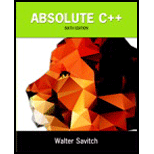
Write a function that computes the average and standard deviation of four scores. The standard deviation is defined to be the square root of the average of the four values:

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Absolute C++
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Concepts Of Programming Languages
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- Write another version of the checkeven function. This version receives 3 integer variables and returns true if all three numbers are even. Otherwise, the function returns false. Write the statements to read in three numbers and call the checkeven function. Then print YES if all three numbers were even, or print NO if they were not all even.arrow_forwardWrite a function that takes an integer. The function should return True if the integer is even and False otherwise. Use the function in a program that prompts for a number and prints "Even" or "Odd". Ex: If the input is: 15 the output is: Odd Ex: If the input is: 10 the output is: Even Your program must define and call the following function. The function should return a bool value (True or False).def is_even(num) pythonarrow_forwardLewis' invention can determine if the weather is too hot (104°F and above) or too cold (50°F and below) once the unit of measure of the temperature is in Fahrenheit. Which is why, he added another function that converts the temperature from Celsius to Fahrenheit to make his invention work. Create a program that contains the function: HotOrCold(). HotOrCold() function will accept as parameter a temperature type and the temperature and will do the following: Convert the given temperature in Celsius to Fahrenheit. a. [F = C + 32] a. Display if depending on the temperature value. Weather is too hot" or "Weather is too cold" , OF THE MADE4Learners NSTITUTE FRAMEWORK VERSITY UNI MULTIPLE APPROACHES TO DISTANCE EDUCATION FOR TECHNOLOGIAN LEARNERS : O Google Slides CHNOLOGarrow_forward
- Modify the program so that it does the following: Performs a function that gets the area of a rectangle. The function must receive two parameters (decimal numbers) that represent the base and height of the rectangle and must return the calculated value (decimal number). Perform a second function that obtains the total area of a rectangular prism with a rectangular base. The total area of such a prism is equal to the sum of the areas of each of its faces. It uses calls to the previous function for this calculation. Call this last function in the main with user data. Execution example Give me the base: 21.3 Give me the height: 10 Give me the depth: 2.0 The total area of the prism is: 551.2arrow_forwardusing phthonarrow_forwardUsing python programming a screenshot will be helpful #2arrow_forward
- Write a function in python code for checking the speed of drivers. This function should have one parameter: speed. If speed is less than 70, it should print “Ok”. Otherwise, for every 5km above the speed limit (70), it should give the driver one demerit point and print the total number of demerit points. For example, if the speed is 80, it should print: “Points: 2”. If the driver gets more than 12 points, the function should print: “License suspended”.arrow_forwardWrite a function that determines whether an exam score is valid. The function should return True if the exam score is valid, False otherwise. Use the function in a program that prompts for an exam score and prints "Valid" or "Not valid". Valid exam scores are between 0 and 100. Ex: If the input is: 82 the output is: Valid Ex: If the input is: 101 the output is: Not Valid Your program must define and call the following function. The function must return a bool value (True or False). The function should not print the result. def is_valid_score(score) pythonarrow_forwardwe will now develop a small game whose rules are as follows: • The program draws a random number between 0 and 100 inclusive and does not display it on the screen. using function : random.randint(1,100) • The player must guess the value of this number by typing his proposal on the keyboard. • The program displays as appropriate: – "Won" if the player has guessed the right number. The program ends immediately. – "Too big" if the player has entered a number larger than the right price. – "Too small" if the player has entered a number smaller than the right price - The player has only 5 tries before the program ends by displaying the message 'Lost!'. If the player wins after X tries, the program should display "Won after X tries!". . at the end of each game, the message "Do you want to start again? (y/n)" is displayed. If the user types 'y', a new part of the game starts, if he types 'n', the program ends by displaying how many tries it took the player on average (over all the games…arrow_forward
- An integer number is said to be a perfect number if its factors, including 1 (but not the number itself), sum to the number. For example, 6 is a perfect number because 6 = 1 + 2 + 3. Write a function named perfect that determines if parameter number is a perfect number or not. Use this perfect function in a program that determines and prints all the perfect numbers between 1 and 1000.Also print the factors of each perfect number to confirm that the number is indeed perfect.arrow_forwardThe function below takes two arguments: a string (name) and an integer (position). Complete the function so that it prints out the a welcome statement that tells them person how many people are ahead of them in line. For example, your code should print out the following message if the person's name is Sally and their position is 24 (and hence have 23 people in front of them). Welcome Sally! There are 23 people, ahead of you in line.arrow_forwardWrite a function that takes a positive integer num and calculates how many dots exist in a pentagonal shape around the center dot on the Nth iteration. In the image below you can see the first iteration is only a single dot. On the second, there are 6 dots. On the third, there are 16 dots, and on the fourth there are 31 dots. Return the number of dots that exist in the whole pentagon on the Nth iteration. Examples pentagonal (1) → 1 pentagonal (2) <-6 pentagonal (3) - 16 pentagonal (8) → 141arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
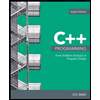
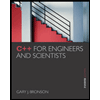