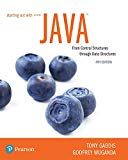
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 5, Problem 6AW
Program Plan Intro
Methods:
- The methods could be used to break a complex program into small pieces.
- The “void” method simply executes a statements group and then terminates.
- A value returning method would return a value to statement that has called it.
- While calling a method, program would branch to that method.
- It would then execute statements in its body.
- The values that are sent into a method are termed as “arguments”.
- A “parameter” denotes a special variable that holds a value that is being passed into a method.
- The “passed by value” means that only copy of a value of argument is been passed into parameter variable.
- A “local variable” is been declared inside a method, it is not accessible for statements outside method.
- A process in which a problem is been divided into smaller pieces is termed as “functional decomposition”.
Example:
Consider the example of method definition which is given below:
Public static void method()
{
Statement;
}
Here, “statement” denotes the body of method, that is to be executed when “method()” is been called.
Given code:
//Define a method display()
public static void display(int arg1 , double arg2, char arg3)
{
//Display values
System.out.println("The values are " + arg1 + " " + arg2 + ", and"+ arg3);
}
//Intialize variable
char initial = 'T';
//Intialize variable
int age = 25;
//Intialize variable
double income = 50000.00;
Explanation:
- Define a method “display()” to display the values of arguments provided to method.
- Display the values.
- Initialize values for variables “initial”, “age” and “income”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Please do not use any AI tools to solve this question.
I need a fully manual, step-by-step solution with clear explanations, as if it were done by a human tutor.
No AI-generated responses, please.
Obtain the MUX design for the function F(X,Y,Z) = (0,3,4,7) using an off-the-shelf MUX with an active low strobe input (E).
I cannot program smart home automation rules from my device using a computer or phone, and I would like to know how to properly connect devices such as switches and sensors together ?
Cisco Packet Tracer
1. Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.
Chapter 5 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 5.1 - Prob. 5.1CPCh. 5.1 - Prob. 5.2CPCh. 5.1 - Prob. 5.3CPCh. 5.1 - What message will the following program display if...Ch. 5.1 - Prob. 5.5CPCh. 5.2 - What is the difference between an argument and a...Ch. 5.2 - Prob. 5.7CPCh. 5.2 - Prob. 5.8CPCh. 5.2 - Prob. 5.9CPCh. 5.2 - What will the following program display? public...
Ch. 5.4 - Prob. 5.11CPCh. 5.4 - Prob. 5.12CPCh. 5.4 - Prob. 5.13CPCh. 5.4 - Prob. 5.14CPCh. 5 - Prob. 1MCCh. 5 - Prob. 2MCCh. 5 - Prob. 3MCCh. 5 - Prob. 4MCCh. 5 - A value that is passed into a method when it is...Ch. 5 - Prob. 6MCCh. 5 - Prob. 7MCCh. 5 - Prob. 8MCCh. 5 - Prob. 9MCCh. 5 - True or False: You terminate a method header with...Ch. 5 - Prob. 11TFCh. 5 - Prob. 12TFCh. 5 - Prob. 13TFCh. 5 - Prob. 14TFCh. 5 - Prob. 15TFCh. 5 - Prob. 16TFCh. 5 - Prob. 17TFCh. 5 - True or False: No two methods in the same program...Ch. 5 - True or False: It is possible for one method to...Ch. 5 - True or False: You must have a return statement in...Ch. 5 - Prob. 1FTECh. 5 - Look at the following method header: public static...Ch. 5 - Prob. 3FTECh. 5 - Prob. 4FTECh. 5 - Prob. 1AWCh. 5 - Here is the code for the displayValue method,...Ch. 5 - Prob. 3AWCh. 5 - What will the following program display? public...Ch. 5 - A program contains the following method...Ch. 5 - Prob. 6AWCh. 5 - Prob. 7AWCh. 5 - Write a method named square that accepts an...Ch. 5 - Write a method named getName that prompts the user...Ch. 5 - Write a method named quartersToDol1ars. The method...Ch. 5 - Prob. 1SACh. 5 - Prob. 2SACh. 5 - What is the difference between an argument and a...Ch. 5 - Where do you declare a parameter variable?Ch. 5 - Prob. 5SACh. 5 - Prob. 6SACh. 5 - Prob. 1PCCh. 5 - Retail Price Calculator Write a program that asks...Ch. 5 - Rectangle AreaComplete the Program If you have...Ch. 5 - Paint Job Estimator A painting company has...Ch. 5 - Prob. 5PCCh. 5 - Celsius Temperature Table The formula for...Ch. 5 - Test Average and Grade Write a program that asks...Ch. 5 - Conversion Program Write a program that asks the...Ch. 5 - Distance TraveLed Modification The distance a...Ch. 5 - Stock Profit The profit from the sale of a stock...Ch. 5 - Multiple Stock Sales Use the method that you wrote...Ch. 5 - Kinetic Energy In physics, an object that is in...Ch. 5 - isPrime Method A prime number is a number that is...Ch. 5 - Prime Number List Use the isPrime method that you...Ch. 5 - Even/Odd Counter You can use the following logic...Ch. 5 - Present Value Suppose you want to deposit a...Ch. 5 - Rock, Paper, Scissors Game Write a program that...Ch. 5 - ESP Game Write a program that tests your ESP...
Knowledge Booster
Similar questions
- using r language for integration theta = integral 0 to infinity (x^4)*e^(-x^2)/2 dx (1) use the density function of standard normal distribution N(0,1) f(x) = 1/sqrt(2pi) * e^(-x^2)/2 -infinity <x<infinity as importance function and obtain an estimate theta 1 for theta set m=100 for the estimate whatt is the estimate theta 1? (2)use the density function of gamma (r=5 λ=1/2)distribution f(x)=λ^r/Γ(r) x^(r-1)e^(-λx) x>=0 as importance function and obtain an estimate theta 2 for theta set m=1000 fir the estimate what is the estimate theta2? (3) use simulation (repeat 1000 times) to estimate the variance of the estimates theta1 and theta 2 which one has smaller variance?arrow_forwardusing r language A continuous random variable X has density function f(x)=1/56(3x^2+4x^3+5x^4).0<=x<=2 (1) secify the density g of the random variable Y you find for the acceptance rejection method. (2) what is the value of c you choose to use for the acceptance rejection method (3) use the acceptance rejection method to generate a random sample of size 1000 from the distribution of X .graph the density histogram of the sample and compare it with the density function f(x)arrow_forwardusing r language a continuous random variable X has density function f(x)=1/4x^3e^-(pi/2)^4,x>=0 derive the probability inverse transformation F^(-1)x where F(x) is the cdf of the random variable Xarrow_forward
- using r language in an accelerated failure test, components are operated under extreme conditions so that a substantial number will fail in a rather short time. in such a test involving two types of microships 600 chips manufactured by an existing process were tested and 125 of them failed then 800 chips manufactured by a new process were tested and 130 of them failed what is the 90%confidence interval for the difference between the proportions of failure for chips manufactured by two processes? using r languagearrow_forwardI want a picture of the tools and the pictures used Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardA. What will be printed executing the code above?B. What is the simplest way to set a variable of the class Full_Date to January 26 2020?C. Are there any empty constructors in this class Full_Date?a. If there is(are) in which code line(s)?b. If there is not, how would an empty constructor be? (create the code lines for it)D. Can the command std::cout << d1.m << std::endl; be included after line 28 withoutcausing an error?a. If it can, what will be printed?b. If it cannot, how could this command be fixed?arrow_forward
- Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardTransform the TM below that accepts words over the alphabet Σ= {a, b} with an even number of a's and b's in order that the output tape head is positioned over the first letter of the input, if the word is accepted, and all letters a should be replaced by the letter x. For example, for the input aabbaa the tape and head at the end should be: [x]xbbxx z/z,R b/b,R F ① a/a,R b/b,R a/a, R a/a,R b/b.R K a/a,R L b/b,Rarrow_forwardGiven the C++ code below, create a TM that performs the same operation, i.e., given an input over the alphabet Σ= {a, b} it prints the number of letters b in binary. 1 #include 2 #include 3 4- int main() { std::cout > str; for (char c : str) { if (c == 'b') count++; 5 std::string str; 6 int count = 0; 7 char buffer [1000]; 8 9 10 11- 12 13 14 } 15 16- 17 18 19 } 20 21 22} std::string binary while (count > 0) { binary = std::to_string(count % 2) + binary; count /= 2; std::cout << binary << std::endl; return 0;arrow_forward
- Considering the CFG described below, answer the following questions. Σ = {a, b} • NT = {S} Productions: P1 S⇒aSa P2 P3 SbSb S⇒ a P4 S⇒ b A. List one sequence of productions that can accept the word abaaaba; B. Give three 5-letter words that can be accepted by this CFG; C. Create a Pushdown automaton capable of accepting the language accepted by this CFG.arrow_forwardGiven the FSA below, answer the following questions. b 1 3 a a b b с 2 A. Write a RegEx that is equivalent to this FSA as it is; B. Write a RegEx that is equivalent to this FSA removing the states and edges corresponding to the letter c. Note: To both items feel free to use any method you want, including analyzing which words are accepted by the FSA, to generate your RegEx.arrow_forward3) Finite State Automata Given the FSA below, answer the following questions. a b a b 0 1 2 b b 3 A. Give three 4-letter words that can be accepted by this FSA; B. Give three 4-letter words that cannot be accepted by this FSA; C. How could you describe the words accepted by this FSA? D. Is this FSA deterministic or non-deterministic?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
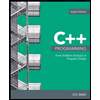
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
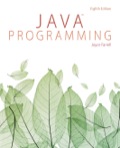
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
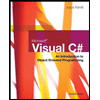
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
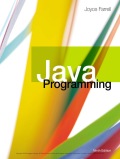
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
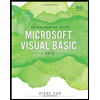
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning