Concept explainers
Rewrite the following code, converting the while loop to a do-while loop:
char doAgain = 'y';
int sum = 0;
cout << "This code will increment sum 1 or more times.\n";
while ((doAgain == 'y') || (doAgain == 'Y'))
{ sum++;
cout << "Sum has been incremented. Increment it again(y/n)? ";
cin >> doAgain;
}
cout << "Sum was incremented " << sum << " times.\n";

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
STARTING OUT WITH C++ MPL
Additional Engineering Textbook Solutions
Starting Out With Visual Basic (7th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Starting Out with C++ from Control Structures to Objects (8th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
- Java program | nested looparrow_forwardChallenge Problem (pyhton) T E S T S C O R E S Write a program that implements a test scores program. Valid test score entries are between 0 and 100 inclusive. The program should display a welcome message and run everything through the "main" function. have the ability to enter several test scores (try a loop) and print out the total score, as well as, the average score. continuously ask for test scores until the number 99.9 has been entered. test for valid entries and the value 99.9. If a test score is valid, the program should add the current score to the total score and update the number of test scores by one (+1), otherwise it displays an error message. Note : This assignment involves the use of a while loop and if-else decision making controls. You CANNOT use the reserved keywords break and continue for any portion of this program or any program for that matter throughout this course.arrow_forward1.Convert the while loop in the following program to for loop. #include int main() { Int num rem,reverse_num3%3; printf("nEnter any number:"); scanf("%d",&num); while(num>=1) { rem=num%10; reverse_num=reverse num*10+rem; num=num/10; printf(nReverse of input number is:%d",reverse_num); return 0:arrow_forward
- Q3: Convert the following while loop into a do-while loop. int number; int sum = 0; count<< "Enter an integer, the input ends if it is 0"; cin >> number; cout<<endl; while (number != 0) { sum += number; cout<< "Enter an integer, the input ends if it is 0"<<endl; cin >> number; }arrow_forwardQ/Rewrite the following loops as for loops. a- int m = 10; do { cout 0) { if (n == 2) break; cout << n<< " } cout << "End of Loop.";arrow_forwardPressure Unit ConversionsWrite a program that converts a value in Torr into Kilopascal. Both of these are units used to measure pressure. Use the following formula to covert Torr to Kilopascal: 1 Torr = 132/1000 KiloPascalsYour Program must use a loop to display a table of the quantities in Torr from 1 to 10 and their KiloPascal equivalents.arrow_forward
- Exercise#1: Number Sequence Using nested loops, continue and break, write a program that prompts the user to input data for five students. Each student's data contains student first name and test scores. The program should accept positive integers only for test scores and ignore non-positive integers. Each student may has a different number of test scores; the program should receive input for test scores until input "0". Finally, the program outputs each student name with the sum of his/her marks. Sample Input/Output: Enter Student name: Khaled 65 78 -40 56 -25 89 0 The student 'Khaled' has the total marks = 288 Enter Student name: Kamal 37 55 44 -77 22 18 56 26 0 The student 'Kamal' has the total marks = 258 Enter Student name: Ali 66 33 87 66 87 -25 25 39 78 0 The student 'Ali' has the total marks = 481 Enter Student name: Taher 72 67 -33 0 The student 'Taher' has the total marks = 139 Enter Student name: Ahmed 75 68 84 -18 90 0 The student 'Ahmed' has the total marks = 317…arrow_forwardCOSC 1336 –Programming Fundamentals IProgram 6 – Repetition Structures An answer should be in Python Programing Write a program that calculates the occupancy rate for a hotel. The program should start by asking the user how many floors the hotel has. A loop should then iterate once for each floor. In each iteration, the loop should ask the user for the number of rooms on the floor and how many of them are occupied. After all the iterations, the program should display how many rooms the hotel has, how many of them are occupied, howmany are unoccupied, and the percentage of rooms that are occupied. The percentage may be calculated by dividing the number of rooms occupied by the number of rooms. Run your program twice with the following data: Run 1 Floors on the hotel: 5Rooms on floor 1: 10Rooms occupied: 5Rooms on floor 2: 12Rooms occupied: 11Rooms on floor 3: 8Rooms occupied: 4Rooms on floor 4: 10Rooms occupied: 0Rooms on floor 5: 12Rooms occupied: 8 Run 2Floors on the hotel: 6Rooms…arrow_forwardConvert the following while loop to a do-while loop: int x = 1; while (x > 0) { cout << "enter a number: "; cin >> x; cout << "the number you just entered is: " << x; }arrow_forward
- Problem: Feed Nibble Monster Till Full Write a program that generates a number in [0, 500] at the beginning -- this corresponds to how hungry the monster is -- and keeps asking the user to feed the monster until that number falls to zero. Each time the user feeds the monster a nibble, hunger decreases by the decimal value of the character (i.e. if the user feeds 'A' hunger decreases by 65). But when the user feeds the monster some character that isn't a nibble, the hunger increases by the decimal value of the character (since puking depletes energy). Use while loop. Sample runs: Notice the loop exits after one iteration, because hunger was very low and one nibble made the monster full: Notice hunger increasing after non-nibble (pink highlight): Notice that the program just keeps going when the user feeds the monster only non-nibbles. Do you think the program will keep running forever if the user never gives the monster nibbles?arrow_forwardcoding language in c++ using for loops and nested for loopsarrow_forwardcoding language c++arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
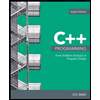