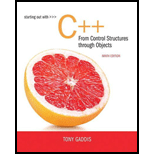
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 21, Problem 9RQE
A node without child is referred a “leaf node”.
Program Plan Intro
Binary tree:
A complete binary tree is a binary tree with the property that every node must have exactly two children, and at the last level the nodes should be from left to right.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
You were requested to design IP addresses for the following network using the address
block 10.10.10.0/24. Specify an address and net mask for each network and router interface
For the following network, propose routing tables in each of the routers R1 to R5
For the following network, propose routing tables in each of the routers R1 to R5
Chapter 21 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 21.1 - Prob. 21.1CPCh. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21.1 - Prob. 21.4CPCh. 21.1 - Prob. 21.5CPCh. 21.1 - Prob. 21.6CPCh. 21.2 - Prob. 21.7CPCh. 21.2 - Prob. 21.8CPCh. 21.2 - Prob. 21.9CPCh. 21.2 - Prob. 21.10CP
Ch. 21.2 - Prob. 21.11CPCh. 21.2 - Prob. 21.12CPCh. 21 - Prob. 1RQECh. 21 - Prob. 2RQECh. 21 - Prob. 3RQECh. 21 - Prob. 4RQECh. 21 - Prob. 5RQECh. 21 - Prob. 6RQECh. 21 - Prob. 7RQECh. 21 - Prob. 8RQECh. 21 - Prob. 9RQECh. 21 - Prob. 10RQECh. 21 - Prob. 11RQECh. 21 - Prob. 12RQECh. 21 - Prob. 13RQECh. 21 - Prob. 14RQECh. 21 - Prob. 15RQECh. 21 - Prob. 16RQECh. 21 - Prob. 17RQECh. 21 - Prob. 18RQECh. 21 - Prob. 19RQECh. 21 - Prob. 20RQECh. 21 - Prob. 21RQECh. 21 - Prob. 22RQECh. 21 - Prob. 23RQECh. 21 - Prob. 24RQECh. 21 - Prob. 25RQECh. 21 - Prob. 1PCCh. 21 - Prob. 2PCCh. 21 - Prob. 3PCCh. 21 - Prob. 4PCCh. 21 - Prob. 5PCCh. 21 - Prob. 6PCCh. 21 - Prob. 7PCCh. 21 - Prob. 8PC
Knowledge Booster
Similar questions
- Using R language. Here is the information link. http://www.cnachtsheim-text.csom.umn.edu/Kutner/Chapter%20%206%20Data%20Sets/CH06PR18.txtarrow_forwardUsing R languagearrow_forwardHow can I type the Java OOP code by using JOptionPane with this following code below: public static void sellCruiseTicket(Cruise[] allCruises) { //Type the code here }arrow_forward
- Draw a system/level-0 diagram for this scenario: You are developing a new customer relationship management system for the BEC store, which rents out movies to customers. Customers will provide comments on new products, and request rental extensions and new products, each of which will be stored into the system and used by the manager for purchasing movies, extra copies, etc. Each month, one employee of BEC will select their favorite movie pick of that week, which will be stored in the system. The actual inventory information will be stored in the Entertainment Tracker system, and would be retrieved by this new system as and when necessary. Example of what a level-0 diagram looks like is attached.arrow_forwardWhat is the value of performing exploratory data analysis in designing data visualizations? What are some examples?arrow_forwardDraw a level-0 diagram for this scenario: You are developing a new customer relationship management system for the BEC store, which rents out movies to customers. Customers will provide comments on new products, and request rental extensions and new products, each of which will be stored into the system and used by the manager for purchasing movies, extra copies, etc. Each month, one employee of BEC will select their favorite movie pick of that week, which will be stored in the system. The actual inventory information will be stored in the Entertainment Tracker system, and would be retrieved by this new system as and when necessary.arrow_forward
- Draw a context diagram for this scenario: You are developing a new customer relationship Management system for the BEC store, which rents out movies to customers. Customers will provide comments on new products, and request rental extensions and new products, each of which will be stored into the system and used by the manager for purchasing movies, extra copies, etc. Each month, one employee of BEC will select their favorite movie pick of that week, which will be stored in. the system. The actual inventory information will be stored in the Entertainment Tracker system, and would be retrieved by this new system as and when necessary.arrow_forwardWrite a complete Java program named FindSumAndAverage that performs the following tasks in 2-D array: Main Method: a. The main() method asks the user to provide the dimension n for a square matrix. A square matrix has an equal number of rows and columns. b. The main() method receives the value of n and calls the matrixSetUp() method that creates a square matrix of size n and populates it randomly with integers between 1 and 9. c. The main method then calls another method named printMatrix() to display the matrix in a matrix format. d. The main method also calls a method named findSumAndAverage() which: • Receives the generated matrix as input. • Calculates the sum of all elements in the matrix. • Calculates the average value of the elements in the matrix. • Stores these values (sum and average) in a single-dimensional array and returns this array • e. The main method prints the sum and average based on the result returned from findSumAndAverage()). Enter the dimension n for the square…arrow_forwardThe partial sums remain the same no matter what indexing we done to s artial sum of each series onverges, * + s of each series to the series or show 12. (1)+(0)+(0)+(+1)+ 17, " (F) + (F) + (F)(F)(- 18. 19. 1 #20. (三)+(三)-(三)+(3) 20 (9)-(0)-(0)-- 10 +1 2.1+(男)+(男)+(罰)+(鄂 9 T29 x222-끝+1-23 + -.... Repeating Decimals 64 Express each of the numbers in Exercises 23-30 as the m integers. 23. 0.23 = 0.23 23 23... 24. 0.234 = 0.234 234 234. 25. 0.7 = 0.7777... 26. 0.d = 0.dddd... where d is a digit natio of own s converges or * 27. 0.06 = 0.06666.. 28. 1.4141.414 414 414... 29. 1.24123 = 1.24 123 123 123... 30. 3.142857 = 3.142857 142857. Using the ath-Term Test In Exercises 31-38, use the ath-Term Test for divergence to show that the series is divergent, or state that the test is inconclusive 8arrow_forward
- CPS 2231 Computer Programming Homework #3 Due Date: Posted on Canvas 1. Provide answers to the following Check Point Questions from our textbook (5 points): a. How do you define a class? How do you define a class in Eclipse? b. How do you declare an object's reference variable (Hint: object's reference variable is the name of that object)? c. How do you create an object? d. What are the differences between constructors and regular methods? e. Explain why we need classes and objects in Java programming. 2. Write the Account class. The UML diagram of the class is represented below (10 points): Account id: int = 0 - balance: double = 0 - annualInterestRate: double = 0.02 - dateCreated: java.util.Date + Account() + Account(id: int, balance: double) + getId(): int + setId(newId: int): void + getBalance(): double + setBalance(newBalance: double): void + getAnnualInterestRate(): double + setAnnualInterest Rate (newRate: double): void + toString(): String + getDataCreated(): java.util.Date +…arrow_forwardTHIS IS NOT A GRADING ASSIGNMENT: Please only do lab 2.2 (bottom part of the first picture) For that Lab 2.2 do: *Part 1 (do the CODE, that's super important I need it) *Part 2 *Part 3 I also attached Section 2.5.2 which is part of the step 1 so you can read what is it about. Thank you!arrow_forwardTHIS IS NOT A GRADING ASSIGNMENT: Please only do lab 2.2 (bottom part of the first picture) For that Lab 2.2 do: *Part 1 *Part 2 *Part 3 I also attached Section 2.5.2 which is part of the step 1 so you can read what is it about. Thank you!arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
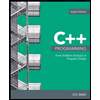
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
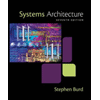
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
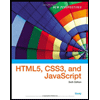
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
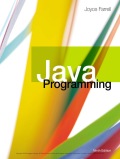
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
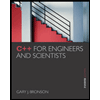
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage