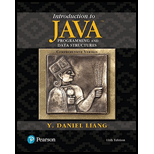
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 24.3, Problem 24.3.5CP
If you change the code in line 33 in Listing 24.2, MyArrayList.java, from
E[] newData = (E[])(new Object[size * 2 + 1]);
to
E[] newData = (E[])(new Object[size * 2]);
the
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
If a method given an ArrayList as a parameter calls a second method giving it the same ArrayList, and that second method deletes one of the elements, this will: (produce a non-deterministic result? trigger an error? have an effect? have no effect?) on the first ArrayList.
Java uses: (pass by reference? pass by value?) So when an object is passed to a method, what is actually passed is just : (the name of the object? a pointer to the object? the index of the object?) .
Select the correct answer
Please use java.
In this assignment, you will implement a class calledCustomIntegerArrayList. This class represents a fancy ArrayList that stores integers and supports additional operations not included in Java's built-in ArrayList methods.
For example, the CustomIntegerArrayList class has a “splice” method which removes a specified number of elements from the CustomIntegerArrayList, starting at a given index. For a CustomIntegerArrayList that includes: 1, 2, 3, 4, and 5, calling splice(1, 2) will remove 2 items starting at index 1. This will remove 2 and 3 (the 2nd and 3rd items).
The Cu stomIntegerArrayList class has 2 different (overloaded) constructors. (Remember, an overloaded constructor is a constructor that has the same name, but a different number, type, or sequence of parameters, as another constructor in the class.) Having 2 different constructors means you can create an instance of the CustomIntegerArrayList class in 2 different ways, depending on which constructor…
What is true after the execution of the following code?
ArrayList<String> arrayList = new ArrayList<String>();arrayList.add("a");arrayList.add("b");arrayList.add("c");arrayList.remove("b");
a.the arrayList will contain a, null, c
b.the arrayList will contain a, c
c.an exception is thrown
d.none of these is correct
Chapter 24 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 24.2 - Prob. 24.2.1CPCh. 24.2 - Prob. 24.2.2CPCh. 24.2 - Prob. 24.2.3CPCh. 24.2 - Prob. 24.2.4CPCh. 24.3 - What are the limitations of the array data type?Ch. 24.3 - Prob. 24.3.2CPCh. 24.3 - Prob. 24.3.3CPCh. 24.3 - What is wrong if lines 11 and 12 in Listing 24.2,...Ch. 24.3 - If you change the code in line 33 in Listing 24.2,...Ch. 24.3 - Prob. 24.3.6CP
Ch. 24.3 - Prob. 24.3.7CPCh. 24.4 - Prob. 24.4.1CPCh. 24.4 - Prob. 24.4.2CPCh. 24.4 - Prob. 24.4.3CPCh. 24.4 - Prob. 24.4.4CPCh. 24.4 - Prob. 24.4.5CPCh. 24.4 - Prob. 24.4.7CPCh. 24.4 - Prob. 24.4.8CPCh. 24.4 - Prob. 24.4.9CPCh. 24.4 - Prob. 24.4.10CPCh. 24.5 - Prob. 24.5.1CPCh. 24.5 - Prob. 24.5.2CPCh. 24.5 - Prob. 24.5.3CPCh. 24.6 - What is a priority queue?Ch. 24.6 - Prob. 24.6.2CPCh. 24.6 - Which of the following statements are wrong?...Ch. 24 - (Implement set operations in MyList) The...Ch. 24 - (Implement MyLinkedList) The implementations of...Ch. 24 - (Use the GenericStack class) Write a program that...Ch. 24 - Prob. 24.5PECh. 24 - Prob. 24.6PECh. 24 - (Fibonacci number iterator) Define an iterator...Ch. 24 - (Prime number iterator) Define an iterator class...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Implement a performance-measuring environment simulator for the vacuum-cleaner world depicted in Figure 2.2 and...
Artificial Intelligence: A Modern Approach
Write a pseudocode statement that assigns the sum of 10 and 14 to the variable total.
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
The ______ is the name of the structure type.
Starting Out with C++ from Control Structures to Objects (9th Edition)
In the following exercises, write a program to carry out the task. The program should use variables for each of...
Introduction to Programming Using Visual Basic (10th Edition)
Lottery Application Write a program that simulates a lottery. The program should have an array of five integers...
Starting Out with C++ from Control Structures to Objects (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 4. Say we wanted to get an iterator for an ArrayList and use it to loop over all items and print them to the console. What would the code look like for this? 5. Write a method signature for a method called foo that takes an array as an argument. The return type is void. 6. What is the difference between remove and clear in ArrayLists.arrow_forwardPlease use java. Show the steps with pictures. In this assignment, you will implement a class calledArrayAndArrayList. This class includes some interesting methods for working with Arrays and ArrayLists. For example, the ArrayAndArrayList class has a “findMax” method which finds and returns the max number in a given array. For a defined array: int[] array = {1, 3, 5, 7, 9}, calling findMax(array) will return 9. There are 4 methods that need to be implemented in the A rrayAndArrayList class: ● howMany(int[] array, int element) - Counts the number of occurrences of the given element in the given array. ● findMax(int[] array) - Finds the max number in the given array. ● maxArray(int[] array) - Keeps track of every occurrence of the max number in the given array. ● swapZero(int[] array) - Puts all of the zeros in the given array, at the end of the given array. Each method has been defined for you, but without the code. See the javadoc for each method for instructions on what the…arrow_forwardHello, can anyone help me with this problem. i want to run and compile this code, thank you.arrow_forward
- Please use java. In this assignment, you will implement a class calledArrayAndArrayList. This class includes some interesting methods for working with Arrays and ArrayLists. For example, the ArrayAndArrayList class has a “findMax” method which finds and returns the max number in a given array. For a defined array: int[] array = {1, 3, 5, 7, 9}, calling findMax(array) will return 9. There are 4 methods that need to be implemented in the A rrayAndArrayList class: ● howMany(int[] array, int element) - Counts the number of occurrences of the given element in the given array. ● findMax(int[] array) - Finds the max number in the given array. ● maxArray(int[] array) - Keeps track of every occurrence of the max number in the given array. ● swapZero(int[] array) - Puts all of the zeros in the given array, at the end of the given array. Each method has been defined for you, but without the code. See the javadoc for each method for instructions on what the method is supposed to do and how…arrow_forwardplease send solutionarrow_forwardI need anyone can check of my Hand class codes: "The getHandValue() method treats all Aces as having a value of 1. This needs to be corrected in the final version" Deck Class: import java.util.ArrayList;public class Hand { ArrayList<Card> hand; public Hand() { hand = new ArrayList<Card>(); } public void add(Card c) { hand.add(c); } public String toString() { String temp = "["; for (int i = 0; i < hand.size(); i++) { temp += hand.get(i); if (i < hand.size() - 1) { temp += ","; } } temp += "]"; return temp; } /* The getHandValue() method treats all Aces as having a value of 1. This needs to be corrected in the final version. */ public int getHandValue() { int total = 0; boolean hasAces = false; for (int i = 0; i < hand.size(); i++) { int value = hand.get(i).getValue(); if (value >…arrow_forward
- Write a Java application CountryList. In the main method, do the following:1. Create an array list of Strings called countries.2. Add "Canada", "India", "Mexico", "Peru" in that order.3. Use the enhanced for loop to print all countries in the array list, one per line.4. Add "Spain" at index 15. Replace the element at index 2 with "Vietnam". You must use the set method.6. Replace the next to the last element with "Brazil". You must use the set method. You willlose one point if you use 3 in the set method. Do this in a manner that would replace thenext to the last element, no matter the size of the array list.7. Remove the object "Canada" Do not remove at an index. Your code should work if"Canada" was at a different location. There is a version of remove method that willremove a specific object.8. Get and print the first element followed by "***"9. Call method toString() on countries to print all elements on one line.10. Use the enhanced for loop to print all countries in the array list,…arrow_forwardA team member submitted the following code for you to review. Identify at least 5 things that would improve the code: package edu.school; import java.util.ArrayList; import java.util.Collections; public class DriversLicenseRepository extends ArrayList<Object> { public List<Object> updateExpirationDate(License license, Date temp) { ArrayList list = new ArrayList(); for(Object v : this) { if((((License)v.getLicenseNo()) == license.getLicenseNo(){ list.add(v); } } return list; } public void recordVote(Ballot ballot, int voter) { ArrayList newList = new ArrayList<>(); Election election = ElectionManager.getInstance(); for(Object v : this) { if(((Voter)v.id) == voter) { election.addVote(ballot, (Voter)v); } newList.add(v); } clear(); addAll(newList); } public Voter getLicense(String i) throws IllegalArgumentException{ if(get(i) instanceof License) { return get(0); } throw new…arrow_forwardOnly number 5arrow_forward
- What is the output of the following Java code? ArrayList<String> names = new ArrayList<>(); names.add("James"); names.add("Mary"); names.add("Henry"); names.add("George"); names.add("Mary"); names.add("John"); names.add("Zachery"); Set names_wo_duplicate = new HashSet(names); Iterator set_val = names_wo_duplicate.iterator(); while(set_val.hasNext()) System.out.print(set_val.next() + ", "); Group of answer choices James, George, John, Zachery, Henry, Mary James, Mary, Henry, George, John, Zachery None of the above Code does not compile because of syntax errorsarrow_forwardWrite a Java program that uses ArrayList and Iterator. It should input from user the names and ages of your few friends in a loop and add into ArrayList. Finally, it should use an Iterator to display the data in a proper format. ( Sample run of the program:-) List of my Friends Enter name and age [friend# 0] Khalid Al-shamri 22.5 Do you want to add another friend (y/n)? y Enter name and age [friend# 1] Rahsed Al-anazi 21.1 Do you want to add another friend (y/n)? y Enter name and age [friend# 2] Salem Al-mutairi 23.7 Do you want to add another friend (y/n)? n Here is the data you entered: 0. Khalid Al-shamri, 22.5 1. Rahsed Al-anazi, 21.1 2. Salem Al-mutairi, 23.7arrow_forwardPLEASE MAKE IT RUN LIKE THE EXPECTED PICTUREarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
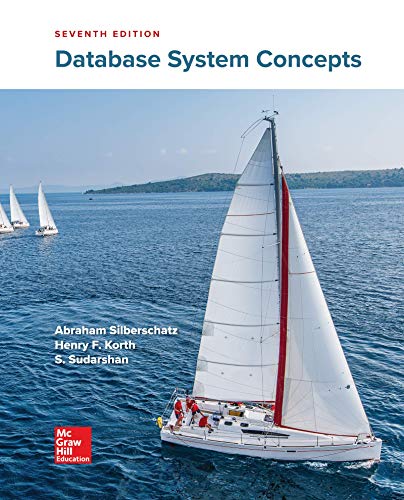
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
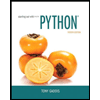
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
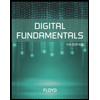
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
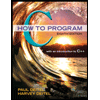
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
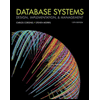
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
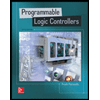
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Definition of Array; Author: Neso Academy;https://www.youtube.com/watch?v=55l-aZ7_F24;License: Standard Youtube License