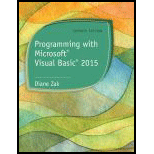
Cranston Berries
Create a Visual Basic Windows Application:
- Open Microsoft Visual Basic 2010.
- Click “File” Menu -> “New Project”.
- In the New Project pop-up window, select the “Windows Form Application” and give the name as “Cranston Project”, and specify the location as “\VB2015\Chap01\”.
Form Design:
- Design the main form and print form by placing the labels, textbox, and button and then change their name and properties.
- Inside the “Calculate” button,
- Calculate the projected sales.
- Inside the “Print” button,
- Print the form data.
- Inside the “Clear” button,
- Clear the screen.
- Inside the “Exit” button,
- Close the form.
Screenshot of the form design

Explanation of Solution
Program:
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Class frmMain
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overrides Sub Dispose(ByVal disposing As Boolean)
Try
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
Finally
MyBase.Dispose(disposing)
End Try
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.components = New System.ComponentModel.Container()
Dim resources As System.ComponentModel.ComponentResourceManager = New System.ComponentModel.ComponentResourceManager(GetType(frmMain))
Me.Label1 = New System.Windows.Forms.Label()
Me.Label2 = New System.Windows.Forms.Label()
Me.Label3 = New System.Windows.Forms.Label()
Me.Label4 = New System.Windows.Forms.Label()
Me.txtProjIncrease = New System.Windows.Forms.TextBox()
Me.txtStraw = New System.Windows.Forms.TextBox()
Me.txtBlue = New System.Windows.Forms.TextBox()
Me.txtRasp = New System.Windows.Forms.TextBox()
Me.Label5 = New System.Windows.Forms.Label()
Me.Label6 = New System.Windows.Forms.Label()
Me.lblStraw = New System.Windows.Forms.Label()
Me.lblBlue = New System.Windows.Forms.Label()
Me.lblRasp = New System.Windows.Forms.Label()
Me.btnCalc = New System.Windows.Forms.Button()
Me.btnPrint = New System.Windows.Forms.Button()
Me.btnClear = New System.Windows.Forms.Button()
Me.btnExit = New System.Windows.Forms.Button()
Me.PrintForm1 = New Microsoft.VisualBasic.PowerPacks.Printing.PrintForm(Me.components)
Me.SuspendLayout()
'
'Label1
'
Me.Label1.AutoSize = True
Me.Label1.Location = New System.Drawing.Point(27, 26)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(268, 20)
Me.Label1.TabIndex = 0
Me.Label1.Text = "Projected &increase % (in decimal form):"
'
'Label2
'
Me.Label2.AutoSize = True
Me.Label2.Location = New System.Drawing.Point(27, 108)
Me.Label2.Name = "Label2"
Me.Label2.Size = New System.Drawing.Size(94, 20)
Me.Label2.TabIndex = 2
Me.Label2.Text = "&Strawberries:"
'
'Label3
'
Me.Label3.AutoSize = True
Me.Label3.Location = New System.Drawing.Point(27, 157)
Me.Label3.Name = "Label3"
Me.Label3.Size = New System.Drawing.Size(86, 20)
Me.Label3.TabIndex = 4
Me.Label3.Text = "&Blueberries:"
'
'Label4
'
Me.Label4.AutoSize = True
Me.Label4.Location = New System.Drawing.Point(27, 203)
Me.Label4.Name = "Label4"
Me.Label4.Size = New System.Drawing.Size(89, 20)
Me.Label4.TabIndex = 6
Me.Label4.Text = "&Raspberries:"
'
'txtProjIncrease
'
Me.txtProjIncrease.Location = New System.Drawing.Point(301, 23)
Me.txtProjIncrease.Name = "txtProjIncrease"
Me.txtProjIncrease.Size = New System.Drawing.Size(57, 27)
Me.txtProjIncrease.TabIndex = 1
'
'txtStraw
'
Me.txtStraw.Location = New System.Drawing.Point(122, 105)
Me.txtStraw.Name = "txtStraw"
Me.txtStraw.Size = New System.Drawing.Size(100, 27)
Me.txtStraw.TabIndex = 3
'
'txtBlue
'
Me.txtBlue.Location = New System.Drawing.Point(122, 154)
Me.txtBlue.Name = "txtBlue"
Me.txtBlue.Size = New System.Drawing.Size(100, 27)
Me.txtBlue.TabIndex = 5
'
'txtRasp
'
Me.txtRasp.Location = New System.Drawing.Point(122, 200)
Me.txtRasp.Name = "txtRasp"
Me.txtRasp.Size = New System.Drawing.Size(100, 27)
Me.txtRasp.TabIndex = 7
'
'Label5
'
Me.Label5.AutoSize = True
Me.Label5.Location = New System.Drawing.Point(118, 78)
Me.Label5.Name = "Label5"
Me.Label5.Size = New System.Drawing.Size(95, 20)
Me.Label5.TabIndex = 12
Me.Label5.Text = "Current Sales"
'
'Label6
'
Me.Label6.AutoSize = True
Me.Label6.Location = New System.Drawing.Point(254, 78)
Me.Label6.Name = "Label6"
Me.Label6.Size = New System.Drawing.Size(110, 20)
Me.Label6.TabIndex = 13
Me.Label6.Text = "Projected Sales"
'
'lblStraw
'
Me.lblStraw.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle
Me.lblStraw.Location = New System.Drawing.Point(258, 105)
Me.lblStraw.Name = "lblStraw"
Me.lblStraw.Size = New System.Drawing.Size(100, 27)
Me.lblStraw.TabIndex = 14
Me.lblStraw.TextAlign = System.Drawing.ContentAlignment.MiddleRight
'
'lblBlue
'
Me.lblBlue.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle
Me.lblBlue.Location = New System.Drawing.Point(258, 154)
Me.lblBlue.Name = "lblBlue"
Me.lblBlue.Size = New System.Drawing.Size(100, 27)
Me.lblBlue.TabIndex = 15
Me.lblBlue.TextAlign = System.Drawing.ContentAlignment.MiddleRight
'
'lblRasp
'
Me.lblRasp.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle
Me.lblRasp.Location = New System.Drawing.Point(258, 200)
Me.lblRasp.Name = "lblRasp"
Me.lblRasp.Size = New System.Drawing.Size(100, 27)
Me.lblRasp.TabIndex = 16
Me.lblRasp.TextAlign = System.Drawing.ContentAlignment.MiddleRight
'
'btnCalc
'
Me.btnCalc.Location = New System.Drawing.Point(17, 261)
Me.btnCalc.Name = "btnCalc"
Me.btnCalc.Size = New System.Drawing.Size(81, 29)
Me.btnCalc.TabIndex = 8
Me.btnCalc.Text = "&Calculate"
Me.btnCalc.UseVisualStyleBackColor = True
'
'btnPrint
'
Me.btnPrint.Location = New System.Drawing.Point(110, 261)
Me.btnPrint.Name = "btnPrint"
Me.btnPrint.Size = New System.Drawing.Size(81, 29)
Me.btnPrint.TabIndex = 9
Me.btnPrint.Text = "&Print"
Me.btnPrint.UseVisualStyleBackColor = True
'
'btnClear
'
Me.btnClear.Location = New System.Drawing.Point(203, 261)
Me.btnClear.Name = "btnClear"
Me.btnClear.Size = New System.Drawing.Size(81, 29)
Me.btnClear.TabIndex = 10
Me.btnClear.Text = "C&lear"
Me.btnClear.UseVisualStyleBackColor = True
'
'btnExit
'
Me.btnExit.Location = New System.Drawing.Point(296, 261)
Me.btnExit.Name = "btnExit"
Me.btnExit.Size = New System.Drawing.Size(81, 29)
Me.btnExit.TabIndex = 11
Me.btnExit.Text = "E&xit"
Me.btnExit.UseVisualStyleBackColor = True
'
'PrintForm1
'
Me.PrintForm1.DocumentName = "document"
Me.PrintForm1.Form = Me
Me.PrintForm1.PrintAction = System.Drawing.Printing.PrintAction.PrintToPreview
Me.PrintForm1.PrinterSettings = CType(resources.GetObject("PrintForm1.PrinterSettings"), System.Drawing.Printing.PrinterSettings)
Me.PrintForm1.PrintFileName = Nothing
'
'frmMain
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(8!, 20!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(392, 314)
Me.Controls.Add(Me.btnExit)
Me.Controls.Add(Me.btnClear)
Me.Controls.Add(Me.btnPrint)
Me.Controls.Add(Me.btnCalc)
Me.Controls.Add(Me.lblRasp)
Me.Controls.Add(Me.lblBlue)
Me.Controls.Add(Me.lblStraw)
Me.Controls.Add(Me.Label6)
Me.Controls.Add(Me.Label5)
Me.Controls.Add(Me.txtRasp)
Me.Controls.Add(Me.txtBlue)
Me.Controls.Add(Me.txtStraw)
Me.Controls.Add(Me.txtProjIncrease)
Me.Controls.Add(Me.Label4)
Me.Controls.Add(Me.Label3)
Me.Controls.Add(Me.Label2)
Me.Controls.Add(Me.Label1)
Me.Font = New System.Drawing.Font("Segoe UI", 11.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0,Byte))
Me.Margin = New System.Windows.Forms.Padding(4, 5, 4, 5)
Me.Name = "frmMain"
Me.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen
Me.Text = "Cranston Berries"
Me.ResumeLayout(false)
