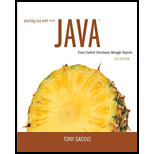
What will the following
public class Checkpoint
{
public static void main(String[ ] args)
{
int num1 = 99;
double num2 = 1.5;
System.out.println(num1 + “ ” + num2); myMethod(num1, num2);
System.out.println(num1 + “ ” + num2);
}
public static void myMethod(int i, double d)
{
System.out.println(i + “ ” + d);
i = 0;
d = 0.0;
System.out.println(i + “ ” + d);
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Starting Out with Java: From Control Structures through Objects (6th Edition)
Additional Engineering Textbook Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with C++: Early Objects (9th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Starting Out with C++ from Control Structures to Objects (8th Edition)
Problem Solving with C++ (9th Edition)
Database Concepts (7th Edition)
- public class Test { } public static void main(String[] args) { String str = "Salom"; System.out.println(str.indexOf('t)); }arrow_forwardclass main { public static void main(String args[]) { int x =2; int y =0; for(; y <10;++y) { if(y % x ==0) continue; elseif(y ==8) break; else System.out.print(y +" "); } } } Give result for the code Java Try to do ASAParrow_forwardFind error and correct it : public class ProgramLayout { public static void main (String[] args) { final double PI = 3.14159; double x; double sum = x+PI ; System.out.println (sum); } // end classarrow_forward
- class operators { public static void main(String args[]) { int x = 8; System.out.println(++x * 3 + " " + x); } } Give result for the code Java Try to do ASAP ?arrow_forwardclass Variables { public static void main(String[] args) { .arrow_forwardpublic class Turn{public static void main(String[] args){// Different initial values will be used for testingint x = 1; int y = 1; // Turn the arrow by 90 degrees System.out.print("x:", y + 90);System.out.println(x);System.out.print("y: ", x - 1 );System.out.println(y); // Turn the arrow again System.out.print("x:", y - 1);System.out.println(x);System.out.print("y: ",x + 90);System.out.println(y);}}arrow_forward
- class isinfinite_output { public static void main(String args[]) { Double d = new Double(1 / 0.); boolean x = d.isInfinite(); System.out.print(x); } } What will the given code prints on the output screen.arrow_forwardpublic class Geometry{public static void main(String[] args){//asks for user's choice Scanner in = new Scanner(System.in);displayMenu();selectOption(choice);System.out.println("Enter your choice (1-3): ");int choice1 = in.nextInt();System.out.println("Thanks for using the Geometry Calculator - Goodbye!");//Prints if input is a number that is not one of the choiceswhile(choice1 < 1 || choice1 > 3 ){System.out.println("Invalid choice. Please enter 1 - 3: ");choice1 = in.nextInt();}}/**Displays the menu*/public static void displayMenu(){System.out.println("Welcome to the Geometry Calculator Application");System.out.println("1. Calculate the Area of a Circle");System.out.println("2. Calculate the Area of a Rectangle");System.out.println("3. Calculate the Area of a Triangle");}/** This method calculates the area of the circle@return the area of the circle*/public static double circle()// calculates the area of the circle{Scanner in = new Scanner(System.in);System.out.println("What is…arrow_forwardclass Output{public static void main(String args[]){Integer i = new Integer(557);float x = i.floatValue();System.out.print(x);}} What will the given code prints on the output screen.arrow_forward
- public class Test { } public static void main(String[] args) { int a = 5; a += 5; } switch(a) { case 5: } System.out.print("5"); break; case 10: System.out.print("10"); System.out.print("0"); default:arrow_forwardFix all errors to make the code compile and complete. //MainValidatorA3 public class MainA3 { public static void main(String[] args) { System.out.println("Welcome to the Validation Tester application"); // Int Test System.out.println("Int Test"); ValidatorNumeric intValidator = new ValidatorNumeric("Enter an integer between -100 and 100: ", -100, 100); int num = intValidator.getIntWithinRange(); System.out.println("You entered: " + num + "\n"); // Double Test System.out.println("Double Test"); ValidatorNumeric doubleValidator = new ValidatorNumeric("Enter a double value: "); double dbl = doubleValidator.getDoubleWithinRange(); System.out.println("You entered: " + dbl + "\n"); // Required String Test System.out.println("Required String Test:"); ValidatorString stringValidator = new ValidatorString("Enter a required string: "); String requiredString =…arrow_forwardFix all errors to make the code compile and complete.//MainValidatorA3 public class MainA3 { public static void main(String[] args) { System.out.println("Welcome to the Validation Tester application"); // Int Test System.out.println("Int Test"); ValidatorNumeric intValidator = new ValidatorNumeric("Enter an integer between -100 and 100: ", -100, 100); int num = intValidator.getIntWithinRange(); System.out.println("You entered: " + num + "\n"); // Double Test System.out.println("Double Test"); ValidatorNumeric doubleValidator = new ValidatorNumeric("Enter a double value: "); double dbl = doubleValidator.getDoubleWithinRange(); System.out.println("You entered: " + dbl + "\n"); // Required String Test System.out.println("Required String Test:"); ValidatorString stringValidator = new ValidatorString("Enter a required string: "); String requiredString =…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
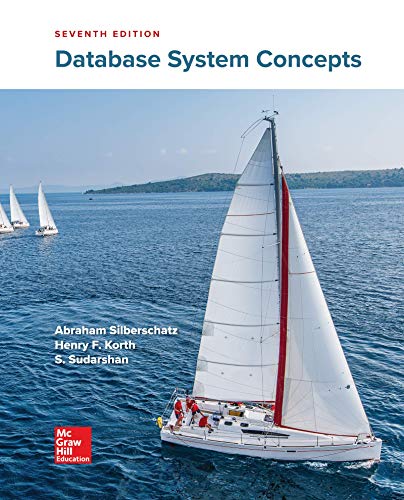
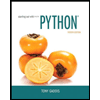
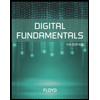
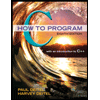
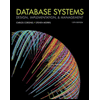
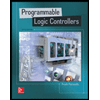