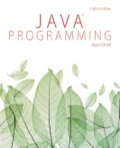
EBK JAVA PROGRAMMING
8th Edition
ISBN: 9781305480537
Author: FARRELL
Publisher: CENGAGE LEARNING - CONSIGNMENT
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 5, Problem 8PE
Program Plan Intro
Automobile application
Program plan:
- In a file “Automobile.java”, create a class “Automobile”,
- Declare the necessary variables.
- Define the constructor,
- Call the method “set_Id()”,
- Call the method “set_Make()”,
- Call the method “set_Model()”,
- Call the method “set_Color()”,
- Call the method “set_Year()”,
- Call the method “set_Mpg()”,
- Define the method “set_Id()”,
- Initialize the final variable.
- Check whether either the id is less than “0” or greater than maximum id,
- If it is true, set the id to “0”.
- Otherwise, set the initial id.
- If it is true, set the id to “0”.
- Define the method “set_Make()” to set the initial make value.
- Define the method “set_Model()” to set the initial model value.
- Define the method “set_Color()” to set the initial color value.
- Define the method “set_Year(),
- Initialize the minimum year and maximum year.
- Check whether either the year is less than the minimum year or greater than the maximum year,
- If it is true, set the year to “0”.
- Otherwise, set the initial year.
- If it is true, set the year to “0”.
- Define the method “set_Mpg()”,
- Initialize the minimum and maximum miles per gallon.
- Check whether either the Miles Per Gallon(MPG) is less than the minimum mpg or greater than the maximum mpg,
- If it is true, set the MPG to “0”.
- Otherwise, set the mpg value.
- If it is true, set the MPG to “0”.
- Define the method “get_Id()” to return the id.
- Define the method “get_Make()” to return the Make.
- Define the method “get_Model()” to return the Model.
- Define the method “get_Color()” to return the Color.
- Define the method “get_Year()” to return the year.
- Define the method “get_Mpg()” to return the MPG.
- In a file “TestAutomobiles.java”, create a class “TestAutomobiles”,
- Define the method “main ()”,
- Call the constructor “Automobile()” that instantiates several job automobile dealers.
- Call the method “display1()” for first automobile dealer.
- Call the method “display1()” for second automobile dealer.
- Call the method “set_Id()” for first automobile dealer.
- Call the method “display1()” for first automobile dealer.
- Call the method “set_Id()” for first automobile dealer.
- Call the method “display1()” for first automobile dealer.
- Call the method “set_Make()” for first automobile dealer.
- Call the method “set_Model()” for first automobile dealer.
- Call the method “display1()” for first automobile dealer.
- Call the method “set_Id()” for second automobile dealer.
- Call the method “set_Color()” for second automobile dealer.
- Call the method “set_Year()” for second automobile dealer.
- Call the method “display1()” for second automobile dealer.
- Call the method “set_Mpg()” for second automobile dealer.
- Call the method “display1()” for second automobile dealer.
- Call the method “set_Mpg()” for second automobile dealer.
- Call the method “display1()” for second automobile dealer.
- Define the method “display1()”,
- Print the output.
- Define the method “main ()”,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Lab 07: Java Graphics
(Bonus lab)
In this lab, we'll be practicing what we learned about GUIs, and Mouse events. You will need to
implement the following:
➤ A GUI with a drawing panel. We can click in this panel, and you will capture those clicks as a
Point (see java.awt.Point) in a PointCollection class (you need to build this).
о The points need to be represented by circles.
Below the drawing panel, you will need 5 buttons:
о
An input button to register your mouse to the drawing panel.
○
о
о
A show button to paint the points in your collection on the drawing panel.
A button to shift all the points to the left by 50 pixels.
The x position of the points is not allowed to go below zero.
Another button to shift all the points to the right 50 pixels.
The x position of the points cannot go further than the
You can implement this GUI in any way you choose. I suggest using the BorderLayout for a panel
containing the buttons, and a GridLayout to hold the drawing panel and button panels.…
If a UDP datagram is sent from host A, port P to host B, port Q, but at host B there is no process listening to port Q, then B is to send back an ICMP Port Unreachable message to A. Like all ICMP messages, this is addressed to A as a whole, not to port P on A.
(a) Give an example of when an application might want to receive such ICMP messages.
(b) Find out what an application has to do, on the operating system of your choice, to receive such messages.
(c) Why might it not be a good idea to send such messages directly back to the originating port P on A?
Discuss how business intelligence and data visualization work together to help decision-makers and data users. Provide 2 specific use cases.
