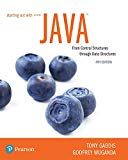
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 5, Problem 10AW
Write a method named quartersToDol1ars. The method should accept an int argument that is a number of quarters, and return the equivalent number of dollars as a double. For example, if you pass 4 as an argument, the method should return 1.0; and if you pass 7 as an argument, the method should return 1.75.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
In a C# Console App do the following
Write a method that takes three integers and returns true if their sum is divisible by 3, false otherwise.
Write a method that takes two strings and displays the string that has fewer characters.
Write a method that takes two bool variables and returns true if they have the same value, false otherwise.
Write a method that takes an int and a double and returns their product.
Write a method that returns the lcm (least common multiple) of an unspecified number of integers. The method header is specified as follows: public static int lcm(int... numbers) Write a test program that prompts the user to enter five numbers, invokes the method to find the lcm of these numbers, and displays the lcm.
Write the following two methods:
computeDiameter: This method accepts the radius (r) of a circle, and returns its diameter (2*r; radius = 7.5).
Declare all variables used here & initialize radius:
Show method call:
Write method code here:
displayData: This method accepts two arguments: radius & diameter. The output should have appropriate messages on the screen.
Show method call:
Write method code here:
Chapter 5 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 5.1 - Prob. 5.1CPCh. 5.1 - Prob. 5.2CPCh. 5.1 - Prob. 5.3CPCh. 5.1 - What message will the following program display if...Ch. 5.1 - Prob. 5.5CPCh. 5.2 - What is the difference between an argument and a...Ch. 5.2 - Prob. 5.7CPCh. 5.2 - Prob. 5.8CPCh. 5.2 - Prob. 5.9CPCh. 5.2 - What will the following program display? public...
Ch. 5.4 - Prob. 5.11CPCh. 5.4 - Prob. 5.12CPCh. 5.4 - Prob. 5.13CPCh. 5.4 - Prob. 5.14CPCh. 5 - Prob. 1MCCh. 5 - Prob. 2MCCh. 5 - Prob. 3MCCh. 5 - Prob. 4MCCh. 5 - A value that is passed into a method when it is...Ch. 5 - Prob. 6MCCh. 5 - Prob. 7MCCh. 5 - Prob. 8MCCh. 5 - Prob. 9MCCh. 5 - True or False: You terminate a method header with...Ch. 5 - Prob. 11TFCh. 5 - Prob. 12TFCh. 5 - Prob. 13TFCh. 5 - Prob. 14TFCh. 5 - Prob. 15TFCh. 5 - Prob. 16TFCh. 5 - Prob. 17TFCh. 5 - True or False: No two methods in the same program...Ch. 5 - True or False: It is possible for one method to...Ch. 5 - True or False: You must have a return statement in...Ch. 5 - Prob. 1FTECh. 5 - Look at the following method header: public static...Ch. 5 - Prob. 3FTECh. 5 - Prob. 4FTECh. 5 - Prob. 1AWCh. 5 - Here is the code for the displayValue method,...Ch. 5 - Prob. 3AWCh. 5 - What will the following program display? public...Ch. 5 - A program contains the following method...Ch. 5 - Prob. 6AWCh. 5 - Prob. 7AWCh. 5 - Write a method named square that accepts an...Ch. 5 - Write a method named getName that prompts the user...Ch. 5 - Write a method named quartersToDol1ars. The method...Ch. 5 - Prob. 1SACh. 5 - Prob. 2SACh. 5 - What is the difference between an argument and a...Ch. 5 - Where do you declare a parameter variable?Ch. 5 - Prob. 5SACh. 5 - Prob. 6SACh. 5 - Prob. 1PCCh. 5 - Retail Price Calculator Write a program that asks...Ch. 5 - Rectangle AreaComplete the Program If you have...Ch. 5 - Paint Job Estimator A painting company has...Ch. 5 - Prob. 5PCCh. 5 - Celsius Temperature Table The formula for...Ch. 5 - Test Average and Grade Write a program that asks...Ch. 5 - Conversion Program Write a program that asks the...Ch. 5 - Distance TraveLed Modification The distance a...Ch. 5 - Stock Profit The profit from the sale of a stock...Ch. 5 - Multiple Stock Sales Use the method that you wrote...Ch. 5 - Kinetic Energy In physics, an object that is in...Ch. 5 - isPrime Method A prime number is a number that is...Ch. 5 - Prime Number List Use the isPrime method that you...Ch. 5 - Even/Odd Counter You can use the following logic...Ch. 5 - Present Value Suppose you want to deposit a...Ch. 5 - Rock, Paper, Scissors Game Write a program that...Ch. 5 - ESP Game Write a program that tests your ESP...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Given the definition of a d structure as defined in this chapter, write a function called d that takes a pointe...
Programming in C
Give an example of each of the following, other than those described in this chapter, and clearly explain why y...
Modern Database Management
When displaying a Java applet, the browser invokes the _____ to interpret the bytecode into the appropriate mac...
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Assume pint is a pointer variable. For each of the following statements, determine whether the statement is val...
Starting Out with C++: Early Objects (9th Edition)
Tuition Increase At one college, the tuition for a full-time student is 6,000 per semester. It has been announc...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Change for a Dollar Game Create a change-counting game that gets the user to enter the number of coins required...
