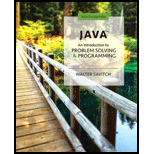
Concept explainers
Write a fragment of code that will read words from the keyboard until the word done is entered. For each word except done, report whether its first character is equal to its last character. For the required loop, use a
- a. while statement
- b. do-while statement

Explanation of Solution
a.
Using “while” statement:
The code fragment to read words until the user enters “done”. For every word, the program should check whether the first and last characters are match or not. The program using “while” condition is as follows. Code fragment is highlighted.
//Import required package
import java.util.Scanner;
//Define the Main class
public class Main
{
//Define main method
public static void main(String[] args)
{
//Create an object for scanner class
Scanner sc = new Scanner(System.in);
//Declare a variable
String word = " ";
//Do till the user enters "done"
while(!word.equals("done"))
{
//Get a word from the user
System.out.print("\nEnter a word: ");
word = sc.next();
//Check if word is equal to "done"
if(word.equals("done"))
//Break the loop
break;
//Check if 1st and last characters are equal
if(word.charAt(0) == word.charAt(word.length()-1))
//Print the message
System.out.println("The first and last character matches in '"+ word + "'");
//Else
else
//Print the message
System.out.println("The first and last character does not matches in '"+ word + "'");
}
}
}
Explanation:
- The statements under the condition “while(!word.equals("done"))” gets executed till the user enters “done”.
- The program then gets a word from the user and then it checks whether the 1st and last characters are match or not.
- The program gets halted if the user enters “done”.
Output:
Enter a word: abi
The first and last character does not matches in 'abi'
Enter a word: aruna
The first and last character matches in 'aruna'
Enter a word: done

Explanation of Solution
b.
Using “do-while” statement:
The code fragment to read words until the user enters “done”. For every word, the program should check whether the first and last character are match or not. The program using “do-while” condition is as follows. Code fragment is highlighted.
//Import required package
import java.util.Scanner;
//Define the Main class
public class Main
{
//Define main method
public static void main(String[] args)
{
//Create an object for scanner class
Scanner sc = new Scanner(System.in);
//Declare a variable
String word = " ";
//Do till the user enters "done"
do
{
//Get a word from the user
System.out.print("\nEnter a word: ");
word = sc.next();
//Check if word is equal to "done"
if(word.equals("done"))
//Break the loop
break;
//Check if 1st and last characters are equal
if(word.charAt(0) == word.charAt(word.length()-1))
//Print the message
System.out.println("The first and last character matches in '"+ word + "'");
//Else
else
//Print the message
System.out.println("The first and last character does not matches in '"+ word + "'");
} while(!word.equals("done"));
}
}
Explanation:
- The statements inside “do-while” condition gets executed till the user enters “done”.
- The program then gets a word from the user and then it checks whether the 1st and last characters are match or not.
- The program gets halted if the user enters “done”.
Output:
Enter a word: charles
The first and last character does not matches in 'charles'
Enter a word: david
The first and last character matches in 'david'
Enter a word: done
Want to see more full solutions like this?
Chapter 4 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Mechanics of Materials (10th Edition)
Starting Out With Visual Basic (8th Edition)
Modern Database Management
Degarmo's Materials And Processes In Manufacturing
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
- Ensure you answer the question asked at the end of the document. Do not just paste things without the GNS3 console outputsarrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward
- "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forwardSolve this "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward
- "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forwardSpecifications: Part-1Part-1: DescriptionIn this part of the lab you will build a single operation ALU. This ALU will implement a bitwise left rotation. Forthis lab assignment you are not allowed to use Digital's Arithmetic components.IF YOU ARE FOUND USING THEM, YOU WILL RECEIVE A ZERO FOR LAB2!The ALU you will be implementing consists of two 4-bit inputs (named inA and inB) and one 4-bit output (named out). Your ALU must rotate the bits in inA by the amount given by inB (i.e. 0-15).Part-1: User InterfaceYou are provided an interface file lab2_part1.dig; start Part-1 from this file.NOTE: You are not permitted to edit the content inside the dotted lines rectangle. Part-1: ExampleIn the figure above, the input values that we have selected to test are inA = {inA_3, inA_2, inA_1, inA_0} = {0, 1, 0,0} and inB = {inB_3, inB_2, inB_1, inB_0} = {0, 0, 1, 0}. Therefore, we must rotate the bus 0100 bitwise left by00102, or 2 in base 10, to get {0, 0, 0, 1}. Please note that a rotation left is…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
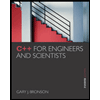
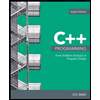
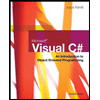
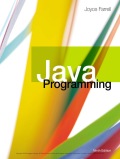
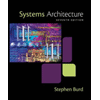