... #include #include #include /* * Function to check whether two passed strings are anagram or not */ int isAnagram(char *firstString, char *secondString) { int firstCharCounter[256] = {0}, secondCharCounter[256] = {0}; int counter; // Two Strings cannot be anagram if their length is not equal if(strlen(firstString) != strlen(secondString)) return 0; // count frequency of characters of firstString for(counter = 0; firstString[counter] != '\0'; counter++) { firstCharCounter[firstString[counter]]++; } // count frequency of characters of secondString for(counter = 0; secondString[counter] != '\0'; counter++) { secondCharCounter[secondString[counter]]++; } // compare character counts of both strings, // If not equal return 0, otherwise 1 for (counter = 0; counter < 256; counter++) { if (firstCharCounter[counter] != secondCharCounter[counter]) return 0; } return 1; } int main() { char firstString[100], secondArray[100]; printf("First string? "); gets(firstString); printf("Second string? "); gets(secondArray); if(isAnagram(firstString, secondArray) == 1) printf("%s and %s are Anagrams\n",firstString,secondArray); else printf("%s and %s are not Anagrams\n",firstString,secondArray); getch(); return 0; }
... #include #include #include /* * Function to check whether two passed strings are anagram or not */ int isAnagram(char *firstString, char *secondString) { int firstCharCounter[256] = {0}, secondCharCounter[256] = {0}; int counter; // Two Strings cannot be anagram if their length is not equal if(strlen(firstString) != strlen(secondString)) return 0; // count frequency of characters of firstString for(counter = 0; firstString[counter] != '\0'; counter++) { firstCharCounter[firstString[counter]]++; } // count frequency of characters of secondString for(counter = 0; secondString[counter] != '\0'; counter++) { secondCharCounter[secondString[counter]]++; } // compare character counts of both strings, // If not equal return 0, otherwise 1 for (counter = 0; counter < 256; counter++) { if (firstCharCounter[counter] != secondCharCounter[counter]) return 0; } return 1; } int main() { char firstString[100], secondArray[100]; printf("First string? "); gets(firstString); printf("Second string? "); gets(secondArray); if(isAnagram(firstString, secondArray) == 1) printf("%s and %s are Anagrams\n",firstString,secondArray); else printf("%s and %s are not Anagrams\n",firstString,secondArray); getch(); return 0; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
PLease help me to add a loop that will let program repeat until the user will type 'exit' C language
...
#include <stdio.h>
#include <conio.h>
#include <string.h>
/*
* Function to check whether two passed strings are anagram or not
*/
int isAnagram(char *firstString, char *secondString)
{
int firstCharCounter[256] = {0}, secondCharCounter[256] = {0};
int counter;
// Two Strings cannot be anagram if their length is not equal
if(strlen(firstString) != strlen(secondString))
return 0;
// count frequency of characters of firstString
for(counter = 0; firstString[counter] != '\0'; counter++)
{
firstCharCounter[firstString[counter]]++;
}
// count frequency of characters of secondString
for(counter = 0; secondString[counter] != '\0'; counter++)
{
secondCharCounter[secondString[counter]]++;
}
// compare character counts of both strings,
// If not equal return 0, otherwise 1
for (counter = 0; counter < 256; counter++)
{
if (firstCharCounter[counter] != secondCharCounter[counter])
return 0;
}
return 1;
}
int main()
{
char firstString[100], secondArray[100];
printf("First string? ");
gets(firstString);
printf("Second string? ");
gets(secondArray);
if(isAnagram(firstString, secondArray) == 1)
printf("%s and %s are Anagrams\n",firstString,secondArray);
else
printf("%s and %s are not Anagrams\n",firstString,secondArray);
getch();
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
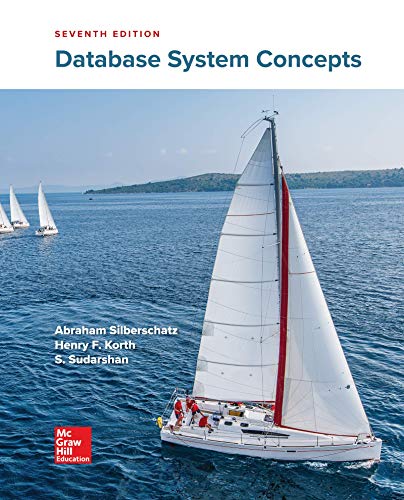
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
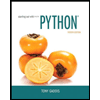
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
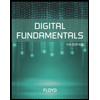
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
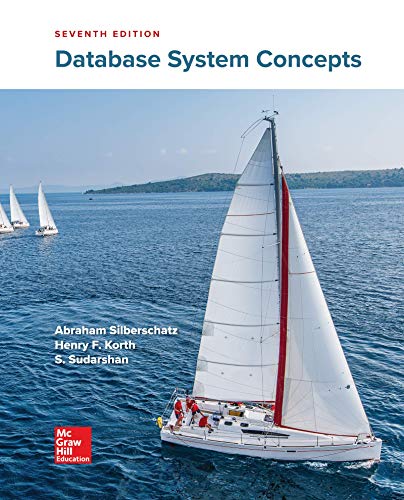
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
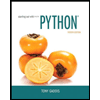
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
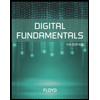
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
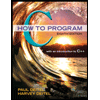
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
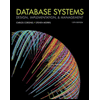
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
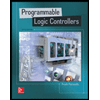
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education