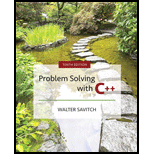
Concept explainers
Write a
(n1 – a)2, (n2 – a)2, (n3 – a)2 and so forth
The number a is the average of the numbers n1 n2, n3, and so forth. If this is being done as a class assignment, obtain the file name from your instructor. (Hint: Write your program so that it first reads the entire file and computes the average of all the numbers, and then doses the file, then reopens the file and computes the standard deviation.)

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Computer Science: An Overview (12th Edition)
Java: An Introduction to Problem Solving and Programming (7th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Database Concepts (7th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Starting Out with Programming Logic and Design (4th Edition)
- By use python languagearrow_forwardComputer Science C++ Create a text file "input.txt" with a certain amount of integers (you decide how many). Write a program that reads these numbers from the file, adds them, and when you have reached the end of the file, calculates the average of these numbers. Print a message and the average to the console. Code this program twice, demonstrating the two methods to detect the end of the file, part A: reading a value from Instream and storing it (boolean expression) in the while loop part B: using the eof() member functionarrow_forwardPython languagearrow_forward
- Write a Python program that calculates the average 5 quiz scores from a file with student ID and scores. Your program must have 2 functions; the main and calculateAvg- The main function reads data from an input file, which has the format shown below. Each line in the file starts with the Student ID followed by 5 values representing the scores attained by the Student in 5 quizzes. For each Student, the calculateAvg function receives a list containing the 5 values and returns another list containing all the values in the original list plus the average score of the quizzes for each student. This information will be written in an output file as shown below. Also, you must include the class average at the end. Which includes all the students in the list. Note: You program must be general and works for any number of Students. All reading and writing must be done in the main function. Assume each student has 5 quiz scores Input text file name must be grades.txt Output text file name must be…arrow_forwardWrite a c++ program that reads a file consisting of students’ test scores in the range 0 – 200. It should then determine the number of students having scores in each of the following ranges: 0 –24, 25 – 49, 50 – 74, 75 – 99, 100 – 124, 125 – 149, 150 – 174, and 175 – 200. Output the score ranges and the number of students. Run your program with the following input data from file: 76, 89, 150, 135, 200, 76, 12, 100, 150, 28, 178, 189, 167, 200, 175, 150, 87, 99, 129, 149, 176, 200, 87, 157, 189.arrow_forwardWrite a C++ program that reads a file consisting of students’ test scores in the range 0 – 200. It should then determine the number of students having scores in each of the following ranges: 0 – 24, 25 – 49, 50 – 74, 75 – 99, 100 – 124, 125 – 149, 150 – 174, and 175 – 200. Output the score ranges and the number of students. Run your program with the following input data from file: 76, 89, 150, 135, 200, 76, 12, 100, 150, 28, 178, 189, 167, 200, 175, 150, 87, 99, 129, 149, 176, 200, 87, 157, 189. The program should have the following functions: • void readData – to read all input values from file. This function has three parameters: the input file variable, an array, and the size. void print – to determine and display the score ranges and the number of students. int main – to call both readData and print functions. Marks are allocated as follows: main function and prototypes void readData void printData indentationarrow_forward
- Write a C++ program that prompts the user to enter a file name that contains the scores of students in a quiz. Each line in the input file contains a student name followed by the student’s score (as shown in the sample file to the right). The program should then find out the students with the minimum score and the maximum score and display their names and scores. Marks.txt Ahmed 90 Badria 85 Noor 91 Khalid 70 Said 65 Suha 80arrow_forwardDesign a python program with commentsarrow_forwardCreate a function that will take a list and returns a new list with the duplicates removed. It also returns the number of duplicates removed. The user may choose to get the data in order or sorted by sending a boolean order. Sample input [1 1 7 7 7 7 2 2 2 2 2 1 4 5 6 7 8 9 2 3 1 1 3 4 2 5 6 7 8 8] Sample output if the same order was needed [1 7 2 4 5 6 8 9 3]. 21 duplicates removed. Sample output if ordering was requested [1 2 3 4 5 6 7 8 9]. 21 duplicates removedarrow_forward
- Hello, can someone help me with this assignment? I am trying to do it on Python language: Develop a program that first reads in the name of an input file, followed by two strings representing the lower and upper bounds of a search range. The file should be read using the file.readlines() method. The input file contains a list of alphabetical, ten-letter strings, each on a separate line. Your program should output all strings from the list that are within that range (inclusive of the bounds). Ex: If the input is: input1.txt ammoniated millennium and the contents of input1.txt are: aspiration classified federation…arrow_forwardI need this code to be in pythonarrow_forwardProgram in JAVA using While Loop Write a program that takes a positive integer input and prints the numbers starting from input until 0 in one line where each numbers are separated by a space. Note: There's an initial code prepared for you. Go directly to the code editor file and complete the solution. Input 1. A positive integer input Output Enter a number: 5 5 4 3 2 1 0arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
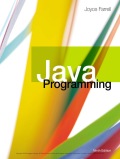