Chapter 5 Solutions
EBK JAVA PROGRAMMING
Ch. 5 - Prob. 1RQCh. 5 - Prob. 2RQCh. 5 - Prob. 3RQCh. 5 - Prob. 4RQCh. 5 - Prob. 5RQCh. 5 - Prob. 6RQCh. 5 - Prob. 7RQCh. 5 - Prob. 8RQCh. 5 - Prob. 9RQCh. 5 - Prob. 10RQ
Ch. 5 - Prob. 11RQCh. 5 - Prob. 12RQCh. 5 - Prob. 13RQCh. 5 - Prob. 14RQCh. 5 - Prob. 16RQCh. 5 - Prob. 17RQCh. 5 - Prob. 18RQCh. 5 - Prob. 19RQCh. 5 - Prob. 20RQCh. 5 - Prob. 1PECh. 5 - Prob. 2PECh. 5 - Prob. 4PECh. 5 - Prob. 5PECh. 5 - Prob. 7PECh. 5 - Prob. 8PECh. 5 - Prob. 9PECh. 5 - Prob. 10PECh. 5 - Prob. 1GZCh. 5 - Prob. 2GZCh. 5 - Prob. 3GZCh. 5 - Prob. 4GZCh. 5 - Prob. 5GZ
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- This week we will be building a regression model conceptually for our discussion assignment. Consider your current workplace (or previous/future workplace if not currently working) and answer the following set of questions. Expand where needed to help others understand your thinking: What is the most important factor (variable) that needs to be predicted accurately at work? Why? Justify its selection as your dependent variable.arrow_forwardAccording to best practices, you should always make a copy of a config file and add a date to filename before editing? Explain why this should be done and what could be the pitfalls of not doing it.arrow_forwardIn completing this report, you may be required to rely heavily on principles relevant, for example, the Work System, Managerial and Functional Levels, Information and International Systems, and Security. apply Problem Solving Techniques (Think Outside The Box) when completing. should reflect relevance, clarity, and organisation based on research. Your research must be demonstrated by Details from the scenario to support your analysis, Theories from your readings, Three or more scholarly references are required from books, UWIlinc, etc, in-text or narrated citations of at least four (4) references. “Implementation of an Integrated Inventory Management System at Green Fields Manufacturing” Green Fields Manufacturing is a mid-sized company specialising in eco-friendly home and garden products. In recent years, growing demand has exposed the limitations of their fragmented processes and outdated systems. Different departments manage production schedules, raw material requirements, and…arrow_forward
- 1. Create a Book record that implements the Comparable interface, comparing the Book objects by year - title: String > - author: String - year: int Book + compareTo(other Book: Book): int + toString(): String Submit your source code on Canvas (Copy your code to text box or upload.java file) > Comparable 2. Create a main method in Book record. 1) In the main method, create an array of 2 objects of Book with your choice of title, author, and year. 2) Sort the array by year 3) Print the object. Override the toString in Book to match the example output: @Javadoc Declaration Console X Properties Book [Java Application] /Users/kuan/.p2/pool/plugins/org.eclipse.justj.openjdk.hotspo [Book: year=1901, Book: year=2010]arrow_forwardQ5-The efficiency of a 200 KVA, single phase transformer is 98% when operating at full load 0.8 lagging p.f. the iron losses in the transformer is 2000 watt. Calculate the i) Full load copper losses ii) half load copper losses and efficiency at half load. Ans: 1265.306 watt, 97.186%arrow_forward2. Consider the following pseudocode for partition: function partition (A,L,R) pivotkey = A [R] t = L for i L to R-1 inclusive: if A[i] A[i] t = t + 1 end if end for A [t] A[R] return t end function Suppose we call partition (A,0,5) on A=[10,1,9,2,8,5]. Show the state of the list at the indicated instances. Initial A After i=0 ends After 1 ends After i 2 ends After i = 3 ends After i = 4 ends After final swap 10 19 285 [12 pts]arrow_forward
- .NET Interactive Solving Sudoku using Grover's Algorithm We will now solve a simple problem using Grover's algorithm, for which we do not necessarily know the solution beforehand. Our problem is a 2x2 binary sudoku, which in our case has two simple rules: •No column may contain the same value twice •No row may contain the same value twice If we assign each square in our sudoku to a variable like so: 1 V V₁ V3 V2 we want our circuit to output a solution to this sudoku. Note that, while this approach of using Grover's algorithm to solve this problem is not practical (you can probably find the solution in your head!), the purpose of this example is to demonstrate the conversion of classical decision problems into oracles for Grover's algorithm. Turning the Problem into a Circuit We want to create an oracle that will help us solve this problem, and we will start by creating a circuit that identifies a correct solution, we simply need to create a classical function on a quantum circuit that…arrow_forwardusing r languagearrow_forward8. Cash RegisterThis exercise assumes you have created the RetailItem class for Programming Exercise 5. Create a CashRegister class that can be used with the RetailItem class. The CashRegister class should be able to internally keep a list of RetailItem objects. The class should have the following methods: A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as an argument should be added to the list. A method named get_total that returns the total price of all the RetailItem objects stored in the CashRegister object’s internal list. A method named show_items that displays data about the RetailItem objects stored in the CashRegister object’s internal list. A method named clear that should clear the CashRegister object’s internal list. Demonstrate the CashRegister class in a program that allows the user to select several items for purchase. When the user is ready to check out, the…arrow_forward
- 5. RetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price. Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them: Description Units in Inventory PriceItem #1 Jacket 12 59.95Item #2 Designer Jeans 40 34.95Item #3 Shirt 20 24.95arrow_forwardFind the Error: class Information: def __init__(self, name, address, age, phone_number): self.__name = name self.__address = address self.__age = age self.__phone_number = phone_number def main(): my_info = Information('John Doe','111 My Street', \ '555-555-1281')arrow_forwardFind the Error: class Pet def __init__(self, name, animal_type, age) self.__name = name; self.__animal_type = animal_type self.__age = age def set_name(self, name) self.__name = name def set_animal_type(self, animal_type) self.__animal_type = animal_typearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
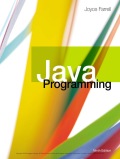
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
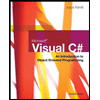
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
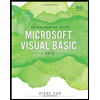
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
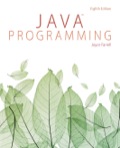
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY