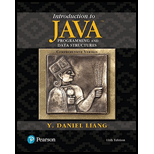
Concept explainers
(Implement set operations in MyList) The implementations of the methods addAll, removeAll, retainAll, toArray(), and toArray(T[]) are omitted in the MyList interface. Implement these methods. Test your new MyList class using the code at liveexample.pearsoncmg.com/test/ Exercise24_01Test.txt.

MyList
Program plan:
- Import required packages.
- Declare the main class named “Main”.
- Give the main method “public static void main ()”.
- Declare a default constructor and data members.
- Prompt the user to get the input.
- Print the value of list1 and list2.
- Print the result by calling the function “addAll()”.
- Print the value of list1 and list2.
- Print the result by calling the method “removeAll()”.
- Print the value of list1 and list2.
- Print the result by calling the method “retainAll()”.
- Print the value of list1 and list2.
- Print the result by calling the method “containsAll()”.
- Includes an interface “MyList”
- Define a method to add a new element at the specified index in the list.
- Define a method to return the element from this list at the specified index
- Define a method to return the index of the first matching element in this list.
- Define a method to return the index of the last matching element in this list
- Define a method to remove the element at the specified position in this list
- Define a method to replace the element at the specified position in this list
- Define a method to add a new element at the end of this list
- Define a method to return true if this list contains no elements
- Define a method to remove the first occurrence of the element e from this list
- Define a method to check whether the elements are present or not
- Define a method to add the elements in otherList to this list.
- Define a method to retains the elements in this list that are also in otherList
- Define a class “MyArrayList”.
- Declare a variable “sum” and assign 0 to it.
- Declare data members and default constructor.
- Create a list from an array of object.
- Define a method to add a new element at the specified index
- Define a method to create a larger array which is double the current size.
- Define a method to clear the list.
- Define a method to return true if the list contains the element.
- Define a method to return the element at the specified index.
- Define a method to trim the capacity to current size.
- Define a method to return the number of element in the list.
- Give the main method “public static void main ()”.
The below program includes the methods addAll(), removeAll(), retainAll(), toArray(), and toArray(T[]) which were omitted in the MyList interface.
Explanation of Solution
Program:
//import required packages
import java.util.*;
//class definition
public class Exercise24_01 {
//define main method
public static void main(String[] args) {
new Exercise24_01();
}
//default constructor
public Exercise24_01() {
Scanner input = new Scanner(System.in);
//data members
String[] name1 = new String[5];
String[] name2 = new String[5];
String[] name3 = new String[2];
//prompt user to get the input
System.out.print("Enter five strings for array name1 separated by space: ");
for (int i = 0; i < 5; i++) {
name1[i] = input.next();
}
//prompt the user to get the input
System.out.print("Enter five strings for array name2 separated by space: ");
for (int i = 0; i < 5; i++) {
name2[i] = input.next();
}
//prompt user to get the input
System.out.print("Enter two strings for array name3 separated by space: ");
for (int i = 0; i < 2; i++) {
name3[i] = input.next();
}
MyList<String> list1 = new MyArrayList<>(name1);
MyList<String> list2 = new MyArrayList<>(name2);
//print the value of list1
System.out.println("list1:" + list1);
//print the value of list2
System.out.println("list2:" + list2);
//calling addAll function
list1.addAll(list2);
//print the result
System.out.println("After addAll:" + list1 + "\n");
list1 = new MyArrayList<>(name1);
list2 = new MyArrayList<>(name2);
//print the value of list1
System.out.println("list1:" + list1);
//print the value of list2
System.out.println("list2:" + list2);
//calling removeAll function
list1.removeAll(list2);
//print the result
System.out.println("After removeAll:" + list1 + "\n");
list1 = new MyArrayList<>(name1);
list2 = new MyArrayList<>(name2);
//print the value of list1
System.out.println("list1:" + list1);
//print the value of list2
System.out.println("list2:" + list2);
//calling the retainAll function
list1.retainAll(list2);
//print the result
