1 // Rectangle.cpp 2 using namespace std; 3 class Rectangle 4 { public: // Declare public methods here dpib;e ummary i this lab, you create a programmer-defined class and then use it in a C++ rogram. The program should create two Rectangle objects and find their rea and perimeter. 7 8 private: // Create length and width here double length; istructions |10 11 double width; 1. Ensure the class file named Rectangle.cpp is open in your editor. 12 }; 13 2. In the Rectangle class, create two private attributes named length and width. Both length and width should be data type double. 14 void Rectangle::setLength(double len) 15 { 16 3. Write public set methods to set the values for length and width. 17 } 18 4. Write public get methods to retrieve the values for length and 19 void Rectangle::setWidth(double wid) 20 { width. 5. Write a public calculateArea() method and a public 21 // write setWidth here 22 } calculatePerimeter() to calculate and return the area of 23 the rectangle and the perimeter of the rectangle. 24 double Rectangle::getLength() 25 { 6. Open the file named MyRectangleClassProgram.cpp. 26 // write getLength here 7. In the MyRectangleClassProgram, create two Rectangle objects named 27 } 28 rectanglel and rectangle2 using the default constructor as you | 29 double Rectangle::getWidth() 30 { // write getWidth here 32 } saw in MyEmployeeClassProgram.cpp. 31 8. Set the length of rectanglel to 10.0 and the width to 5.0. Set the length of rectangle2 to 7.0 and the width to 3.O. 33 34 double Rectangle::calculateArea() 35 { 9. Print the value of rectanglel's perimeter and area, and then print the value of rectangle2's perimeter and area. 36 // write calculateArea here 37 } O. Execute the program by clicking the Run button at the bottom of the 38 39 double Rectangle::calculatePerimeter() 40 { screen 41 // write calculatePerimeter here 42 }
1 // Rectangle.cpp 2 using namespace std; 3 class Rectangle 4 { public: // Declare public methods here dpib;e ummary i this lab, you create a programmer-defined class and then use it in a C++ rogram. The program should create two Rectangle objects and find their rea and perimeter. 7 8 private: // Create length and width here double length; istructions |10 11 double width; 1. Ensure the class file named Rectangle.cpp is open in your editor. 12 }; 13 2. In the Rectangle class, create two private attributes named length and width. Both length and width should be data type double. 14 void Rectangle::setLength(double len) 15 { 16 3. Write public set methods to set the values for length and width. 17 } 18 4. Write public get methods to retrieve the values for length and 19 void Rectangle::setWidth(double wid) 20 { width. 5. Write a public calculateArea() method and a public 21 // write setWidth here 22 } calculatePerimeter() to calculate and return the area of 23 the rectangle and the perimeter of the rectangle. 24 double Rectangle::getLength() 25 { 6. Open the file named MyRectangleClassProgram.cpp. 26 // write getLength here 7. In the MyRectangleClassProgram, create two Rectangle objects named 27 } 28 rectanglel and rectangle2 using the default constructor as you | 29 double Rectangle::getWidth() 30 { // write getWidth here 32 } saw in MyEmployeeClassProgram.cpp. 31 8. Set the length of rectanglel to 10.0 and the width to 5.0. Set the length of rectangle2 to 7.0 and the width to 3.O. 33 34 double Rectangle::calculateArea() 35 { 9. Print the value of rectanglel's perimeter and area, and then print the value of rectangle2's perimeter and area. 36 // write calculateArea here 37 } O. Execute the program by clicking the Run button at the bottom of the 38 39 double Rectangle::calculatePerimeter() 40 { screen 41 // write calculatePerimeter here 42 }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:1 // Rectangle.cpp
2 using namespace std;
3 class Rectangle
ummary
i this lab, you create a programmer-defined class and then use it in a C++
public:
// Declare public methods here
dpib;e|
private:
// Create length and width here
double length;
double width;
rogram. The program should create two Rectangle objects and find their
rea and perimeter.
8
istructions
10
11
1. Ensure the class file named Rectangle.cpp is open in your editor.
12 };
13
2. In the Rectangle class, create two private attributes named length
14 void Rectangle::setLength(double len)
15 {
and width . Both length and width should be data type double.
16
3. Write public set methods to set the values for length and width.
17 }
18
4. Write public get methods to retrieve the values for length and
19 void Rectangle::setWidth(double wid)
20 {
width.
5. Write a public calculateArea() method and a public
// write setWidth here
21
22 }
calculatePerimeter() method to calculate and return the area of
23
the rectangle and the perimeter of the rectangle.
24 double Rectangle::getLength()
25 {
6. Open the file named MyRectangleClassProgram.cpp.
26
// write getLength here
7. In the MyRectangleClassProgram, create two Rectangle objects named
27 }
28
rectanglel and rectangle2 using the default constructor as you
29 double Rectangle::getWidth()
30 {
saw in MyEmployeeClassProgram.cpp.
31
// write getWidth here
8. Set the length of rectanglel to 10.0 and the width to 5.0. Set the
32 }
length of rectangle2 to 7.0 and the width to 3.0.
33
34 double Rectangle::calculateArea()
35 {
9. Print the value of rectanglel's perimeter and area, and then print
the value of rectangle2's perimeter and area.
36
// write calculateArea here
37 }
O. Execute the program by clicking the Run button at the bottom of the
38
39 double Rectangle::calculatePerimeter()
40 {
screen
41
// write calculatePerimeter here
42 }

Transcribed Image Text:MyRectangleClassPro.. Rectangle.cpp
+
1 // This program uses the programmer-defined Rectangle class.
2 #include "Rectangle.cpp"
3 #include <iostream>
4 using namespace std;
5 int main()
6 {
7
Rectangle rectanglel;
Rectangle rectangle2;
8
rectanglel.setLength(10.0);
rectanglel.setWidth(5.0);
rectangle2.setLength(7.0);
rectangle2.setWidth(3.0);
2
3
4
6
cout <« "Perimeter of rectanglel is " « rectanglel.calculatePerimeter() « endl;
cout <« "Area of rectanglel is " <« rectanglel.calculateArea() « endl;
cout <« "Perimeter of rectangle2 is " « rectangle2.calculatePerimeter() <« endl;
cout <« "Area of rectangle2 is " <« rectangle2.calculateArea() <« endl;
8
1
2
3 }
:4
return Ø;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
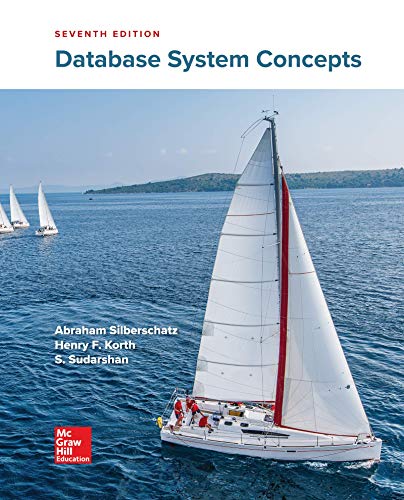
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
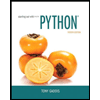
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
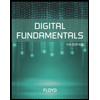
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
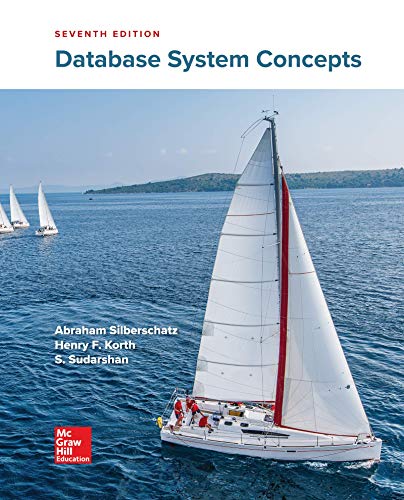
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
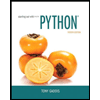
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
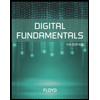
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
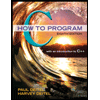
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
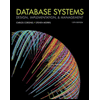
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
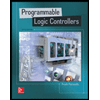
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education
Expert Answers to Latest Homework Questions
Q: Sunshine Traders uses the accrual basis of accounting. If it provides services worth $5,000 in March…
Q: I need assistance with this general accounting question using appropriate principles.
Q: Provide Answer
Q: Please explain the solution to this general accounting problem using the correct accounting…
Q: General accounting question
Q: Need help with this question solution general accounting
Q: Can you solve this general accounting problem using accurate calculation methods?
Q: Please provide the answer to this general accounting question using the right approach.
Q: I am trying to find the accurate solution to this general accounting problem with appropriate…
Q: I am looking for help with this general accounting question using proper accounting standards.
Q: Please explain the solution to this financial accounting problem with accurate explanations.
Q: I need help with this financial accounting problem using proper accounting guidelines.
Q: Can you explain the correct approach to solve this general accounting question?
Q: Please provide the accurate answer to this general accounting problem using appropriate methods.
Q: Please explain the solution to this general accounting problem with accurate explanations.
Q: Can you solve this general accounting problem with appropriate steps and explanations?
Q: Aloha Airlines Flight 007 is flying due east but finds it necessary to detour around a group of…
Q: Q7
Q: Question 6
Aloha Airlines Flight 007 is flying due east but finds it necessary to detour around a…
Q: Help fix my arrows please
Q: Given the data attached, provide a drawing of the corresponding structure.