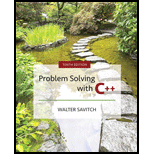
Concept explainers
Write a

Largest and Smallest numbers
Program Plan:
- Include the required headers.
- Define “main()” method.
- Declare the required “int” and “char” variables.
- Prompt the user for input file.
- Read the required input file.
- Create the object for “ifstream”.
- Open the required file.
- Check the file can be opened or not using “if” condition.
- If the file includes any error, print file “cannot be opened”.
- Initialize the input and check for largest and smallest numbers present in the file.
- Print the output using “cout”
- Return the required variable.
- Close the “main()” method.
The below C++ program describes about the displaying of largest and smallest values among the numbers present in a given file.
Explanation of Solution
Program:
//Include the needed headers
#include <iostream>
#include <fstream>
#include <cstdlib>
#include <climits>
using namespace std;
//main() Method
int main()
{
//Declaration of int variable sum
int sum = 0;
//Declaration of char variable file_name
char file_name [31];
//Declaration of input and index
int input, i = 0;
//Declaration of largest
int largest = -INT_MAX;
//Declaration of smallest
int smallest = INT_MAX;
//prompt the user for input file name
cout << "Enter a file name."
<< " This Program limits file names to"
<< endl
<< " a maximum of 30 characters. " << endl;
//read the input file name
cin >> file_name;
//creating object for ifstream
ifstream infile;
//a handle for opening the input file
infile.open(file_name);
//check the condition
if(!infile)
{
//display the error
cout << "Cannot open file " << file_name
<< " Aborting program " << endl;
//stop and exit
exit (1);
}
//initialize the input
infile >> input;
//start the loop
while(infile)
{
//check the condition
if(input > largest)
//get the largest value
largest = input;
//check the file
if(input < smallest)
//get the smallest value
smallest = input;
cout << input << " " << endl;
infile >> input;
}
//display the output
cout << "smallest in file = " << smallest
<< " largest in file = " << largest << endl;
getchar();
getchar();
//return the required value
return 0;
}
Output:
Want to see more full solutions like this?
Chapter 6 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Concepts Of Programming Languages
SURVEY OF OPERATING SYSTEMS
Degarmo's Materials And Processes In Manufacturing
- I have a few questions I need help with Statement: When we build a nearest neighbor model, we shall not remove the redundant dummies when coding a categorical variable. True or False Statement: One reason why a neural network model often requires a significant number of data observations to train is that it often has a significant number of model parameters to estimate even if there are only a few predictors. True or False. Which of the following statements about confusion matrix is wrong A) Confusion matrix is a performance measure for probability prediction techniques B) Confusion matrix is derived based on classification rules with cut-off value 0.5 C) Confusion matrix is derived based on training partition to measure a model’s predictive performance D) None of the abovearrow_forwardStudent ID is 24241357arrow_forwardWhich of the following methods help when a model suffers from high variance? a. Increase training data. b. Increase model size. c. Decrease the amount of regularization. d. Perform feature selection.arrow_forward
- 57 Formula 1 point Use shift folding, length 3, on the following value to calculate the Hash Value. 114184121 Type your answer...arrow_forwardWrite a program that reads a list of 10 integers, and outputs those integers in reverse. For coding simplicity, follow each output integer by a space, including the last one. Then, output a newline. Ex: If the input is: 2 4 6 8 10 12 14 16 18 20 the output is: 20 18 16 14 12 10 8 642 To achieve the above result, first read the integers into an array. Then output the array in reverse. 623802 1031906 nx3zmv7.arrow_forward6.3B-2. Multiple Access protocols (2). Consider the figure below, which shows the arrival of 6 messages for transmission at different multiple access nodes at times t=0.1, 0.8, 1.35, 2.6, 3.9, 4.2. Each transmission requires exactly one time unit. 1 2 3 4 t=0.0 t=1.0 t=2.0 t=3.0 5 6 t=4.0 t=5.0 For the slotted ALOHA protocol, indicate which packets are successfully transmitted. You can assume that if a packet experiences a collision, a node will not attempt a retransmission of that packet until sometime after t=5. 1 2 3 4 5 Karrow_forward
- Problem of checking Compile errors Runtime errors ======== } ng; } You have the following IQueue interface. Implement a Queue class derived from IQueue. You can use STL containers discussed in class, such as vector, queue, stack, deque, map. #include using namespace std; class IQueue { public: }; virtual void Enqueue(int val) virtual int Dequeue() = 0; virtual int Size() const = 0; int main() = 0; { Queue q; ===== } cout << q.Size() << endl; q. Enqueue(10); q.Enqueue(20); q. Enqueue(30); cout << q.Size() << endl; cout << q.Dequeue() << endl; cout << q.Size() << endl; cout << q.Dequeue() << endl; cout << q.Size() << endl; cout << q.Dequeue() << endl; cout << q.Size() << endl; ==== ====arrow_forwardlogicarrow_forwardQ1: For the Figure Below if the input to the first tank is step with magnitude 2 find 1. What type of relation between (tanks 1 and 2) and tank 3 2. Initial real value of H2, if the steady state value is 10 3. Final Value of H3 4. H1 at t=1.5 5. For the system tank1 and tank 2 only which case is applied to them (overdamping, underdamped or critically damping) A₁=1 A₂=1 Tank 1 R₁ = 2 * Tank 2 R₁₂=2 A3=0.5 hy R₁=4 Tank 3arrow_forward
- Please original work Talk about the most common challenges encountered in a data warehouse What are some creative ways to overcome those challenges What is for one real world example where your method would be effective Please cite in text references and add weblinksarrow_forwardWhat is the differences between mobile website navigation and traditional website navigation?arrow_forwardPoint 10:26 Explain P 10:26 10:25arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
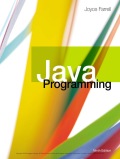
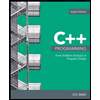