a.
Explanation of Solution
Program:
File name: “CarCareChoice.java”
//Import necessary header files
import javax.swing.*;
//Define a class named CarCareChoice
public class CarCareChoice
{
//Define a main method
public static void main (String[] args)
{
//Declare a Boolean variable and initialize the value
boolean isMatch = false;
//Declare a list of available services
String[] items = { "oil change", "tire rotation",
"battery check", "brake inspection"};
//Declare an array of values
int[] prices = {25, 22, 15, 5};
//Declare the variables
int x;
int matchIndex = 0;
//Prompt the user to enter a selection
String menu = "Enter selection:";
for(x = 0; x < items.length; ++x)
menu += "\n " + items[x];
//Show input dialog box
String selection = JOptionPane...
b.
Explanation of Solution
Program:
File name: “CarCareChoice2.java”
//Import necessary header files
import javax.swing.*;
//Define a class named CarCareChoice2
public class CarCareChoice2
{
//Define a main method
public static void main (String[] args)
{
//Declare a Boolean variable and initialize the value
boolean isMatch = false;
//Declare a list of available services
String[] items = { "oil change", "tire rotation",
"battery check", "brake inspection"};
//Declare an array of values
int[] prices = {25, 22, 15, 5};
//Declare the variables
int x;
int matchIndex = 0;
//Prompt the user to enter a selection
String menu = "Enter selection:";
for(x = 0; x < items.length; ++x)
menu += "\n " + items[x];
//Show input dialog box
String selection = JOptionPane...

Trending nowThis is a popular solution!

Chapter 8 Solutions
MindTapV2.0 for Farrell's Java Programming with 2021 Updates, 9th Edition [Instant Access], 1 term
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
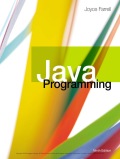
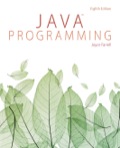
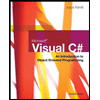
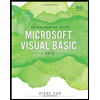