Me.PerformLayout
End Sub
Friend WithEvents Label1 As Label
Friend WithEvents Label2 As Label
Friend WithEvents Label3 As Label
Friend WithEvents Label4 As Label
Friend WithEvents txtProjIncrease As TextBox
Friend WithEvents txtStraw As TextBox
Friend WithEvents txtBlue As TextBox
Friend WithEvents txtRasp As TextBox
Friend WithEvents Label5 As Label
Friend WithEvents Label6 As Label
Friend WithEvents lblStraw As Label
Friend WithEvents lblBlue As Label
Friend WithEvents lblRasp As Label
Friend WithEvents btnCalc As Button
Friend WithEvents btnPrint As Button
Friend WithEvents btnClear As Button
Friend WithEvents btnExit As Button
Friend WithEvents PrintForm1 As PowerPacks.Printing.PrintForm
End Class
- Run the program, by pressing the F5.
Screenshot of the output form
Screenshot of Calculate
Screenshot of Print Preview
Want to see more full solutions like this?
Chapter 3 Solutions
Programming with Microsoft Visual Basic 2015 (MindTap Course List)
- The purpose of this exercise is to demonstrate the importance of testing an application thoroughly. Open the FixIt Solution.sln file contained in the VB2017\Chap04\FixIt Solution folder. The application displays a shipping charge that is based on the total price entered by the user, as shown in Figure 4-64. Start the application and then test it by clicking the Display shipping button. Notice that the Shipping charge box contains $13, which is not correct. Now, test the application using the following total prices: 100, 501, 1500, 500.75, 30, 1000.33, and 2000. Here too, notice that the application does not always display the correct shipping charge. (More specifically, the shipping charge for two of the seven total prices is incorrect.) Open the Code Editor window and correct the errors in the code. Save the solution and then start and test the application.arrow_forwardFor this lab, you will be doing the following in C#: 1) Create a Home form with buttons that when clicked, will open the Account, Email, and eventually the Contact forms. The Account form should be opened in "View" mode. 2) Add code to the Login form so the Account form opens when the user clicks "Create New Account". The Account form should be opened in "Modify" mode. 3) Add code to the Login form that validates the user input before allowing the user the access the Home form. For now, just verify the user enters "user1" and "12345" for the username and password respectively.arrow_forwardHow do you trigger a PrintDocument control’s PrintPage event?arrow_forward
- Please look at image attached. This is done in Visual Basic, using Microsoft Visual Studio Community Version.arrow_forward8. Astronomy HelperCreate an application that displays the following data about the planets of the solar system (including Pluto, which is no longer considered a planet). (For your information, the distances are shown in AUs, or astronomical units. 1 AU equals approximately 93 million miles. In your application simply display the distances as they are shown here, in AUs.)MercuryType TerrestrialAverage distance from the sun 0.387 AUMass 3.31 * 1023 kgSurface temperature -173°C to 430°CVenusType TerrestrialAverage distance from the sun 0.7233 AUMass 4.87 * 1024 kgSurface temperature 472°CEarthType TerrestrialAverage distance from the sun 1 AUMass 5.967 * 1024 kgSurface temperature -50°C to 50°CMarsType TerrestrialAverage distance from the sun 1.5237 AUMass 0.6424 * 1024 kgSurface temperature -140°C to 20°CJupiterType JovianAverage distance from the sun 5.2028 AUMass 1.899 * 1027 kgTemperature at cloud tops -110°CSaturnType JovianAverage distance from the sun 9.5388 AUMass 5.69 * 1026…arrow_forwardNeed help in C# language, please.arrow_forward
