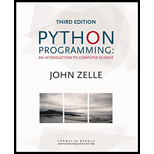
Concept explainers
Calculate day number corresponding to a date
Program plan:
- Define the function “isLeapYear()”,
- Check whether the year is not divisible by "4",
- If it is true, return “False”.
- Otherwise, check whether the year is divisible by "100",
- If it is true, check whether the year is divisible by "400",
- If it is true, return “True”.
- Otherwise, return “False”.
- Otherwise, return “True”.
- If it is true, check whether the year is divisible by "400",
- Check whether the year is not divisible by "4",
- Define the function “verifyDate()”,
- Check whether either a month is greater than "12" or day is greater than "31",
- If it is true, return “False”.
- Otherwise, Check whether day is less than or equal to "28",
- If the condition is “True”, return “True”.
- Otherwise, check whether month is "2" and day is "29",
- If it is “True” check whether the return value from “isLeapYear()” is “False”,
- If “True”, return “False”.
- Otherwise, return “True”.
- Otherwise, check whether day is equal to “31”,
- If “True”, Check whether month is either "2" or "4" or "6" or "11",
- If “True” return “False”.
- Otherwise, return “True”.
- Otherwise, return “True”.
- If “True”, Check whether month is either "2" or "4" or "6" or "11",
- Define the function “main()”,
- Get the date from the user.
- Assign month, day, and year by splitting the date by "/" using “split()” method
- Typecast month, day, and year to “int” type.
- Check whether the value return from “verifyDate()” is “False”,
- If it is “True”, print invalid.
- Otherwise, calculate the number of day using the formula.
- Check whether the month is “2”,
- If “True”, check the value return from “isLeapYear()” method is “True”,
- If “True”, calculate the day number using the formula.
- Otherwise, calculate the day number using another formula.
- Otherwise, calculate the day number using the given formula.
- Print the numeric value for the date.
- Call the function “main()”.

This Python program accepts a date as month/ day /year format, and verifies that it is a valid date, and then calculates the corresponding day number.
Explanation of Solution
Program:
File name: “Number.py”
#Define the function isLeapYear()
def isLeapYear(y):
#Check whether the year is not divisible by "4"
if (y % 4) != 0:
#Return false
return False
#Otherwise
else:
#Check whether the year is divisible by "100"
if (y % 100) == 0:
#Check whether the year is divisible by "400"
if (y % 400) ==0:
#Return true
return True
#Otherwise
else:
#Return false
return False
#Otherwise
else:
#Return true
return True
#Define the function verifyDate()
def verifyDate(mon, d, yr):
'''Check whether either a month is greater than "12" or day is gretaer than "31"'''
if mon > 12 or d > 31:
#Return "False"
return False
#Otherwise
else:
#Check whether day is less than or equal to "28"
if d <= 28:
#Return True
return True
#Check whether month is "2" and day is "29"
elif mon == 2 and d == 29:
#Check whether the return value is false
if isLeapYear(yr) == False:
#Return "False"
return False
#Otherwise
else:
#Return "True"
return True
#Check whether day is "31"
elif d == 31:
#Check whether month is either "2" or "4" or "6" or "11"
if mon == 2 or 4 or 6 or 11:
#Return "False"
return False
#Otherwise
else:
#Return True
return True
#Otherwise
else:
#Return True
return True
#Define the function main()
def main():
#Get the date from the user
date=eval(input("Enter date"))
#Initialize the value
dayNum=0
'''Assign month, day, and year by splitting the date by "/" using split() method'''
month_Str, day_Str, year_Str = date.split("/")
#Typecast month to int type
mon = int(month_Str)
#Typecast day to int type
d = int(day_Str)
#Typecast year to int type
yr = int(year_Str)
#Check whether the return value is false
if verifyDate(mon, d, yr) == False:
#Print invalid
print("This date is invalid.")
#Otherwise
else:
#Calculate the number of a day
dayNum = 31 * (mon - 1) + d
#Check whether month is "2"
if mon == 2:
#Check whether the return value is True
if isLeapYear(yr) == True:
#Calculate the day numbber
dayNum = dayNum - (4 * (mon) + 23)//10 + 1
#Otherwise
else:
#Calculate the day number
dayNum = dayNum - (4 * (mon) + 23)//10
#Otherwise
else:
#Calculate day number
dayNum = 31 * (mon - 1) + d
#Print the day number
print("The numeric value of this date is {}.".format(dayNum))
#Call the function main()
main()
Output:
Enter date'2/28/2001'
The numeric value of this date is 56.
Additional Output:
Enter date'05/25/1885'
The numeric value of this date is 149.
Want to see more full solutions like this?
Chapter 7 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- EX:[AE00]=fa50h number of ones =1111 1010 0101 0000 Physical address=4AE00h=4000h*10h+AE00h Mov ax,4000 Mov ds,ax; DS=4000h mov ds,4000 X Mov ax,[AE00] ; ax=[ae00]=FA50h Mov cx,10; 16 bit in decimal Mov bl,0 *: Ror ax,1 Jnc ** Inc bl **:Dec cx Jnz * ;LSB⇒CF Cf=1 ; it jump when CF=0, will not jump when CF=1 HW1: rewrite the above example use another wayarrow_forwardEX2: Write a piece of assembly code that can count the number of ones in word stored at 4AE00harrow_forwardWrite a program that simulates a Magic 8 Ball, which is a fortune-telling toy that displays a random response to a yes or no question. In the student sample programs for this book, you will find a text file named 8_ball_responses.txt. The file contains 12 responses, such as “I don’t think so”, “Yes, of course!”, “I’m not sure”, and so forth. The program should read the responses from the file into a list. It should prompt the user to ask a question, then display one of the responses, randomly selected from the list. The program should repeat until the user is ready to quit. Contents of 8_ball_responses.txt: Yes, of course! Without a doubt, yes. You can count on it. For sure! Ask me later. I'm not sure. I can't tell you right now. I'll tell you after my nap. No way! I don't think so. Without a doubt, no. The answer is clearly NO. (You can access the Computer Science Portal at www.pearsonhighered.com/gaddis.)arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
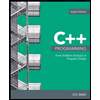
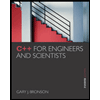
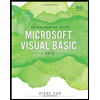
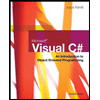
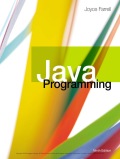