A3Q3.c - You are to write a C program that implements the following disk scheduling algorithms: a. FCFS [10 marks] b. SCAN [10 marks] c. C-SCAN [10 marks] d. SSTF [10 marks] e. LOOK [10 marks] f. C-LOOK [10 marks] • Your program will service a disk with 300 cylinders numbered 0 to 299. • • • • The program will service the requests (a list of 20 cylinder numbers) given in the file request.bin. This file contains (4 byte) integer values representing requests ranging from 0-299. Your program will take the initial position of the disk head as the first command line argument and the direction of the head as the second command line argument. It will then output the requests in the order in which they are serviced, and the total amount of head movements required by each algorithm. In particular, your program needs to do the following: Your program should take two command line arguments a) First command line argument - initial position of the disk head (an integer value) b) Second command line argument - direction of the head (LEFT or RIGHT) 2) Read the requests from the binary file (requests.bin), and store these requests in an integer array (for example: requests), after which you need to close the file. 3) Write functions for each disk scheduling algorithm. 4) The scheduling algorithms will service requests present in the requests array. 5) Since the requests are serviced in an order by the scheduling algorithms, you may need to first sort the requests in the requests array in an increasing order and store the sorted requests in another integer array (for example: sortedrequests). These algorithms will then service the requests in the sortedrequests array and compute the head movements. 6) You can use any sorting algorithm of your choice. You should simply analysis whether your sorting algorithm is efficient or not. 7) Your program should output the following (see sample output given below): a) Requests in the order in which they are serviced by each algorithm. b) Total amount of head movement incurred by each algorithm while servicing all the requests.
A3Q3.c - You are to write a C program that implements the following disk scheduling algorithms: a. FCFS [10 marks] b. SCAN [10 marks] c. C-SCAN [10 marks] d. SSTF [10 marks] e. LOOK [10 marks] f. C-LOOK [10 marks] • Your program will service a disk with 300 cylinders numbered 0 to 299. • • • • The program will service the requests (a list of 20 cylinder numbers) given in the file request.bin. This file contains (4 byte) integer values representing requests ranging from 0-299. Your program will take the initial position of the disk head as the first command line argument and the direction of the head as the second command line argument. It will then output the requests in the order in which they are serviced, and the total amount of head movements required by each algorithm. In particular, your program needs to do the following: Your program should take two command line arguments a) First command line argument - initial position of the disk head (an integer value) b) Second command line argument - direction of the head (LEFT or RIGHT) 2) Read the requests from the binary file (requests.bin), and store these requests in an integer array (for example: requests), after which you need to close the file. 3) Write functions for each disk scheduling algorithm. 4) The scheduling algorithms will service requests present in the requests array. 5) Since the requests are serviced in an order by the scheduling algorithms, you may need to first sort the requests in the requests array in an increasing order and store the sorted requests in another integer array (for example: sortedrequests). These algorithms will then service the requests in the sortedrequests array and compute the head movements. 6) You can use any sorting algorithm of your choice. You should simply analysis whether your sorting algorithm is efficient or not. 7) Your program should output the following (see sample output given below): a) Requests in the order in which they are serviced by each algorithm. b) Total amount of head movement incurred by each algorithm while servicing all the requests.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter8: I/o Streams And Data Files
Section8.4: File Streams As Function Arguments
Problem 3E
Related questions
Question
![A3Q3.c - You are to write a C program that implements the following disk scheduling
algorithms:
a. FCFS [10 marks]
b. SCAN [10 marks]
c. C-SCAN [10 marks]
d. SSTF [10 marks]
e. LOOK [10 marks]
f. C-LOOK [10 marks]
•
Your program will service a disk with 300 cylinders numbered 0 to 299.
•
•
•
•
The program will service the requests (a list of 20 cylinder numbers) given in the file
request.bin.
This file contains (4 byte) integer values representing requests ranging from 0-299.
Your program will take the initial position of the disk head as the first command line
argument and the direction of the head as the second command line argument.
It will then output the requests in the order in which they are serviced, and the total
amount of head movements required by each algorithm.
In particular, your program needs to do the following:
Your program should take two command line arguments
a) First command line argument - initial position of the disk head (an integer value)
b) Second command line argument - direction of the head (LEFT or RIGHT)
2) Read the requests from the binary file (requests.bin), and store these requests in
an integer array (for example: requests), after which you need to close the file.
3) Write functions for each disk scheduling algorithm.
4) The scheduling algorithms will service requests present in the requests array.
5) Since the requests are serviced in an order by the scheduling algorithms, you may
need to first sort the requests in the requests array in an increasing order and
store the sorted requests in another integer array (for example: sortedrequests).
These algorithms will then service the requests in the sortedrequests array and
compute the head movements.
6) You can use any sorting algorithm of your choice. You should simply analysis
whether your sorting algorithm is efficient or not.
7) Your program should output the following (see sample output given below):
a) Requests in the order in which they are serviced by each algorithm.
b) Total amount of head movement incurred by each algorithm while servicing all
the requests.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F92e5c125-0162-44af-aecb-b4cf9027a9d3%2Fd2bbe2ae-4268-4fff-874f-db466c5d358c%2F3m8czqs_processed.png&w=3840&q=75)
Transcribed Image Text:A3Q3.c - You are to write a C program that implements the following disk scheduling
algorithms:
a. FCFS [10 marks]
b. SCAN [10 marks]
c. C-SCAN [10 marks]
d. SSTF [10 marks]
e. LOOK [10 marks]
f. C-LOOK [10 marks]
•
Your program will service a disk with 300 cylinders numbered 0 to 299.
•
•
•
•
The program will service the requests (a list of 20 cylinder numbers) given in the file
request.bin.
This file contains (4 byte) integer values representing requests ranging from 0-299.
Your program will take the initial position of the disk head as the first command line
argument and the direction of the head as the second command line argument.
It will then output the requests in the order in which they are serviced, and the total
amount of head movements required by each algorithm.
In particular, your program needs to do the following:
Your program should take two command line arguments
a) First command line argument - initial position of the disk head (an integer value)
b) Second command line argument - direction of the head (LEFT or RIGHT)
2) Read the requests from the binary file (requests.bin), and store these requests in
an integer array (for example: requests), after which you need to close the file.
3) Write functions for each disk scheduling algorithm.
4) The scheduling algorithms will service requests present in the requests array.
5) Since the requests are serviced in an order by the scheduling algorithms, you may
need to first sort the requests in the requests array in an increasing order and
store the sorted requests in another integer array (for example: sortedrequests).
These algorithms will then service the requests in the sortedrequests array and
compute the head movements.
6) You can use any sorting algorithm of your choice. You should simply analysis
whether your sorting algorithm is efficient or not.
7) Your program should output the following (see sample output given below):
a) Requests in the order in which they are serviced by each algorithm.
b) Total amount of head movement incurred by each algorithm while servicing all
the requests.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 3 images

Recommended textbooks for you
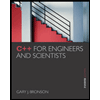
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
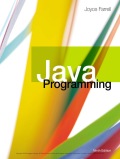
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
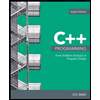
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
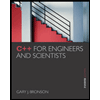
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
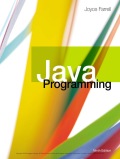
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
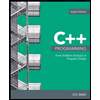
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
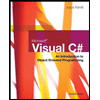
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
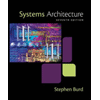
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning