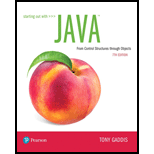
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
7th Edition
ISBN: 9780134802213
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 7.13, Problem 7.31CP
Program Plan Intro
Arraylist:
It is a collection framework and the java package “java.util.package” is used for this. Arraylist is a dynamic list which is used to store dynamic array elements.
To use Arraylist class in a Java program, it must be imported first from the Java library files.
The statement which must be imported in order to use Arraylist class is,
import java.util.Arraylist;
Syntax for Arraylist:
ArrayList() object_name=new ArrayList();
Here,
- “ArrayList()” is the class name with no arguments. The programmer can use “ArrayList(Collection c)” and “ArrayList(int)” from “util” package.
- “object_name” describes name of the object of Arraylist.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
We are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7).
A code breaker manages to factories 247 = 13 x 19
Determine Alice's secret key.
To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.
Consider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.
Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnn
Chapter 7 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Ch. 7.1 - Write statements that create the following arrays:...Ch. 7.1 - Whats wrong with the following array declarations?...Ch. 7.1 - Prob. 7.3CPCh. 7.1 - Prob. 7.4CPCh. 7.1 - Prob. 7.5CPCh. 7.1 - Prob. 7.6CPCh. 7.1 - Prob. 7.7CPCh. 7.1 - Prob. 7.8CPCh. 7.2 - Prob. 7.9CPCh. 7.2 - Prob. 7.10CP
Ch. 7.2 - A program has the following declaration: double[]...Ch. 7.2 - Look at the following statements: int[] a = { 1,...Ch. 7.3 - Prob. 7.13CPCh. 7.3 - Write a method named zero, which accepts an int...Ch. 7.6 - Prob. 7.15CPCh. 7.7 - Recall that we discussed a Rectangle class in...Ch. 7.10 - Prob. 7.17CPCh. 7.11 - What value in an array does the selection sort...Ch. 7.11 - How many times will the selection sort swap the...Ch. 7.11 - Prob. 7.20CPCh. 7.11 - Prob. 7.21CPCh. 7.11 - If a sequential search is performed on an array,...Ch. 7.13 - What import statement must you include in your...Ch. 7.13 - Write a statement that creates an ArrayList object...Ch. 7.13 - Write a statement that creates an ArrayList object...Ch. 7.13 - Prob. 7.26CPCh. 7.13 - Prob. 7.27CPCh. 7.13 - Prob. 7.28CPCh. 7.13 - Prob. 7.29CPCh. 7.13 - Prob. 7.30CPCh. 7.13 - Prob. 7.31CPCh. 7 - In an array declaration, this indicates the number...Ch. 7 - Each element of an array is accessed by a number...Ch. 7 - The first subscript in an array is always. a. 1 b....Ch. 7 - The last subscript in an array is always. a. 100...Ch. 7 - Array bounds checking happens. a. when the program...Ch. 7 - This array field holds the number of elements that...Ch. 7 - Prob. 7MCCh. 7 - This search algorithm repeatedly divides the...Ch. 7 - Prob. 9MCCh. 7 - When initializing a two-dimensional array, you...Ch. 7 - Prob. 11MCCh. 7 - To delete an item from an ArrayList object, you...Ch. 7 - To determine the number of items stored in an...Ch. 7 - True or False: java does not allow a statement to...Ch. 7 - True or False: An arrays sitze declarator can be a...Ch. 7 - Prob. 16TFCh. 7 - True or False: The subscript of the last element...Ch. 7 - Prob. 18TFCh. 7 - True or False: The Java compiler does not display...Ch. 7 - Prob. 20TFCh. 7 - True or False: The first size declarator in the...Ch. 7 - Prob. 22TFCh. 7 - Prob. 23TFCh. 7 - int[] collection = new int[-20];Ch. 7 - Prob. 2FTECh. 7 - Prob. 3FTECh. 7 - Prob. 4FTECh. 7 - Prob. 5FTECh. 7 - The variable names references an integer array...Ch. 7 - The variables numberArray1 and numberArray2...Ch. 7 - Prob. 3AWCh. 7 - In a program you need to store the populations of...Ch. 7 - In a program you need to store the identification...Ch. 7 - Prob. 6AWCh. 7 - Prob. 7AWCh. 7 - Prob. 8AWCh. 7 - Prob. 9AWCh. 7 - Prob. 10AWCh. 7 - Prob. 11AWCh. 7 - Prob. 1SACh. 7 - Prob. 2SACh. 7 - Prob. 3SACh. 7 - Prob. 4SACh. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 7SACh. 7 - Prob. 8SACh. 7 - Prob. 9SACh. 7 - Rainfall Class Write a RainFall class that stores...Ch. 7 - Payroll Class Write a Payroll class that uses the...Ch. 7 - Charge Account Validation Create a class with a...Ch. 7 - Charge Account Modification Modify the charge...Ch. 7 - Prob. 5PCCh. 7 - Drivers License Exam The local Drivers License...Ch. 7 - Magic 8 Ball Write a program that simulates a...Ch. 7 - Grade Book A teacher has five students who have...Ch. 7 - Grade Book Modification Modify the grade book...Ch. 7 - Average Steps Taken A Personal Fitness Tracker is...Ch. 7 - Array Operations Write a program with an array...Ch. 7 - Prob. 12PCCh. 7 - Sorted List of 1994 Gas Prices Note: This...Ch. 7 - Name Search If you have downloaded this books...Ch. 7 - Population Data If you have downloaded this books...Ch. 7 - World Series Champions If you have downloaded this...Ch. 7 - 2D Array Operations Write a program that creates a...Ch. 7 - Prob. 18PCCh. 7 - Trivia Game In this programming challenge, you...Ch. 7 - Prob. 20PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
- Management Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forwardPlease original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forward
- Please original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
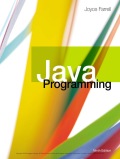
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT