Explanation of Solution
Program:
File name: “Purchase.java”
//Define a class named Purchase
public class Purchase
{
//Declare the private variables
private int invoiceNumber;
private double saleAmount;
private double tax;
private static final double RATE = 0.05;
//Define a method to set the invoice number
public void setInvoiceNumber(int num)
{
invoiceNumber = num;
}
//Define a method to set the amount of sale
public void setSaleAmount(double amt)
{
saleAmount = amt;
//Calculate the amount of sales tax
tax = saleAmount * RATE;
}
/*Define a method to display the invoice number,
amount of sale, and amount of sales tax*/
public void display()
{
//Print the result
System.out.println("Invoice #" + invoiceNumber +
" Amount of sale: $" + saleAmount + " Tax: $" + tax);
}
}
File name: “PurchaseArray.java”
//Import necessary header files
import java.util.*;
//Define a class named PurchaseArray
public class PurchaseArray
{
//Define a main method
public static void main(String[] args)
{
//Declare a purchases object
Purchase[] purchases = new Purchase[5];
//Declare the variables
int num;
double amount;
String entry;
//Create an object for Scanner class
Scanner input = new Scanner(System.in);
//Declare the variables and initialize the values
int x;
final int LOW = 1000, HIGH = 8000;
//For loop to be executed until x exceeds 5
for(x = 0; x < purchases.length; ++x)
{
purchases[x] = new Purchase();
//Prompt the user to enter an invoice number
System.out.print("Enter invoice number >> ");
num = input...

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
MindTapV2.0 for Farrell's Java Programming with 2021 Updates, 9th Edition [Instant Access], 1 term
- RSA and Diffie-Hellman are important algorithms in public-key cryptography. What are the differences between the two? Assume you intercept the ciphertext C = 105 sent to a user whose public key is e = 7, n = 403. Explain in detail how you will find the private key of the user and crack the ciphertext. What is the plaintext M?arrow_forwardCBC-Pad is a block cipher mode of operation commonly used in block ciphers. CBC-Pad handles plain text of any length. Padding is used to ensure that the plaintext input is a multiple of the block length. Hence, the ciphertext is longer than the plaintext by at most the size of a single block.Assume that the original plaintext is 556 bytes and the size of a cipher block is 28 bytes. What will be the padding? If the original plaintext is an integer multiple of the block size, will padding still be needed? Why or why not?arrow_forwardAbstract classes & Interfaces (Ch13) 5. See the code below and solve the following. class Circle { protected double radius; // Default constructor public Circle() ( } this(1.0); // Construct circle with specified radius public Circle(double radius) { } this.radius radius; // Getter method for radius public double getRadius() { } return radius; // Setter method for radius public void setRadius(double radius) { } this.radius = radius; // Implement the findArea method defined in GeometricObject public double findArea() { } return radius* radius * Math. PI; // Implement the find Perimeter method defined in GeometricObject public double findPerimeter() { } return 2*radius*Math.PI; // Override the equals() method defined in the Object class public boolean equals(Circlel circle) { } return this.radius == circle.getRadius(); // Override the toString() method defined in the Object class public String toString() { } } return "[Circle] radius = " + radius; 5-1. Define a class name…arrow_forward
- 6. What is Race condition? How to prevent it? [2 marks] 7. How many synchronization methods do you know and compare the differences. [2 marks] 8. Explain what are the “mutual exclusion”, “deadlock”, “livelock”, and “eventual entry”, with the traffic intersection as an example like dinning philosophy. [2 marks] 9. For memory allocation, what are the difference between internal fragmentation and external fragmentation. Explain with an example. [2 marks] 10. How can the virtual memory map to the physical memory. Explain with an example. [2 marks]arrow_forwardYour answers normally have 50 words. Less than 50 words will not get marks. 1. What is context switch between multiple processes? [2 marks] 2. Draw the memory layout for a C program. [2 marks] 3. How many states does a process has? [2 marks] 4. Compare the non-preemptitve scheduling and preemptive scheduling. [2 marks] 5. Given 4 process and their arrival times and next CPU burst times, what are the average times and average Turnaround time, for different scheduling algorithms including: a. First Come, First-Served (FCFS) Scheduling [2 marks] b. Shortest-Job-First (SJF) Scheduling [2 marks] c. Shortest-remaining-time-first [2 marks] d. Priority Scheduling [2 marks] e. Round Robin (RR) [2 marks] Process Arrival Time Burst Time P1 0 8 P2 1 9 P3 3 2 P4 5 4arrow_forwarda database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. [Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]arrow_forward
- a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. [Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]arrow_forwardIf a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? Order, Product, store, customer.arrow_forward
- Inheritance & Polymorphism (Ch11) There are 6 classes including Person, Student, Employee, Faculty, and Staff. 4. Problem Description: • • Design a class named Person and its two subclasses named student and Employee. • Make Faculty and Staff subclasses of Employee. • A person has a name, address, phone number, and e-mail address. • • • A person has a class status (freshman, sophomore, junior and senior). Define the status as a constant. An employee has an office, salary, and date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString() method in each class to display the class name and the person's name. 4-1. Explain on how you would code this program. (1 point) 4-2. Implement the program. (2 point) 4-3. Explain your code. (2 point)arrow_forwardSuppose you buy an electronic device that you operate continuously. The device costs you $300 and carries a one-year warranty. The warranty states that if the device fails during its first year of use, you get a new device for no cost, and this new device carries exactly the same warranty. However, if it fails after the first year of use, the warranty is of no value. You plan to use this device for the next six years. Therefore, any time the device fails outside its warranty period, you will pay $300 for another device of the same kind. (We assume the price does not increase during the six-year period.) The time until failure for a device is gamma distributed with parameters α = 2 and β = 0.5. (This implies a mean of one year.) Use @RISK to simulate the six-year period. Include as outputs (1) your total cost, (2) the number of failures during the warranty period, and (3) the number of devices you own during the six-year period. Your expected total cost to the nearest $100 is _________,…arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
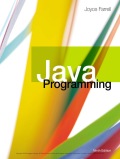
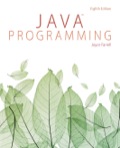
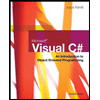
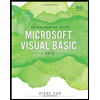
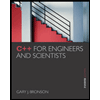