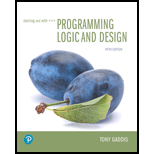
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
5th Edition
ISBN: 9780134801155
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 6, Problem 10PE
Prime Numbers
A prime number is a number that is only evenly divisible by itself and 1. For example, the number 5 is prime because it can only be evenly divided by 1 and 5. The number 6, however, is not prime because it can be divided evenly by 1, 2, 3, and 6.
Design a Boolean function named isPrime, which takes an integer as an argument and returns True if the argument is a prime number, or False otherwise. Use the function in a
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I need help in server client project. It is around 1200 lines of code in both . I want to meet with the expert online because it is complicated. I want the server send a menu to the client and the client enters his choice and keep on this until the client chooses to exit . the problem is not in the connection itself as far as I know.I tried while loops but did not work. please help its emergent
I need help in my server client in C language
Exercise
docID document text
docID document text
1
hot chocolate cocoa beans
7
sweet sugar
2345
9
cocoa ghana africa
8
sugar cane brazil
beans harvest ghana
9
sweet sugar beet
cocoa butter
butter truffles
sweet chocolate
10
sweet cake icing
11
cake black forest
Clustering by k-means, with preprocessing tokenization, term weighting TFIDF.
Manhattan Distance. Number of cluster is 2. Centroid docID 2 and docID 9.
Chapter 6 Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Ch. 6.1 - How does a function differ from a module?Ch. 6.1 - Prob. 6.2CPCh. 6.1 - Prob. 6.3CPCh. 6.1 - In pseudocode, what does the following statement...Ch. 6.1 - In pseudocode, what does the following statement...Ch. 6.2 - What is the purpose of the Return statement in a...Ch. 6.2 - Look at the following pseudocode function...Ch. 6.2 - What is a Boolean function?Ch. 6 - This is a prewritten function that is built into a...Ch. 6 - This term describes any mechanism that accepts...
Ch. 6 - This part of a function definition specifies the...Ch. 6 - This part of a function definition is comprised of...Ch. 6 - In pseudocode, this statement causes a function to...Ch. 6 - This is a design tool that describes the input,...Ch. 6 - This type of function returns either True or...Ch. 6 - This is an example of a data type conversion...Ch. 6 - Prob. 9MCCh. 6 - Prob. 10MCCh. 6 - The code for a library function must appear in a...Ch. 6 - Prob. 2TFCh. 6 - In many languages it is an error to assign a real...Ch. 6 - In some languages you must use a library function...Ch. 6 - Prob. 5TFCh. 6 - What is the difference between a module and a...Ch. 6 - Prob. 2SACh. 6 - Prob. 3SACh. 6 - Prob. 4SACh. 6 - Prob. 5SACh. 6 - Prob. 6SACh. 6 - As shown in this chapter, write a pseudocode...Ch. 6 - The following pseudocode statement calls a...Ch. 6 - A pseudocode program contains the following...Ch. 6 - Design a pseudocode function named timesTen that...Ch. 6 - Design a pseudocode function named getFirstName...Ch. 6 - Assume that a program has two String variables...Ch. 6 - The programmer intends for this pseudocode to...Ch. 6 - Can you find the reason that the following...Ch. 6 - Can you find the reason that the following...Ch. 6 - Feet to Inches One foot equals 12 inches. Design a...Ch. 6 - Math Quiz Design a program that gives simple math...Ch. 6 - Maximum of Two Values Design a function named max...Ch. 6 - Falling Distance When an object is falling because...Ch. 6 - Kinetic Energy In physics, an object that is in...Ch. 6 - Test Average and Grade Write a program that asks...Ch. 6 - Odd/Even Counter In this chapter you saw an...Ch. 6 - Guess the Number Design a number guessing game...Ch. 6 - Prime Numbers A prime number is a number that is...Ch. 6 - Prime Number List This exercise assumes you have...Ch. 6 - Rock, Paper, Scissors Game Design a program that...Ch. 6 - Prob. 13PECh. 6 - ESP Game Design a program that tests your ESP, or...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Change the following code so that there is always at least one way to get from the left corner to the top right, but the labyrinth is still randomized. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. Take care that the player and the dragon cannot start off on walls. Also the dragon starts off from a randomly chosen position public class Labyrinth { private final int size; private final Cell[][] grid; public Labyrinth(int size) { this.size = size; this.grid = new Cell[size][size]; generateLabyrinth(); } private void generateLabyrinth() { Random rand = new Random(); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { // Randomly create walls and paths grid[i][j] = new Cell(rand.nextBoolean()); } } // Ensure start and end are…arrow_forwardChange the following code so that it checks the following 3 conditions: 1. there is no space between each cells (imgs) 2. even if it is resized, the components wouldn't disappear 3. The GameGUI JPanel takes all the JFrame space, so that there shouldn't be extra space appearing in the frame other than the game. Main(): Labyrinth labyrinth = new Labyrinth(10); Player player = new Player(9, 0); Dragon dragon = new Dragon(9, 9); JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon); frame.add(gui); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; //labyrinth, player, dragon are just public classes private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");…arrow_forwardMake the following game user friendly with GUI, with some simple graphics. The GUI should be in another seperate class, with some ImageIcon, and Game class should be added into the pane. The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return…arrow_forward
- Discuss the negative and positive impacts or information technology in the context of your society. Provide two references along with with your answerarrow_forwardA cylinder of diameter 10 cm rotates concentrically inside another hollow cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poisearrow_forwardMake the following game user friendly with GUI, with some simple graphics The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…arrow_forward
- Please original work What are four of the goals of information lifecycle management think they are most important to data warehousing, Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each. Please cite in text references and add weblinksarrow_forwardThe following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forwardIn this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forward
- How do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forwardYou are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forwardDistributed Systems: Consistency Models fer to page 45 for problems on data consistency. structions: Compare different consistency models (e.g., strong, eventual, causal) for distributed databases. Evaluate the trade-offs between availability and consistency in a given use case. Propose the most appropriate model for the scenario and explain your reasoning. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440AZF/view?usp=sharing]arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
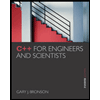
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
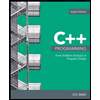
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
.2: Function Parameters and Arguments - p5.js Tutorial; Author: The Coding Train;https://www.youtube.com/watch?v=zkc417YapfE;License: Standard Youtube License