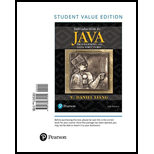
Assign grades
Program Plan:
- Import necessary packages into program.
- Define the class named “Exercise30_01”.
- Define main method.
- Define the “Scanner” object “obj” for input.
- Prompt the user and get the number of students “N” from user.
- Declare the array variable “marks[]” in type of “double”.
- Prompt and get scores using “for” loop.
- Using “DoubleStream” class assign maximum value into “best” variable.
- Declare the “grade” in type of “character”.
- Using “for” loop which is execute from “0” to “marks.length”.
- Using “if..elseif..else” condition, check the score value.
- If the value greater than “best-10”, assign “A” to “grade”.
- If the value greater than “best-20”, assign “B” to “grade”.
- If the value greater than “best-30”, assign “C” to “grade”.
- If the value greater than “best-40”, assign “D” to “grade”.
- Otherwise, assign “E” to “grade”.
- Print appropriate grade with statement on screen.
- Using “if..elseif..else” condition, check the score value.

The following JAVA code is to calculate the grade of student scores using “DoubleStream”.
Explanation of Solution
Program:
/*Include necessary packages*/
import java.util.Scanner;
//Include Stream package
import java.util.stream.DoubleStream;
//Class definition
class Exercise30_01
{
//Main method
public static void main(String[] args)
{
/*Definition of "Scanner" object*/
Scanner obj = new Scanner(System.in);
/*Prompt the user for number of students*/
System.out.print("Enter number of students: ");
//Get input from user
int N = obj.nextInt();
/*Declaration and definition of array variable*/
double[] marks = new double[N];
//Prompt the user for marks
System.out.print("Enter " + N + " scores: ");
//Loop
for (int i = 0; i < marks.length; i++)
{
/*Get scores and store into "marks[]" variable*/
marks[i] = obj.nextDouble();
}
/*Get maximum value using stream*/
double best = DoubleStream.of(marks).max().getAsDouble();
//Declaration of variable
char grade;
//Loop
for (int i = 0; i < marks.length; i++)
{
/*Condition to check marks*/
if (marks[i] >= best - 10)
//Assign grade
grade = 'A';
/*Condition to check marks*/
else if (marks[i] >= best - 20)
//Assign grade
grade = 'B';
/*Condition to check marks*/
else if (marks[i] >= best - 30)
//Assign grade
grade = 'C';
/*Condition to check marks*/
else if (marks[i] >= best - 40)
//Assign grade
grade = 'D';
//Else statement
else
//Assign grade
grade = 'F';
//Print statement
System.out.println("Student " + i + " score is " +marks[i] + " and grade is " + grade);
}
}
}
Enter number of students: 4
Enter 4 scores: 40 55 70 58
Student 0 score is 40.0 and grade is C
Student 1 score is 55.0 and grade is B
Student 2 score is 70.0 and grade is A
Student 3 score is 58.0 and grade is B
Want to see more full solutions like this?
Chapter 30 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version, Student Value Edition (11th Edition)
- Could you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forwardFor this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward
- 1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forwarda) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forward
- using r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardusing r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
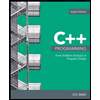
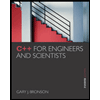
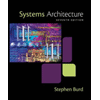