System.out.println("After retainAll:" + list1 + "\n");
list1 = new MyArrayList<>(name1);
list2 = new MyArrayList<>(name2);
//print the value of list1
System.out.println("list1:" + list1);
//print the value of list2
System.out.println("list2:" + list2);
//calling the retainAll function
list1.retainAll(list2);
//print the result
System.out.println("list1 contains all list2? " + list1.containsAll(list2) + "\n");
list1 = new MyArrayList<>(name1);
list2 = new MyArrayList<>(name3);
//print the value of list1
System.out.println("list1:" + list1);
//print the value of list2
System.out.println("list2:" + list2);
//print the result
System.out.println("list1 contains all list2? " + list1.containsAll(list2) + "\n");
Object[] name4 = list1.toArray();
//print the result
System.out.print("name4: ");
for (Object e: name4) {
System.out.print(e + " ");
}
String[] name5 = new String[list1.size()];
String[] name6 = list1.toArray(name5);
//print the result
System.out.print("\nname6: ");
for (Object e: name6) {
System.out.print(e + " ");
}
}
//includes the interface
public interface MyList<E> extends java.util.Collection<E> {
/* Add a new element at the specified index in this list */
public void add(int index, E e);
/*Return the element from this list at the specified index */
public E get(int index);
/*Return the index of the first matching element in this list. Return -1 if no match. */
public int indexOf(Object e);
/*Return the index of the last matching element in this list, Return -1 if no match. */
public int lastIndexOf(E e);
/* Remove the element at the specified position in this list, Shift any subsequent elements to the left. Return the element that was removed from the list. */
public E remove(int index);
/* Replace the element at the specified position in this list, with the specified element and returns the new set. */
public E set(int index, E e);
@Override /* Add a new element at the end of this list */
public default boolean add(E e) {
add(size(), e);
return true;
}
@Override /* Return true if this list contains no elements */
public default boolean isEmpty() {
return size() == 0;
}
@Override /* Remove the first occurrence of the element e from this list. Shift any subsequent elements to the left, Return true if the element is removed. */
public default boolean remove(Object e) {
if (indexOf(e) >= 0) {
remove(indexOf(e));
return true;
}
else
return false;
}
@Override
/*method to check whether the elements are present or not */
public default boolean containsAll(Collection<?> c) {
for (Object e: c)
if (!this.contains(e))
return false;
return true;
}
/* Adds the elements in otherList to this list.
Returns true if this list changed as a result of the call */
@Override
public default boolean addAll(Collection<? extends E> c) {
for (E e: c)
this.add(e);
return c.size() > 0;
}
/* Removes all the elements in otherList from this list Returns true if this list changed as a result of the call */
@Override
public default boolean removeAll(Collection<?> c) {
boolean changed = false;
for (Object e: c) {
if (remove(e))
changed = true;
}
return changed;
}
/* Retains the elements in this list that are also in otherList, Returns true if this list changed as a result of the call */
@Override
public default boolean retainAll(Collection<?> c) {
boolean changed = false;
for (int i = 0; i < this.size(); ) {
if (!c.contains(this.get(i))) {
this.remove(get(i));
changed = true;
}
else
i++;
}
return changed;
}
@Override
public default Object[] toArray() {
// Left as an exercise
Object[] result = new Object[size()];
for (int i = 0; i < size(); i++) {
result[i] = this.get(i);
}
//return statement
return result;
}
@Override
public default <T> T[] toArray(T[] a) {
// Left as an exercise
for (int i = 0; i < size(); i++) {
a[i] = (T)this.get(i);
}
//return statement
return a;
}
}
//class definition
public class MyArrayList<E> implements MyList<E> {
//data members
public static final int INITIAL_CAPACITY = 16;
private E[] data = (E[])new Object[INITIAL_CAPACITY];
private int size = 0;
// Create an empty list
public MyArrayList() {
}
//Create a list from an array of objects
public MyArrayList(E[] objects) {
for (int i = 0; i < objects.length; i++)
add(objects[i]);
}
@Override /* Add a new element at the specified index */
public void add(int index, E e) {
ensureCapacity();
/* Move the elements to the right after the specified index */
for (int i = size - 1; i >= index; i--)
data[i + 1] = data[i];
// Insert new element to data[index]
data[index] = e;
// Increase size by 1
size++;
}
/*Create a new larger array, double the current size + 1 */
private void ensureCapacity() {
if (size >= data.length) {
E[] newData = (E[])(new Object[size * 2 + 1]);
System.arraycopy(data, 0, newData, 0, size);
data = newData;
}
}
@Override // Clear the list
public void clear() {
data = (E[])new Object[INITIAL_CAPACITY];
size = 0;
}
@Override /*Return true if this list contains the element */
public boolean contains(Object e) {
for (int i = 0; i < size; i++)
if (e.equals(data[i])) return true;
return false;
}
@Override /*Return the element at the specified index */
public E get(int index) {
checkIndex(index);
return data[index];
}
private void checkIndex(int index) {
if (index < 0 || index >= size)
throw new IndexOutOfBoundsException
("Index: " + index + ", Size: " + size);
}
@Override /*Return the index of the first matching element in this list. Return -1 if no match. */
public int indexOf(Object e) {
for (int i = 0; i < size; i++)
if (e.equals(data[i])) return i;
return -1;
}
@Override /*Return the index of the last matching element in this list. Return -1 if no match. */
public int lastIndexOf(E e) {
for (int i = size - 1; i >= 0; i--)
if (e.equals(data[i])) return i;
return -1;
}
@Override /* Remove the element at the specified position in this list. Shift any subsequent elements to the left. Return the element that was removed from the list. */
public E remove(int index) {
checkIndex(index);
E e = data[index];
// Shift data to the left
for (int j = index; j < size - 1; j++)
data[j] = data[j + 1];
data[size - 1] = null;
// Decrement size
size--;
return e;
}
@Override /*Replace the element at the specified position in this list with the specified element. */
public E set(int index, E e) {
checkIndex(index);
E old = data[index];
data[index] = e;
return old;
}
@Override
public String toString() {
StringBuilder result = new StringBuilder("[");
for (int i = 0; i < size; i++) {
result.append(data[i]);
if (i < size - 1) result.append(", ");
}
return result.toString() + "]";
}
// Trims the capacity to current size
public void trimToSize() {
if (size != data.length) {
E[] newData = (E[])(new Object[size]);
System.arraycopy(data, 0, newData, 0, size);
data = newData;
} // If size == capacity, no need to trim
}
@Override /*Override iterator() defined in Iterable */
public java.util.Iterator<E> iterator() {
return new ArrayListIterator();
}
private class ArrayListIterator
implements java.util.Iterator<E> {
// Current index
private int current = 0;
@Override
public boolean hasNext() {
return (current < size);
}
@Override
public E next() {
return data[current++];
}
@Override
public void remove() {
MyArrayList.this.remove(current);
}
}
@Override /* Return the number of elements in this list */
public int size() {
return size;
}
}
}
Enter five strings for array name1 separated by space: tom george peter jean jane
Enter five strings for array name2 separated by space: tom george michael michelle daniel
Enter two strings for array name3 separated by space: tom peter
list1:[tom, george, peter, jean, jane]
list2:[tom, george, michael, michelle, daniel]
After addAll:[tom, george, peter, jean, jane, tom, george, michael, michelle, daniel]
list1:[tom, george, peter, jean, jane]
list2:[tom, george, michael, michelle, daniel]
After removeAll:[peter, jean, jane]
list1:[tom, george, peter, jean, jane]
list2:[tom, george, michael, michelle, daniel]
After retainAll:[tom, george]
list1:[tom, george, peter, jean, jane]
list2:[tom, george, michael, michelle, daniel]
list1 contains all list2? false
list1:[tom, george, peter, jean, jane]
list2:[tom, peter]
list1 contains all list2? true
name4: tom george peter jean jane
name6: tom george peter jean jane
Want to see more full solutions like this?
Chapter 24 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Introduction To Programming Using Visual Basic (11th Edition)
Mechanics of Materials (10th Edition)
Database Concepts (8th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- Provide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forwardComplete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forward
- Solve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forwardInformation Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forward
- Could you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forwardFor this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
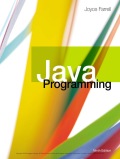
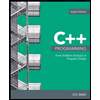
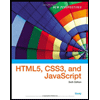
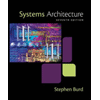