Add a main function () in main.py and 3 other functions with 3 parameters and 2 arguments in a seperate module file, while using return statements to return the values. Use 3 predefined modules in your code and add a menu to the main.py file in the code below: main.py Code: from TicTac import game if __name__ == "__main__": game() TicTac.py code: import random from display import printBoard theBoard = {'7': '7' , '8': '8' , '9': '9' , '4': '4' , '5': '5' , '6': '6' , '1': '1' , '2': '2' , '3': '3' } board_keys = [] for key in theBoard: board_keys.append(key) #main function which will have all the gameplay functionality. def game(): turn = 'X' count = 0 while(True): printBoard(theBoard) move =str(random.randint(1,9)) print("It's " + turn + " turn and location is "+move) if theBoard[move] == move: theBoard[move] = turn count += 1 else: print("That place is already filled.\nMove to which place?") continue # To check if player X or O has won,for every move after 5 moves. if count >= 5: if theBoard['7'] == theBoard['8'] == theBoard['9'] != ' ': # across the top printBoard(theBoard) print("\nGame Over.\n") print(" **** " +turn + " won. ****") break elif theBoard['4'] == theBoard['5'] == theBoard['6'] != ' ': # across the middle printBoard(theBoard) print("\nGame Over.\n") print(" **** " +turn + " won. ****") break elif theBoard['1'] == theBoard['2'] == theBoard['3'] != ' ': # across the bottom printBoard(theBoard) print("\nGame Over.\n") print(" **** " +turn + " won. ****") break elif theBoard['1'] == theBoard['4'] == theBoard['7'] != ' ': # down the left side printBoard(theBoard) print("\nGame Over.\n") print(" **** " +turn + " won. ****") break elif theBoard['2'] == theBoard['5'] == theBoard['8'] != ' ': # down the middle printBoard(theBoard) print("\nGame Over.\n") print(" **** " +turn + " won. ****") break elif theBoard['3'] == theBoard['6'] == theBoard['9'] != ' ': # down the right side printBoard(theBoard) print("\nGame Over.\n") print(" **** " +turn + " won. ****") break elif theBoard['7'] == theBoard['5'] == theBoard['3'] != ' ': # diagonal printBoard(theBoard) print("\nGame Over.\n") print(" **** " +turn + " won. ****") break elif theBoard['1'] == theBoard['5'] == theBoard['9'] != ' ': # diagonal printBoard(theBoard) print("\nGame Over.\n") print(" **** " +turn + " won. ****") break # If neither X nor O wins and the board is full, we'll declare the result as 'tie'. if count == 9: print("\nGame Over.\n") print("It's a Tie!!") break # Now we have to change the player after every move. if turn =='X': turn = 'O' else: turn = 'X' display.py code: '''To print the updated board after every move in the game''' def printBoard(board): print(board['7'] + '|' + board['8'] + '|' + board['9']) print('-+-+-') print(board['4'] + '|' + board['5'] + '|' + board['6']) print('-+-+-') print(board['1'] + '|' + board['2'] + '|' + board['3'])
Add a main function () in main.py and 3 other functions with 3 parameters and 2 arguments in a seperate module file, while using return statements to return the values. Use 3 predefined modules in your code and add a menu to the main.py file in the code below:
main.py Code:
from TicTac import game
if __name__ == "__main__":
game()
TicTac.py code:
import random
from display import printBoard
theBoard = {'7': '7' , '8': '8' , '9': '9' ,
'4': '4' , '5': '5' , '6': '6' ,
'1': '1' , '2': '2' , '3': '3' }
board_keys = []
for key in theBoard:
board_keys.append(key)
#main function which will have all the gameplay functionality.
def game():
turn = 'X'
count = 0
while(True):
printBoard(theBoard)
move =str(random.randint(1,9))
print("It's " + turn + " turn and location is "+move)
if theBoard[move] == move:
theBoard[move] = turn
count += 1
else:
print("That place is already filled.\nMove to which place?")
continue
# To check if player X or O has won,for every move after 5 moves.
if count >= 5:
if theBoard['7'] == theBoard['8'] == theBoard['9'] != ' ': # across the top
printBoard(theBoard)
print("\nGame Over.\n")
print(" **** " +turn + " won. ****")
break
elif theBoard['4'] == theBoard['5'] == theBoard['6'] != ' ': # across the middle
printBoard(theBoard)
print("\nGame Over.\n")
print(" **** " +turn + " won. ****")
break
elif theBoard['1'] == theBoard['2'] == theBoard['3'] != ' ': # across the bottom
printBoard(theBoard)
print("\nGame Over.\n")
print(" **** " +turn + " won. ****")
break
elif theBoard['1'] == theBoard['4'] == theBoard['7'] != ' ': # down the left side
printBoard(theBoard)
print("\nGame Over.\n")
print(" **** " +turn + " won. ****")
break
elif theBoard['2'] == theBoard['5'] == theBoard['8'] != ' ': # down the middle
printBoard(theBoard)
print("\nGame Over.\n")
print(" **** " +turn + " won. ****")
break
elif theBoard['3'] == theBoard['6'] == theBoard['9'] != ' ': # down the right side
printBoard(theBoard)
print("\nGame Over.\n")
print(" **** " +turn + " won. ****")
break
elif theBoard['7'] == theBoard['5'] == theBoard['3'] != ' ': # diagonal
printBoard(theBoard)
print("\nGame Over.\n")
print(" **** " +turn + " won. ****")
break
elif theBoard['1'] == theBoard['5'] == theBoard['9'] != ' ': # diagonal
printBoard(theBoard)
print("\nGame Over.\n")
print(" **** " +turn + " won. ****")
break
# If neither X nor O wins and the board is full, we'll declare the result as 'tie'.
if count == 9:
print("\nGame Over.\n")
print("It's a Tie!!")
break
# Now we have to change the player after every move.
if turn =='X':
turn = 'O'
else:
turn = 'X'
display.py code:
'''To print the updated board after every move in the game'''
def printBoard(board):
print(board['7'] + '|' + board['8'] + '|' + board['9'])
print('-+-+-')
print(board['4'] + '|' + board['5'] + '|' + board['6'])
print('-+-+-')
print(board['1'] + '|' + board['2'] + '|' + board['3'])

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 7 images

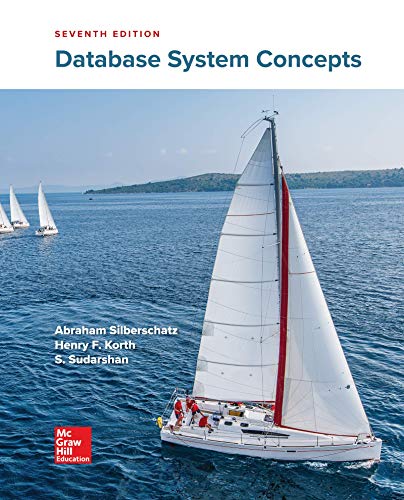
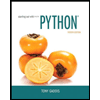
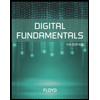
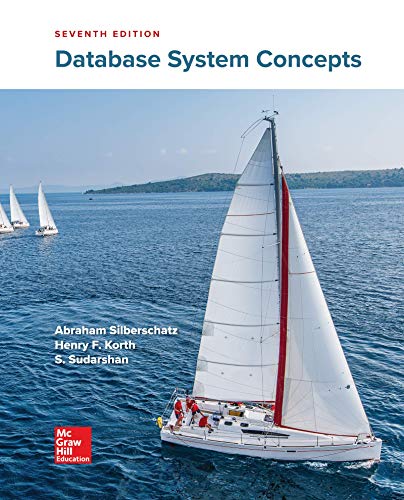
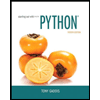
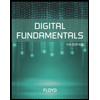
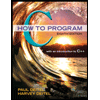
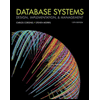
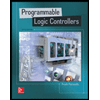