Starting out with Visual C# (4th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In this program, create a method with the following signature: public static double getAverage(double d1, double d2, double d3, double d4, double d5) This method will accept five doubles and return the average. Also in program, create a method with the following signature: public static char determineGrade(double avg) This method will take an average, then return the corresponding letter grade. Write in java and use test case# as an example.arrow_forwardThis program will ask a user to enter their favorite color. Your program will tell the user what their “spirit animal” is based on the color entered. (Or you can do something similar but different – like online quizzes that tell you what kind of potato you are.) You will create a static method called getAnimal that takes in a single String and returns a String. In this method, you will write the code that will determine which animal gets returned. You may choose the possible colors and animals (or you may choose something else ridiculous for this program) but you must have at least 4 options for the user. For example, if they enter “red”, you might return “Tiger”. Make sure to include a response for incorrect inputs. In the main method you will give the program explanation, ask for input (favorite color), capture the input, call the getAnimal method (passing in the user’s input), and print the result. No try/catch blocks or loops are necessary. The static method should contain…arrow_forwardCreate a method that takes a value and indicate whether it is positive or negative by a return aBoolean value.Declared as: boolean isPositive (float a)arrow_forward
- Write a program (use value returning method) that prompts you to enter five scores. The program should display the average score and the corresponding letter grade for the average score. Write the following “solider” methods in the program: calc_average: this method accepts five scores as arguments and returns the average determine_grade: this method accepts an average score as an argument and returns a letter based on the following: 90-100: A 80-89: B 70-79: C 60-69: D.using java programmingarrow_forwardGiven the code below, write a method call for the method (assume that each parameter is a String). Then determine that method’s output given the arguments that you pass. METHOD trickOrTreat (parameters: candy) BEGIN CREATE treat ← 26 SWITCH candy CASE “Tootsie Roll”: treat ++ CASE “Candy Corn”: treat += 2 BREAK CASE “Twizzlers”: treat -= 2 CASE “Dots”: treat -- CASE “Kit Kat”: treat *= 2 END SWITCH RETURN treat END METHOD Method Call Exact Outputarrow_forward088..arrow_forward
- The area of a pentagon can be computed usingthe following formula: Write a method that returns the area of a pentagon using the following header:public static double area(double side)Write a main method that prompts the user to enter the side of a pentagon anddisplays its area. Here is a sample run:Enter the side: 5.5 ↵EnterThe area of the pentagon is 52.04444136781625arrow_forwardQuestion 2 Write a method int countMultiplesofFive (int x, int y) that returns the number of multiples of 5 bounded between x and y inclusive. In the main, ask the user to enter two positive numbers (both must be positive, if users enter a negative value, they keep reentering values until both numbers are positive). Use the method countMultiplesofFive to find and print how many multiples of 5 exists between the two entered numbers inclusive. Sample run 1: First random: 11 Second random: 80 There are 14 umber that are multiple of five between 11 and 80 Sample run 2: First random: 75 Second random: 33 There are 9 number that are multiple of five between 33 and 75arrow_forwardWrite an application that prompts for and reads "double" value representing a monetary amount. Then determine the fewest number of each bill and coin needed to represent that amount, starting with the highest (assuming that a ten-dollar bill is the maximum size needed). For example, if the value entered is 47.63, then the program should print the equivalent amount as:4 ten dollar bills1 five dollar bills2 one dollar bills2 quarters1 dimes0 nickels3 pennies Using your Eclipse / Java integrated development environment (IDE), develop and code a solution to Programming Project 2.11 from pages 94-95 of the text book.arrow_forward
- Prime Numbers A prime number is a number that can be evenly divided by only itself and 1. For example, the number 5 is prime because it can be evenly divided by only 1 and 5. The number 6, however, is not prime because it can be evenly divided by 1, 2, 3, and 6. Write a Boolean method named IsPrime that takes an integer as an argument and returns true if the argument is a prime number or false otherwise. Use the method in an application that lets the user enter a number and then displays a message indicating whether the number is prime.arrow_forwarda) Write a boolean method called productDivisibleByThree that returns true if the product of the digits of its integer parameter is divisible by 3 and false otherwise. For example, if the value passed to the method is 23 then the product of its digits is 6 (2*3). The method should retum true since 6 is divisible by 3. b) Write a main method that calls the method productDivisibleByThree for the values between 10 and 50 inclusive 10 per line. This should output the following numbers: 10 13 16 19 20 23 26 29 30 31 Using java 32 33 34 35 36 37 38 39 40 43 46 49 50arrow_forwarda) Write a boolean method called sumDivisibleByFive that returns true if the sum of the digits of its integer parameter is divisible by 5 and false otherwise. For example, if the parameter passed to the method is 73 then the sum of its digits is 10 (7+3). The method should return true since 10 is divisible by 5. b) Write a main method that calls the method sumDivisibleByFive for the values between 10 and 100 inclusive 10 per line. This should output the following numbers: 14 19 23 28 32 37 41 46 50 55 Using java 64 69 73 78 82 87 91 96arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
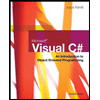
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
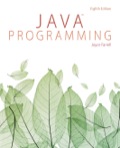
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
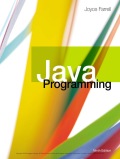
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Java Math Library; Author: Alex Lee;https://www.youtube.com/watch?v=ufegX5o8uc4;License: Standard YouTube License, CC-BY