1) Create a new Java Project named Final 2) Create a superclass VehicleRental a. Instance private data: year (int) make (String) (String) (double) model rental price b. Constructor sets instance private data fields C. Methods: • displayRental - Only displays year, make, model • Include get (use to print) and set methods for each private data field 3) Create a subclass CarRental a. This subclass contains two additional private data fields: passengers (int) (char) suv b. This subclass also contains a final and static constant for the premium amount of $50 c. The subclass constructor receives parameters for all private instance data (year, make, model, rental price) plus passengers and auv • Use the superclass constructor to set all instance data defined in the superclass (use the super keyword) • The subclass constructor also sets the additional passengers (int) private data field and the auv (char) private data field defined in the subclass. • The constructor includes logic for a $50 premium amount (constant) for an suv and applies the update to the instance data field for rental price, if applicable. Hint: retrieve the price with get method, apply premium amount to the rental price, then call the set method to update the rental price data field d. Methods: • Override the superclass di splayRental method. Call the superclass version of the method, then display additional CarRental information. Use logic to determine if "SUV upgrade" should print. Display the rental price and the number of passengers. • Include get (use to print) and set methods for each additional private data field 4) Create a subclass TruckRental a. This subclass contains two additional private data fields: capacity discount (int) (char) b. This subclass also contains a final and static constant for the discount amount of $25 . The subclass constructor receives parameters for all private instance data (year, make, model, rental price) plus capacity and discount • Use the superclass constructor to set all instance data defined in the superclass (use the super keyword) • The subclass constructor also sets the additional capacity (int) private data field and the discount (char) private data field defined in the subclass. • The constructor includes logic for a $25 discount amount (constant) and applies the update to the instance data field for rental price, ifapplicable. Hint: retrieve the price with get method, apply discount amount to the rental price, then call the set method to update the rental price data field d. Methods: • Override the superclass di aplayRental method. Call the super class version of the method, then display additional TruckRental information. Use logic to determine if "DISCOUNT applied" should print. Display the rental price and the truck capacity in pounds. • Include get (use to print) and set methods for each additional private data field 5) Create a driver program RentalDeno Create 4 appropriate objects from data below: CarRentalt 2022, "Chevrolet", "Malibu", 39.99, 5, 'N' CarRentalt 2022, "Chevrolet", "Tahoe", 79.99, 7, Y TruckRentalt 2020, "Ford", "E450", 135.99, 8900, 'Y' TruckRentalt 2006, "GMC", "C6500", 92.99, 25000, 'N' The driver program does not perform any printing or output line advancements; it invokes the appropriate di splayRental method for each object. Exnected Outnut YEAR: 2022
1) Create a new Java Project named Final 2) Create a superclass VehicleRental a. Instance private data: year (int) make (String) (String) (double) model rental price b. Constructor sets instance private data fields C. Methods: • displayRental - Only displays year, make, model • Include get (use to print) and set methods for each private data field 3) Create a subclass CarRental a. This subclass contains two additional private data fields: passengers (int) (char) suv b. This subclass also contains a final and static constant for the premium amount of $50 c. The subclass constructor receives parameters for all private instance data (year, make, model, rental price) plus passengers and auv • Use the superclass constructor to set all instance data defined in the superclass (use the super keyword) • The subclass constructor also sets the additional passengers (int) private data field and the auv (char) private data field defined in the subclass. • The constructor includes logic for a $50 premium amount (constant) for an suv and applies the update to the instance data field for rental price, if applicable. Hint: retrieve the price with get method, apply premium amount to the rental price, then call the set method to update the rental price data field d. Methods: • Override the superclass di splayRental method. Call the superclass version of the method, then display additional CarRental information. Use logic to determine if "SUV upgrade" should print. Display the rental price and the number of passengers. • Include get (use to print) and set methods for each additional private data field 4) Create a subclass TruckRental a. This subclass contains two additional private data fields: capacity discount (int) (char) b. This subclass also contains a final and static constant for the discount amount of $25 . The subclass constructor receives parameters for all private instance data (year, make, model, rental price) plus capacity and discount • Use the superclass constructor to set all instance data defined in the superclass (use the super keyword) • The subclass constructor also sets the additional capacity (int) private data field and the discount (char) private data field defined in the subclass. • The constructor includes logic for a $25 discount amount (constant) and applies the update to the instance data field for rental price, ifapplicable. Hint: retrieve the price with get method, apply discount amount to the rental price, then call the set method to update the rental price data field d. Methods: • Override the superclass di aplayRental method. Call the super class version of the method, then display additional TruckRental information. Use logic to determine if "DISCOUNT applied" should print. Display the rental price and the truck capacity in pounds. • Include get (use to print) and set methods for each additional private data field 5) Create a driver program RentalDeno Create 4 appropriate objects from data below: CarRentalt 2022, "Chevrolet", "Malibu", 39.99, 5, 'N' CarRentalt 2022, "Chevrolet", "Tahoe", 79.99, 7, Y TruckRentalt 2020, "Ford", "E450", 135.99, 8900, 'Y' TruckRentalt 2006, "GMC", "C6500", 92.99, 25000, 'N' The driver program does not perform any printing or output line advancements; it invokes the appropriate di splayRental method for each object. Exnected Outnut YEAR: 2022
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 1GZ
Related questions
Question

Transcribed Image Text:1) Create a new Java Project named Final
2) Create a superclass VehieleRental
a. Instance private data:
(int)
(String)
(String)
(double)
year
make
model
rental price
b. Constructor sets instance private data fields
c. Methods:
• displaytental - Only displays year, make, model
• Include get (use to print) and set methods for each private data field
3) Create a subclass CarRental
a. This subclass contains two additional private data fields:
(int)
(char)
passengera
auv
b. This subclass also contains a final and static constant for the premium amount of $50
c. The subclass constructor receives parameters for all private instance data (year, make, model,
rental price) plus passengera and auv
• Use the superclass constructor to set all instance data defined in the superclass (use the super
keyword)
• The subclass constructor also sets the additional passengers (int) private data field and the
auv (char) private data field defined in the subclass.
• The constructor includes logic for a $50 premium amount (constant) for an suv and applies the
update to the instance data field for rental price, if applicable. Hint: retrieve the price
with get method, apply premium amount to the rental price, then call the set method to
update the rental price data field
d. Methods:
• Override the superclass di splayRental method. Call the superclass version of the method,
then display additional CarRental information. Use logic to determine if "SUV upgrade" should
print. Display the rental price and the number of passengers.
• Include get (use to print) and set methods for each additional private data field
4) Create a subclass TruckRental
a. This subclass contains two additional private data fields
(int)
capacity
discount
(char)
b. This subclass also contains a final and static constant for the discount amount of $25
c. The subclass constructor receives parameters for all private instance data (year, make, model,
rental price) plus capacity and discount
• Use the superclass constructor to set all instance data defined in the supercass (use the super
keyword)
• The subclass constructor also sets the additional capacity (int) private data field and the
discount (char) private data field defined in the subclass.
• The constructor includes logic for a $25 discount amount (constant) and applies the update to
the instance data field for rental price, if applicable. Hint: retrieve the price with get
method, apply discount amount to the rental price, then call the set method to update the
rental price data field
d. Methods:
• Override the superclass di aplaykantal method. Call the super class version of the method,
then display additional TruckRental information. Use logic to determine if "DISCOUNT applied"
should print. Display the rental price and the truck capacity in pounds.
• Include get (use to print) and set methods for each additional private data field
5) Create a driver program RentalDemo
Create 4 appropriate objects from data below:
CarRentalt 2022, "Chevrolet", "Malibu", 39.99, 5, 'N
CarRentalt 2022, "Chevrolet", "Tahoe", 79.99, 7, Y
TruckRentalt 2020, "Ford", "E450", 135.99, 8900, 'Y'
TruckRentalt 2006, "GMC", "C6500", 92.99, 25000, 'N'
The driver program does not perform any printing or output line advancements; it invokes the
appropriate displaykental method for each object.
Expected Qutnut
YEAR: 2022
MAKE: Chevrolet
MODEL: Malibu
RENTAL PRICE: $39.99
PASSENGERS: 5
YEAR: 2022
MAKE: Chevrolet
MODEL: Tahoe
SUV upgrade
RENTAL PRICE: $129.99
PASSENGERS : 7
YEAR: 2020
MAKE: Ford
MODEL: E45e
DISCOUNT applied
RENTAL PRICE: $110.99
CAPACITY: 8,900 pounds
YEAR: 2006
MAKE: GHC
MODEL: C650e
RENTAL PRICE: $92.99
САРАСITY: 25,0ее рounds
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
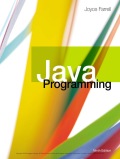
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
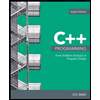
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
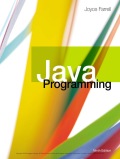
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
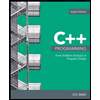
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning