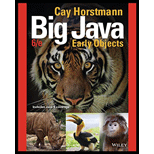
Concept explainers
a.
Explanation of Solution
Allocating an array
- In java, an array is created with the keyword new that is used for allocating memory.
- Hence allocating an array consists of two steps that is to declare a variable of the desired array type and to allocate the memory that will hold the array using new keyword and assigning it to the array variable.
- In java, all arrays are dynamically allocated.
- The code for allocating an array of ten integers is
// Declaring an array
int[] nums = new int[10];
b.
Explanation of Solution
Declaring initial element of the array
- In java, an array is created with the keyword new that is used for allocating memory.
- In java, all arrays are dynamically allocated.
- An integer variable can be used as the index of the array.
- Hence here 0 is the index of the array.
- The code for putting 17 as the initial element of the array is
// Declaring initial element
nums[0] = 17;
c.
Explanation of Solution
Declaring last element of array
- In java, an array is created with the keyword new that is used for allocating memory.
- In java, all arrays are dynamically allocated.
- An integer variable can be used as the index of the array.
- Hence here 9 is the last element of the array.
- The code for putting 29 as the last element of the array is
// Declaring last element
nums[9] = 29;
d.
Explanation of Solution
Filling remaining elements with -1
- In java, an array is created with the keyword new that is used for allocating memory.
- In java, all arrays are dynamically allocated.
- The for loop is executed for filling the remaining elements with -1.
- The code for filling the remaining elements with -1 is
// Execute for loop
for (int i = 1; i < 9; i++)
{
nums[i] = -1;
}
e.
Explanation of Solution
Adding 1 to each element of array
- In java, an array is created with the keyword new that is used for allocating memory.
- In java, all arrays are dynamically allocated.
- The for loop is executed for adding 1 to each element of array.
- The code for adding 1 to each element of array is
// Execute for loop
for (int i = 0; i < nums.length; i++)
{
nums[i]++;
}
f.
Explanation of Solution
Printing all elements of array one per line
- In java, an array is created with the keyword new that is used for allocating memory.
- In java, all arrays are dynamically allocated.
- The for loop is executed for printing all elements of array one per line.
- The code for printing all elements of array one per line is
// Execute for loop
for (int num : nums)
{
System.out.println(num);
}
g.
Explanation of Solution
Printing all elements of array in a single line
- In java, an array is created with the keyword new that is used for allocating memory.
- In java, all arrays are dynamically allocated.
- Here if condition is executed inside the for loop for printing all elements of array in a single line.
- The code for printing all elements of array in a single line is
// Execute for loop
for (int i = 0; i < nums.length; i++)
{
// Execute if condition
if (i < nums.length - 1)
// Print the statement
System.out.print(nums[i] + ",");
else
// Print the statement
System.out.println(nums[i]);
}
Want to see more full solutions like this?
Chapter 7 Solutions
Big Java, Binder Ready Version: Early Objects
- Inheritance & Polymorphism (Ch11) There are 6 classes including Person, Student, Employee, Faculty, and Staff. 4. Problem Description: • • Design a class named Person and its two subclasses named student and Employee. • Make Faculty and Staff subclasses of Employee. • A person has a name, address, phone number, and e-mail address. • • • A person has a class status (freshman, sophomore, junior and senior). Define the status as a constant. An employee has an office, salary, and date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString() method in each class to display the class name and the person's name. 4-1. Explain on how you would code this program. (1 point) 4-2. Implement the program. (2 point) 4-3. Explain your code. (2 point)arrow_forwardSuppose you buy an electronic device that you operate continuously. The device costs you $300 and carries a one-year warranty. The warranty states that if the device fails during its first year of use, you get a new device for no cost, and this new device carries exactly the same warranty. However, if it fails after the first year of use, the warranty is of no value. You plan to use this device for the next six years. Therefore, any time the device fails outside its warranty period, you will pay $300 for another device of the same kind. (We assume the price does not increase during the six-year period.) The time until failure for a device is gamma distributed with parameters α = 2 and β = 0.5. (This implies a mean of one year.) Use @RISK to simulate the six-year period. Include as outputs (1) your total cost, (2) the number of failures during the warranty period, and (3) the number of devices you own during the six-year period. Your expected total cost to the nearest $100 is _________,…arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forward
- Please answer the JAVA OOP Programming Assignment scenario below: Patriot Ships is a new cruise line company which has a fleet of 10 cruise ships, each with a capacity of 300 passengers. To manage its operations efficiently, the company is looking for a program that can help track its fleet, manage bookings, and calculate revenue for each cruise. Each cruise is tracked by a Cruise Identifier (must be 5 characters long), cruise route (e.g. Miami to Nassau), and ticket price. The program should also track how many tickets have been sold for each cruise. Create an object-oriented solution with a menu that allows a user to select one of the following options: 1. Create Cruise – This option allows a user to create a new cruise by entering all necessary details (Cruise ID, route, ticket price). If the maximum number of cruises has already been created, display an error message. 2. Search Cruise – This option allows to search a cruise by the user provided cruise ID. 3. Remove Cruise – This op…arrow_forwardI need to know about the use and configuration of files and folders, and their attributes in Windows Server 2019.arrow_forwardSouthern Airline has 15 daily flights from Miami to New York. Each flight requires two pilots. Flights that do not have two pilots are canceled (passengers are transferred to other airlines). The average profit per flight is $6000. Because pilots get sick from time to time, the airline is considering a policy of keeping four *reserve pilots on standby to replace sick pilots. Such pilots would introduce an additional cost of $1800 per reserve pilot (whether they fly or not). The pilots on each flight are distinct and the likelihood of any pilot getting sick is independent of the likelihood of any other pilot getting sick. Southern believes that the probability of any given pilot getting sick is 0.15. A) Run a simulation of this situation with at least 1000 iterations and report the following for the present policy (no reserve pilots) and the proposed policy (four reserve pilots): The average daily utilization of the aircraft (percentage of total flights that fly) The…arrow_forward
- Why is JAVA OOP is really difficult to study?arrow_forwardMy daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5. Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?arrow_forwardQ4 For the network of Fig. 1.41: a- Determine re b- Find Aymid =VolVi =Vo/Vi c- Calculate Zi. d- Find Ay smid e-Determine fL, JLC, and fLE f-Determine the low cutoff frequency. g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e). h-Sketch the low-frequency response for the amplifier using the results of part (f). Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz. 14V 15.6ΚΩ 68kQ 0.47µF Vo 0.82 ΚΩ V₁ B-120 3.3kQ 0.47µF 10kQ 1.2k0 =20µF Z₁ Fig. 1.41 Circuit forarrow_forward
- a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 1 1 0 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report.arrow_forwardUsing XML, design a simple user interface for a fictional app. Your UI should include at least three different UI components (e.g., TextView, Button, EditText). Explain the purpose of each component in your design-you need to add screenshots of your work with your name as part of the code to appear on the interface-. Screenshot is needed.arrow_forwardQ4) A thin ring of radius 5 cm is placed on plane z = 1 cm so that its center is at (0,0,1 cm). If the ring carries 50 mA along a^, find H at (0,0,a).arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
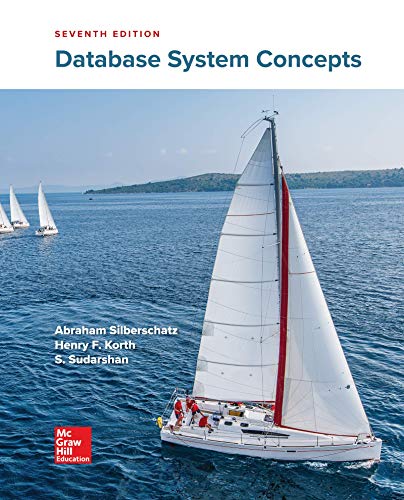
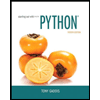
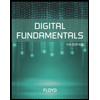
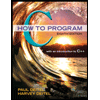
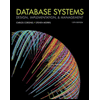
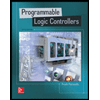