JAVA Part One: The Member Class Write the class to represent a member. Include the following components: a constant to represent the current year of 2023 instance data variables: a name the year the member joined (stored as an int) whether or not the member is a VIP a constructor that sets all of the instance data variables using parameters getter and setter methods in your setter for the year, use validity checking to make sure the parameter is valid before assigning it to the instance data variable a toString method include the name and year joined in the text representation if the member is a VIP, include that in the text as well you can see my example of the formatting in the sample output below (note that I used tabs in my formatting) You should iteratively write, compile, and test each of these components while writing your class to make sure each works before moving on. Part Two: Class Method Write a method that determines whether a member is a "founding member." A founding member is defined as a member who is both a VIP and has been a member for at least ten years. Part Three: Driver Class Write a driver class with a main method that does each of the following: create six Member objects using the data below create an ArrayList of members and fill it with the six objects use a loop to print a text representation of each member count and output the number of members who have been members for less than three years count and output the number of founding members Additional Coding Requirements code should compile follow Java naming conventions for classes (upper camel case) and for variables and methods (lower camel case with no underscores) choose the best loops and conditional structures for a task use constants instead of hard-coded values when possible to improve clarity and readability and to make code easier to maintain reduce duplicated code (consider how to replace repeated code with a method) Member Data Jack Pott, joined in 2021 Chris P. Bacon, joined in 2020 Sarah Bellum, joined in 2010 as a VIP Raynor Schein, joined in 2012 as a VIP Polly Ester, joined in 2022 as a VIP Earl Lee Riser, joined in 2011 Output Here is what the output of running your driver should be: Members: Jack Pott Joined in 2021 Chris P. Bacon Joined in 2020 Sarah Bellum Joined in 2010 VIP member Raynor Schein Joined in 2012 VIP member Polly Ester Joined in 2022 VIP member Earl Lee Riser Joined in 2011 New members (members for less than three years): 2 Founding members (VIP members that have been members for at least ten years): 2
JAVA
Part One: The Member Class
Write the class to represent a member. Include the following components:
- a constant to represent the current year of 2023
- instance data variables:
- a name
- the year the member joined (stored as an int)
- whether or not the member is a VIP
- a constructor that sets all of the instance data variables using parameters
- getter and setter methods
- in your setter for the year, use validity checking to make sure the parameter is valid before assigning it to the instance data variable
- a toString method
- include the name and year joined in the text representation
- if the member is a VIP, include that in the text as well
- you can see my example of the formatting in the sample output below (note that I used tabs in my formatting)
You should iteratively write, compile, and test each of these components while writing your class to make sure each works before moving on.
Part Two: Class Method
Write a method that determines whether a member is a "founding member." A founding member is defined as a member who is both a VIP and has been a member for at least ten years.
Part Three: Driver Class
Write a driver class with a main method that does each of the following:
- create six Member objects using the data below
- create an ArrayList of members and fill it with the six objects
- use a loop to print a text representation of each member
- count and output the number of members who have been members for less than three years
- count and output the number of founding members
Additional Coding Requirements
- code should compile
- follow Java naming conventions for classes (upper camel case) and for variables and methods (lower camel case with no underscores)
- choose the best loops and conditional structures for a task
- use constants instead of hard-coded values when possible to improve clarity and readability and to make code easier to maintain
- reduce duplicated code (consider how to replace repeated code with a method)
Member Data
- Jack Pott, joined in 2021
- Chris P. Bacon, joined in 2020
- Sarah Bellum, joined in 2010 as a VIP
- Raynor Schein, joined in 2012 as a VIP
- Polly Ester, joined in 2022 as a VIP
- Earl Lee Riser, joined in 2011
Output
Here is what the output of running your driver should be:
Members:
Jack Pott Joined in 2021
Chris P. Bacon Joined in 2020
Sarah Bellum Joined in 2010 VIP member
Raynor Schein Joined in 2012 VIP member
Polly Ester Joined in 2022 VIP member
Earl Lee Riser Joined in 2011
New members (members for less than three years): 2
Founding members (VIP members that have been members for at least ten years): 2

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

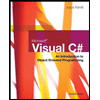
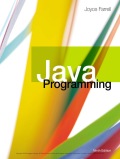
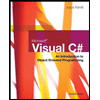
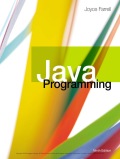