noname The students are: 1101 Taylor 1102 Smith 1103 Alice 1104 Tom Lab Exercise Write C++ programs that • Implement the class Student in the file Student.cpp #ifndef STUDENT_H #define STUDENT_H #include #include class Student { public: // Default constructor Student():id(0), name("") { } // Creates a student with the specified id and name. Student(int idvalue, const string & namevalue) { } // Returns the student name. string get_name() const { } // Returns the student id. int get_id () const { } // Sets the student name. void set_name(const string& namevalue) { } // Sets the student id. void set_id(int idvalue) } // Prints the student id and name. void print_student () const { cout << id << " <> capacity; // Add your code ... return 0;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write the full C++ code for the expected output provided in the second screenshot

Transcribed Image Text:The main function,
1. Prompts the user to enter a capacity, and then declares a pointer groups pointing to a dynamic array of Student with the entered capacity.
2. Prompts the user to enter a student's id and name. If the entered id is not 0, creates a Student object newStudent with the entered id and name values.
3. Adds the object newStudent in the dynamic array groups. You may declare an int variable size to count the number of student objects stored in the dynamic array.
4. Repeats the step 2 and 3 until the entered student id is O.
5. Displays the student information in groups, that is, print out the student id and name in the dynamic array.
The expected result:
Enter the capacity of dynamic array (int): 20
Enter the student id (int) and name (string): 1101 Taylor
Enter the student id (int) and name (string): 1102 Smith
Enter the student id (int) and name (string): 1103 Alice
Enter the student id (int) and name (string): 1104 Tom
Enter the student id (int) and name (string): 0 noname
The students are:
1101 Taylor
1102 Smith
1103 Alice
1104 Tom

Transcribed Image Text:Lab Exercise
Write C++ programs that
•
Implement the class Student in the file Student.cpp
#ifndef STUDENT_H
#define STUDENT_H
#include <string>
#include <iostream>
class Student
{
public:
// Default constructor
Student():id(0), name("")
{
}
// Creates a student with the specified id and name.
Student(int idvalue, const string & namevalue)
{
}
// Returns the student name.
string get_name() const
{
}
// Returns the student id.
int get_id () const
{
}
// Sets the student name.
void set_name(const string& namevalue)
{
}
// Sets the student id.
void set_id(int idvalue)
}
// Prints the student id and name.
void print_student () const
{
cout << id <<
"
<<name <<endl;
}
private:
};
// student name
string name;
// student id
int id;
#endif
The test program lab02.cpp contains the main function.
#include "Student.cpp'
int main()
{
"
// Declare variables
int capacity = 0, size = 0, id =0;
string name;
cout<<"Enter the capacity: ";
cin>> capacity;
// Add your code ...
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
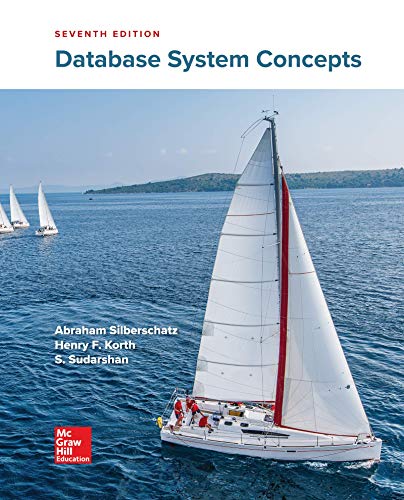
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
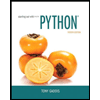
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
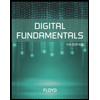
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
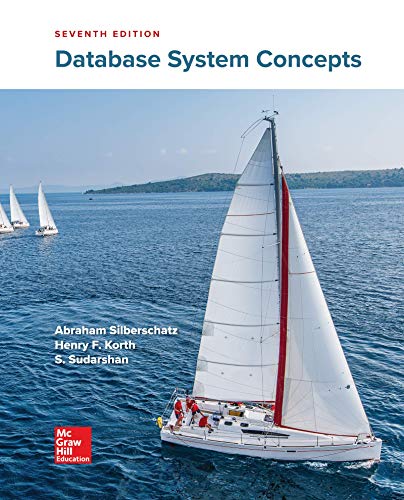
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
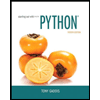
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
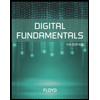
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
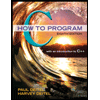
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
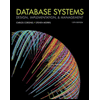
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
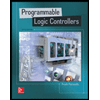
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education