Week2_Design_ender
.pdf
keyboard_arrow_up
School
University of Toronto *
*We aren’t endorsed by this school
Course
106
Subject
Mechanical Engineering
Date
Apr 3, 2024
Type
Pages
14
Uploaded by AmbassadorFang201
Week2_Design_ender
January 21, 2022
0.1
APS106 Lecture Notes - Week 2, Lecture 3
1
An Engineering Design Process for Programming
As you have seen in APS111/112, a key part of engineering is the design of objects, processes, and
systems.
From an engineering perspective, programming is the design, implementation, testing,
and documentation of a piece of software that solves a particular problem.
The software might
be part of a larger system (e.g., the avionics software of an aircraft, the accounting or human
resources software of a business), but it represents the solution to a design problem (or part of a
design problem).
We will therefore approach programing as an engineering design process and adapt the process you
have already seen.
1
1.1
An Engineering Design Process (for programming)
1.1.1
1. Define the Problem
Develop a clear and detailed problem statement. Be clear on what needs to be done. Sometimes the
problem will be easy enough (especially as you are learning programming) that the initial problem
statement given by the client/prof is suffcient. More often, the problem is complex enough that
forming a complete, explicit definition is a challenge itself and sometimes (even, often) the client
doesn’t really understand the problem him/herself. In such cases, research and iteration with the
client is necessary.
1.1.2
2. Define Test Cases
Work out specific test cases for which you know the answer.
This will help in the solidifying
the problem definition and provide you with tests once you have working code.
Try to cover a
reasonable span of possible cases that may come up. Think about strange cases that might break
the code. Think about reasonable measures of effciency, speed, and memory size.
1.1.3
3. Generate Many Creative Solutions
Think about solutions and write them down. Try to be as creative as possible.
A “solution” at this stage is two things:
1.
An Algorithm Plan
: a list of a few (from 4 or 5 to a dozen) steps that your algorithm will
execute to solve the problem. These are high-level steps that can correspond to many lines of
code. In real projects, these steps will themselves be subject to the design process (i.e. they
will in turn be broken down into sub-steps perhaps may layers deep).
2.
A Programming Plan
: a list of steps you will take in programming the algorithm. Some-
times this will be the form of programming, testing, and debugging each of the algorithm
steps in order.
But it doesn’t have to be that way.
Especially for larger systems, the al-
gorithm steps may be designed and implemented by different people in parallel or you may
choose to program, test, and debug the hardest step first to make sure you understand the
problem enough. Or you may decide to do the easiest steps first.
The point is that you program not by trying to write all the code at once and then hoping it all
works. Rather, you divide it up into a number of steps and make sure each step is implemented
and works as you proceed.
1.1.4
4. Select a Solution
Evaluate the algorithm and programming plans you have generated. Does it appear that this solu-
tion will truly solve the problem? You may write some prototype code to understand if particular
design ideas will work. Pick the best solution. If it is good enough, continue to Step 5, otherwise
return to an earlier step (maybe even Step 1 as you have uncovered new parts of the problem
definition).
1.1.5
5. Implement the Solution
Follow your chosen programming plan to implement the code. For each step in your programming
plan, you should ensure that the code is working: it runs some “sub-tests” correctly. Even though
2
it doesn’t solve the whole problem, it should produce intermediate results that you can verify are
correct. If it doesn’t, you should debug it before moving onto the next step. Implementation in-
cludes the documentation in the code: functions should have well-written docstrings and comments
should be used – it is better to over-comment than under-comment.
1.1.6
6. Perform Final Testing
Evaluate the solution against the test metrics, ensuring everything is in order. If the solution is not
satisfactory, you need to either return to Step 5 to debug the code or return to Step 1 to develop
a better understanding of the problem.
1.2
Final Remark: Design is Iterative
The above seems very proper and linear.
Real programming isn’t.
Real programming is a but
chaotic because you are creating something that doesn’t yet exist and figuring out how to solve the
problem as you go. Having some structure will help you not get lost.
One of the most essential parts of all engineering design processes is iteration. Programming is no
different. In fact, iteration may be even more important in programming because it is relatively
inexpensive to write prototype code (compared to, say, building a prototype engine). This means
that steps in the process are repeated over and over, in a loop. You might realize that you need
to jump back to an earlier step because you missed a key requirement or because you mistakenly
thought that you understood how to program a particular step. Each iteration brings with it an
increased level of understanding of the problem that deepens your knowledge. Iteration may allow
you to conceive solutions that were not initially apparent.
2
Design Project # 1: Forward Kinematics
2.1
Problem Background
If you have a robotic arm (e.g., the Canadarm) with joints, it is important to be able to calculate
where the end of the arm (i.e., the part usually used for picking something up) will be based on
the characteristics of the arm (e.g., the length of the components) and the angles of its joints.
Forward kinematics is the use of the kinematic equations of a robot to compute the position of the
end of the arm (end-effector) from specified values for the joint parameters. Forward kinematics is
3
used heavily in robotics, computer games, and animation.
2.2
1. Define the Problem
Given a robotic arm with two degrees of freedom (see above diagram), determine the position (x,y)
of the effector given the component-arm lengths and joint angles.
We need to find the
x
and
y
coordinates of the end of the arm. Those coordinates will obviously
depend on the location of the base of the arm. And so a relevant question to the client is if we
can define our own coordinate system or if there is a larger system that this arm is part of. Let’s
assume that we can define our own coordinate system.
Something to think about: how expensive will it be if this assumption is wrong? Will we have to
throw away all our work and start again? Or is there likely to be an easy way to take a solution
with a fixed coordinate system and reuse it in an externally specified coordinate system?
2.3
2. Define Test Cases
2.3.1
Test Case 1
len1 = 1, len2 = 1, ang1 = 60, ang2 = 30
End effector position x = 0.5, y = 1.87
2.3.2
Test Case 2
len1 = 1, len2 = 1, ang1 = 60, ang2 = -30
4
End effector position x = 1.37, y = 1.37
Where do these test cases come from? Either the client gives them to you or you have to figure out
from first principles (or research) how to calculate the answers by hand.
2.4
3. Generate Many Creative Solutions
Based on simple physics and math, we can obtain the (
∆
x
1
,
∆
y
1
) position of the end of the first
component arm.
∆
x
1
=
L
1
cos
(
θ
1
)
(1)
∆
y
1
=
L
1
sin
(
θ
1
)
(2)
Then we can obtain the (
∆
x
2
,
∆
y
2
) position for arm 2.
∆
x
2
=
L
2
cos
(
θ
2
+
θ
1
)
(3)
∆
y
2
=
L
2
sin
(
θ
2
+
θ
1
)
(4)
Finally we can find the (x,y) position by adding up the components.
x
= ∆
x
1
+ ∆
x
2
(5)
y
= ∆
y
1
+ ∆
y
2
(6)
These steps nicely form an Algorithm Plan
1. Get arm lengths and angles from the user.
2. Calculate (x,y) position of the end of arm 1.
3. Calculate the (x,y) position of the end of arm 2.
5
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
You are a biomedical engineer working for a small orthopaedic firm that fabricates rectangular shaped fracture
fixation plates from titanium alloy (model = "Ti Fix-It") materials. A recent clinical report documents some problems with the plates
implanted into fractured limbs. Specifically, some plates have become permanently bent while patients are in rehab and doing partial
weight bearing activities.
Your boss asks you to review the technical report that was generated by the previous test engineer (whose job you now have!) and used to
verify the design. The brief report states the following... "Ti Fix-It plates were manufactured from Ti-6Al-4V (grade 5) and machined into
solid 150 mm long beams with a 4 mm thick and 15 mm wide cross section. Each Ti Fix-It plate was loaded in equilibrium in a 4-point bending
test (set-up configuration is provided in drawing below), with an applied load of 1000N. The maximum stress in this set-up was less than the
yield stress for the Ti-6Al-4V…
arrow_forward
Please do not copy other's work and do not use ChatGPT or Gpt4,i will be very very very appreciate!!!
Thanks a lot!!!!!
arrow_forward
https://ethuto.cut.ac.za/webapps x
Content
Take Test: Main assessment link - x
ethuto.cut.ac.za/webapps/assessment/take/launch.jsp?course_assessment_id= 26832 1&course_id3_10885_1&content_id%3 625774 1&step3Dnull
Question Completion Status:
QUESTION 20
A single-stage, single-acting air compressor with runs at speed 600 rpm. It has a polytropic index of
1.26. The induced volume flow rate is 7.2 cubic meters per min and the air must be delivered at a
pressure of 668 kPa. The free air conditions are 101.3 kPa and 18 degrees Celsius. The clearance
volume is 6% of the stroke volume and the stroke/bore ratio is 1.6/1. Calculate the delivery air.
Hint answer usaully in C and no need for °C.
arrow_forward
+ → CO
A student.masteryconnect.com/?iv%3D_n5SY3Pv5S17e01Piby
Gr 8 Sci Bench 1 GradeCam Rutherford TN 2021
AHMAD, ASHNA
D0
3 of 35
A student develops a model of an electric motor using two pins, a wire coil,
coil continues to spin with a certain speed.
wire coil
pins
magnet
tape
battery
How can the student increase the speed of the electric motor?
O by using wider pins
O by using thinner pins
O by using less wire in the clil
O by using more wire in the coil
e Type here to search
近
arrow_forward
MECT361
Mechatronics Components and Instrumentation
PLEASE GIVE ME THE short answer and wite it by keyword
thanks
arrow_forward
Please solve, engineering econ
arrow_forward
Identify the lines
arrow_forward
Problem 1: You are working in a consulting company that does a lot of hand calculations for designs in
Aerospace Industry for mechanical, thermal, and fluidic systems. You took the Virtual engineering
course, and you want to convince your boss and the team you work to move to modelling and simulation
in computers using a certain software (Ansys, Abaqus, etc). Discuss the benefits and pitfalls of computer
based models used within an industrial environment to solve problems in engineering.
arrow_forward
Motiyo
Add explanation
arrow_forward
The following tools / resources may be useful for you to complete the assignment:a. Chatgpt (You may use it to learn Matlab coding or any other computer language. An example is given here: https://shareg.pt/mXHGne9 ). Please take note that code generated by chatgpt can be directly copied and pasted.b. Matlabi) Useful cheat sheet (https://n.ethz.ch/~marcokre/download/ML-CheatSheet.pdf)ii) Getting started with Matlab (https://matlabacademy.mathworks.com/en/details/gettingstarted )iii) Getting 30-day Matlab trial license (https://www.mathworks.com/campaigns/products/trials.html ) iv) Polyfit (https://www.mathworks.com/help/matlab/ref/polyfit.html )v) Exponential Fit (https://www.mathworks.com/matlabcentral/answers/91159-how-do-i-fit-an-exponential-curve-to-my-data)c. PlotDigitizer (https://plotdigitizer.sourceforge.net/ ) or a free online app that does not requires installation (https://plotdigitizer.com/app )You may use your own engineering judgement to make any assumptions on any…
arrow_forward
I need help solving this problem.
arrow_forward
Please solve very soon
arrow_forward
PLTW Engineering
Activity 3.8 Precision and Accuracy of
Measurement
Introduction
This concept of random and systematic errors is related to the precision and accuracy
of measurements. Precision characterizes the system's probability of providing the
same result every time a sample is measured (related to random error). Accuracy
characterizes the system's ability to provide a mean close to the true value when a
sample is measured many times (related to systematic error). We can determine the
precision of a measurement instrument by making repeated measurements of the same
sample and calculating the standard deviation of those measurements. However, we
will not be able to correct any single measurement due to a low precision instrument.
Simply stated, the effects of random uncertainties can be reduced by repeated
measurement, but it is not possible to correct for random errors.
We can determine the accuracy of a measurement instrument by comparing the
experimental mean of a large number…
arrow_forward
Content
WP NWP Assessment Builder UI Appl x
WP NWP Assessment Player UI Appli x
b My Questions | bartleby
bartleby premium - Twitter Searc x
+
i education.wiley.com/was/ui/v2/assessment-player/index.html?launchld=3bd9f9b2-6dae-43e7-a150-c87c490093e0#/question/0
e M1A4-ATTEMPT 1
Question 1 of 6
>
-/2
View Policies
Current Attempt in Progress
A particle which moves with curvilinear motion has coordinates in millimeters which vary with the time t in seconds according to x =
1.9t2 - 3.6t and y = 3.0t2 - t°/4.6. Determine the magnitudes of the velocity v and acceleration a and the angles which these vectors
make with thex-axis when t =7.0 s.
Answers: When t = 7.0 s,
V =
mm/s,
e, =
i
mm/s?, 0x =
i
a =
eTextbook and Media
Save for Later
Attempts: 0 of 1 used
Submit Answer
7:31 PM
O Type here to search
日
DEI
1/24/2022
...
II
arrow_forward
immediately
arrow_forward
4. Documents business requirements use-case narratives.for only one process
note: please i want Documents like this in pic
arrow_forward
I want to briefly summarize what he is talking about and what you conclude.
pls very urgent
arrow_forward
Please recheck and provide clear and complete step-by-step solution/explanation in scanned handwriting or computerized output thank you
arrow_forward
In the automotive industry, supercars are highly accredited with how they are manufacture; from the
type of car chassis used to the type of materials employed. Often enough, companies like BMW,
Mercedes & Audi produces supercars that exemplifies a better reliability compared to other automotive
manufacturing companies. This is because they pay close attention to the details on how the car is
manufactured; right from raw materials to a finished supercar. The task given to you is to watch the
video link provided below & explain the electrostatic process acquired for the two different models of
BMW vehicles.
https://www.youtube.com/watch?v=sUqKUbmdOr0
Pls watch the video before answering
arrow_forward
Scenario
You are assigned a role as a mechanical engineer for a vehicle design manufacturing company. Your
department has a software to perform numerical differentiation and integration. To be able to verify the
results of using the software and validate these results, your department manager has asked you to
analytically perform some tasks to validate the results generated by the software.
Q: is the last two digits of your student Id number. If your number is (20110092) then Q=92.
P: is the last digit of your student Id Number. If your number is (20110092) then P=2,
If that digit equals zero then use P=1. Example: If your number is (20110040) then P=1.
Task 1
Determine the gradient of following functions at the given points:
a) x(t) = (2t7 + P t-2)² + (6vi – 5) when t = 1
5s+7
b) v(s) =
when s = 3
(s²-P)2
c) i(t) = 5(1 – In(2t – 1) )
when t= 1 sec.
d) V(t) =5sin(100nt + 0.2) Volts , find i(t) = 10 × x10-6 dV©)
Ampere when t= 1ms.
dt
e) y(t) = e¬(t-n) sin(Qt + P)
when t = n radian
f)…
arrow_forward
ethuto.cut.ac.za/ultra/courses/_9063_1/cl/outline
Question Completion Status:
Moving to another question will save this response.
gestion 9
MECHANICAL AND CIVIL ENGINEERING
Two forces
and 2 are acting on a particle.
20 N
45°
30°
16N
The magnitude of the resultant force is:
O4 287+6.147
O B. 12.37 N
OC 28.67 N
D.
None of the above is correct
arrow_forward
You are an engineer in a company that manufactures and designs several mechanical devices, and your manager asked you to help your customers. In this time, you have two customers, one of them wants to ask about internal combustion engines while the other requires a heat exchanger with particular specifications. Follow the parts in the following tasks to do your job and support your customers.Task 1:Your first customer asked for an internal combustion engine to use it in a designed car. Your role is to describe the operation sequence of different types of available engines, explain their mechanical efficiency, and deliver a detailed technical report which includes the following steps:STEP 1Describe with the aid of diagrams the operational sequence of four stroke spark ignition and four stroke compression ignition engines.STEP 2Explain and compare the mechanical efficiency of two and four-stroke engines.STEP 3Review the efficiency of ideal heat engines operating on the Otto and Diesel…
arrow_forward
You are an engineer in a company that manufactures and designs several mechanical devices, and your manager asked you to help your customers. In this time, you have two customers, one of them wants to ask about internal combustion engines while the other requires a heat exchanger with particular specifications. Follow the parts in the following tasks to do your job and support your customers.Task 1:Your first customer asked for an internal combustion engine to use it in a designed car. Your role is to describe the operation sequence of different types of available engines, explain their mechanical efficiency, and deliver a detailed technical report which includes the following steps:STEP 1Describe with the aid of diagrams the operational sequence of four stroke spark ignition and four stroke compression ignition engines.STEP 2Explain and compare the mechanical efficiency of two and four-stroke engines.STEP 3Review the efficiency of ideal heat engines operating on the Otto and Diesel…
arrow_forward
Hello tutors, help me. Just answer "Let Us Try"
arrow_forward
Please do not rely too much on chatgpt, because its answer may be wrong. Please consider it carefully and give your own answer. You can borrow ideas from gpt, but please do not believe its answer.Very very grateful!Please do not rely too much on chatgpt, because its answer may be wrong. Please consider it carefully and give your own answer. You can borrow
ideas from gpt, but please do not believe its answer.Very very grateful!
arrow_forward
kamihq.com/web/viewer.html?state%=D%7B"ids"%3A%5B"1vSrSXbH_6clkKyVVKKAtzZb_GOMRwrCG"%5D%...
lasses
Gmail
Copy of mom it for..
Маps
OGOld Telephone Ima.
Preview attachmen...
Kami Uploads ►
Sylvanus Gator - Mechanical Advantage Practice Sheet.pdf
rec
Times New Roman
14px
1.5pt
BIUSA
A Xa x* 三三
To find the Mechanical Advantage of ANY simple machine when given the force, use MA = R/E.
1.
An Effort force of 30N is appliled to a screwdriver to pry the lid off of a can of paint. The
screwdriver applies 90N of force to the lid. What is the MA of the screwdriver?
MA =
arrow_forward
HW_5_01P.pdf
PDF
File | C:/Users/Esther/Downloads/HW_5_01P.pdf
2 Would you like to set Microsoft Edge as your default browser?
Set as default
To be most productive with Microsoft Edge, finish setting up your
Complete setup
Maybe later
browser.
(D Page view A Read aloud V Draw
7 Highlight
2
of 3
Erase
5. Two cables are tied to the 2.0 kg ball shown below. The ball revolves in a horizontal
circle at constant speed. (Hint: You will need to use some geometry and properties of
triangles and their angles!)
60°
1.0 m
60°
© 2013 Pearson Education, Inc.
(a) For what speed is the tension the same in both cables?
(b) What is the tension?
2.
2:04 PM
O Type here to search
C A
2/9/2021
(8)
arrow_forward
Please recheck and provide clear and complete step-by-step solution in scanned handwriting or computerized output thank you
arrow_forward
: Fundamentals of Mechatronics Technology
arrow_forward
REC
M Gmail
x4 BIT203 - Google x
Gmail: Free. Pria x
Dashboard
BIT203-Study W x
docs.google.com/document/d/1unVPvr_GhUFhrQuNdGICLqFle1ZB51_BICKagvoXoSU/edite
BIT203-Study WorkShop * D e
a Share
File Edit View insert Format Tools Add-ons Help Lastedit was made minutes ngoby Seri ECT
150 -
Nomal tet
Times Ne
12 • BIU
Discuss the tools and technologies for collaboration and social business that are available and
how they provide value to an organization.
I
arrow_forward
HELLO, CAN U HELP ME TO MY RESEARCH PART?, MY RESEARCH PART IS DATA GATHERING PROCEDURE, AND I'LL SEND THE SAMPLE THAT MY TEACHER GAVE ME, THIS IS MY TITLE BTW " Students who play Axie Infinity as scholars do not make enough money to support their studies: a qualitative study of Axie Infinity scholars among the students of Precious High Academy" THANKS!
arrow_forward
Berserk - Chapter 2- Read Berserk
compressor.
reggienet.illinoisstate.edu
https://reggienet.illinoisstate.edu/access/content/attachment/f6b18576-acf9-...
Hint. Both power-sizing and indexing will be used.
Ć
+
Question 3. The purchase price of a natural gas-fired commercial boiler (capacity X) was $181,000 eight years
ago. Another boiler of the same basic design, except with the capacity 1.42X, is currently being considered for
purchase. If it is purchased, some optional features presently costing $28,000 would be added for your
application. If the cost index was 162 for this type of equipment when the capacity X boiler was purchased and
is 221 now, and the applicable cost capacity factor is 0.8, what is your estimate of the purchase price for the
new boiler?
Hint: Use both indexing and power-sizing methods.
88
Illinois State University: TEC 330 001 FA2022 - Applied Economic Analysis For...