- For this interactive assignment, you will create an application that grades the written portion of the driver’s license exam. The exam has 20 multiple-choice questions. Here are the correct answers: B 2. D 3. A 4. A 5. B 6. A 7. B 8. A 9. C 10. D B 12. C 13. D 14. A 15. D 16. C 17. C 18. B 19. D 20. A Your program should store these correct answers in a list. The program should read the student’s answers for each of the 20 questions from a text file and store the answers in another list. (Create your own answer text file to test the application.) After the student’s answers have been read from the text file, the program should display a message indicating whether the student passed or failed the exam. (A student must correctly answer 15 of the 20 questions to pass the exam.) It should then display the total number of correctly answered questions, the total number of incorrectly answered questions, and a list showing the…arrow_forwardDriver’s License Exam The local driver’s license office has asked you to create an application that grades the written portion of the driver’s license exam. The exam has 20 multiple-choice questions. Here are the correct answers: 1. B 2. D 3. A 4. A 5. C 6. A 7. B 8. A 9. C 10. D 11. B 12. C 13. D 14. A 15. D 16. C 17. C 18. B 19. D 20. A Your program should store these correct answers in an array. The program should read the student’s answers for each of the 20 questions from a text file and store the answers in another array. (Create your own text file to test the application.) After the student’s answers have been read from the file, the program should display a message indicating whether the student passed or failed the exam. (A student must correctly answer 15 of the 20 questions to pass the exam.) It should then display the total number of correctly answered questions, the total number of incorrectly answered questions, and a list showing the question numbers of the incorrectly…arrow_forwardAssume that variable someVariable exists in Workspace (which means it has some value assigned to it). In order to change the value assigned to someVariable to something else, you would need another variable assignment operation involving someVariable on the left side of the corresponding expression. True or false? a) True b) Falsearrow_forward
- homework Instructions: For each Exercise below, write your code in an IDE and run your code within the IDE as well. Once you have satisfied the Exercise requirements, paste your code below under the corresponding Exercise. Exercise 1: Create a Basic Calculator application using the switch statement. Your program should prompt the user to "Enter an operator (+,-, *, /): "and then take in two numbers from the user "Enter #1: "and "Enter #2: ". Use a switch to solve this. ● Don't forget to include a default case. Example (Expected) Output: ● ● Enter an operator (+, -, *, /): + Enter two numbers: 2.3 4.5 2.3+ 4.5 = 6.8arrow_forwardBrowse the web to locate the lyrics to the song “The Twelve Days of Christmas.” The song contains a list of gifts received for the holiday. The list is collective so that as each “day” passes, a new verse contains all the words of the previous verse, plus a new item. Write an application that displays the words to the song starting with any day the user enters. (Hint: Use a switch statement with cases in descending day order and without any break statements so that the lyrics for any day repeat all the lyrics for previous days.)arrow_forwardTrue or False: Adding more control variables will always increase the R 2 value. True Falsearrow_forward
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
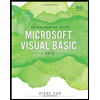
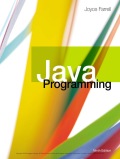