arrow_forward
Don't Use Chat GPT Will Upvote And Give Handwritten Solution Please
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
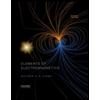
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
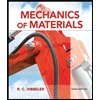
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
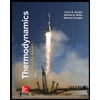
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
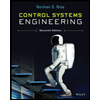
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
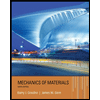
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
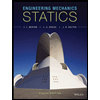
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY
Related Questions
- You are a biomedical engineer working for a small orthopaedic firm that fabricates rectangular shaped fracture fixation plates from titanium alloy (model = "Ti Fix-It") materials. A recent clinical report documents some problems with the plates implanted into fractured limbs. Specifically, some plates have become permanently bent while patients are in rehab and doing partial weight bearing activities. Your boss asks you to review the technical report that was generated by the previous test engineer (whose job you now have!) and used to verify the design. The brief report states the following... "Ti Fix-It plates were manufactured from Ti-6Al-4V (grade 5) and machined into solid 150 mm long beams with a 4 mm thick and 15 mm wide cross section. Each Ti Fix-It plate was loaded in equilibrium in a 4-point bending test (set-up configuration is provided in drawing below), with an applied load of 1000N. The maximum stress in this set-up was less than the yield stress for the Ti-6Al-4V…arrow_forwardPlease do not copy other's work and do not use ChatGPT or Gpt4,i will be very very very appreciate!!! Thanks a lot!!!!!arrow_forwardhttps://ethuto.cut.ac.za/webapps x Content Take Test: Main assessment link - x ethuto.cut.ac.za/webapps/assessment/take/launch.jsp?course_assessment_id= 26832 1&course_id3_10885_1&content_id%3 625774 1&step3Dnull Question Completion Status: QUESTION 20 A single-stage, single-acting air compressor with runs at speed 600 rpm. It has a polytropic index of 1.26. The induced volume flow rate is 7.2 cubic meters per min and the air must be delivered at a pressure of 668 kPa. The free air conditions are 101.3 kPa and 18 degrees Celsius. The clearance volume is 6% of the stroke volume and the stroke/bore ratio is 1.6/1. Calculate the delivery air. Hint answer usaully in C and no need for °C.arrow_forward
- + → CO A student.masteryconnect.com/?iv%3D_n5SY3Pv5S17e01Piby Gr 8 Sci Bench 1 GradeCam Rutherford TN 2021 AHMAD, ASHNA D0 3 of 35 A student develops a model of an electric motor using two pins, a wire coil, coil continues to spin with a certain speed. wire coil pins magnet tape battery How can the student increase the speed of the electric motor? O by using wider pins O by using thinner pins O by using less wire in the clil O by using more wire in the coil e Type here to search 近arrow_forwardMECT361 Mechatronics Components and Instrumentation PLEASE GIVE ME THE short answer and wite it by keyword thanksarrow_forwardPlease solve, engineering econarrow_forward
- Identify the linesarrow_forwardProblem 1: You are working in a consulting company that does a lot of hand calculations for designs in Aerospace Industry for mechanical, thermal, and fluidic systems. You took the Virtual engineering course, and you want to convince your boss and the team you work to move to modelling and simulation in computers using a certain software (Ansys, Abaqus, etc). Discuss the benefits and pitfalls of computer based models used within an industrial environment to solve problems in engineering.arrow_forwardMotiyo Add explanationarrow_forward
- The following tools / resources may be useful for you to complete the assignment:a. Chatgpt (You may use it to learn Matlab coding or any other computer language. An example is given here: https://shareg.pt/mXHGne9 ). Please take note that code generated by chatgpt can be directly copied and pasted.b. Matlabi) Useful cheat sheet (https://n.ethz.ch/~marcokre/download/ML-CheatSheet.pdf)ii) Getting started with Matlab (https://matlabacademy.mathworks.com/en/details/gettingstarted )iii) Getting 30-day Matlab trial license (https://www.mathworks.com/campaigns/products/trials.html ) iv) Polyfit (https://www.mathworks.com/help/matlab/ref/polyfit.html )v) Exponential Fit (https://www.mathworks.com/matlabcentral/answers/91159-how-do-i-fit-an-exponential-curve-to-my-data)c. PlotDigitizer (https://plotdigitizer.sourceforge.net/ ) or a free online app that does not requires installation (https://plotdigitizer.com/app )You may use your own engineering judgement to make any assumptions on any…arrow_forwardI need help solving this problem.arrow_forwardPlease solve very soonarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Elements Of ElectromagneticsMechanical EngineeringISBN:9780190698614Author:Sadiku, Matthew N. O.Publisher:Oxford University PressMechanics of Materials (10th Edition)Mechanical EngineeringISBN:9780134319650Author:Russell C. HibbelerPublisher:PEARSONThermodynamics: An Engineering ApproachMechanical EngineeringISBN:9781259822674Author:Yunus A. Cengel Dr., Michael A. BolesPublisher:McGraw-Hill Education
- Control Systems EngineeringMechanical EngineeringISBN:9781118170519Author:Norman S. NisePublisher:WILEYMechanics of Materials (MindTap Course List)Mechanical EngineeringISBN:9781337093347Author:Barry J. Goodno, James M. GerePublisher:Cengage LearningEngineering Mechanics: StaticsMechanical EngineeringISBN:9781118807330Author:James L. Meriam, L. G. Kraige, J. N. BoltonPublisher:WILEY
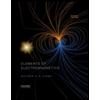
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
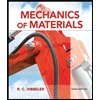
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
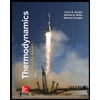
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
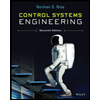
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
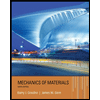
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
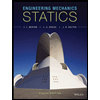
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY