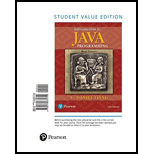
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
11th Edition
ISBN: 9780134671710
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 20.7, Problem 20.7.6CP
Explanation of Solution
max() method for array of comparable objects:
The max() is used to determine the largest element in an array using comparator.
The Syntax is given below:
Collections.max(list);
For Example:
LinkedList<String> l = new LinkedList<>();
l.add(20);
l.add(10);
l.add(25);
Colections...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
2. Creates an array of Triangle type of size 10. Prompts the user to enter the index of the array, then
displays the area of corresponding element value. If the specified index is out of bounds, display
the message Out of Bounds.
4. Declare a variable as an array of 20 MyStruct objects.
Problem2
Write a program that display the position of a given element in an array. You should print the index (i.e. the
position) of the element. If the element appears more than one time than you should print all its positions.
The size of the array should be entered by the user. If the element does not occur then you should display
element not found.
Sample1:
Enter the size of the array: 5
Enter an array of size 5: 44 5 13 44 67
Enter the element to find: 44
44 is found at position
44 is found at position
44 occurs 2 time(s)
Sample2:
Enter the size of the array: 4
Enter an array of size 4: 12 150 17 20
Enter the element: 18
18 is not found
Chapter 20 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Ch. 20.2 - Prob. 20.2.1CPCh. 20.2 - Prob. 20.2.2CPCh. 20.2 - Prob. 20.2.3CPCh. 20.2 - Prob. 20.2.4CPCh. 20.2 - Prob. 20.2.5CPCh. 20.3 - Prob. 20.3.1CPCh. 20.3 - Prob. 20.3.2CPCh. 20.3 - Prob. 20.3.3CPCh. 20.3 - Prob. 20.3.4CPCh. 20.4 - Prob. 20.4.1CP
Ch. 20.4 - Prob. 20.4.2CPCh. 20.5 - Prob. 20.5.1CPCh. 20.5 - Suppose list1 is a list that contains the strings...Ch. 20.5 - Prob. 20.5.3CPCh. 20.5 - Prob. 20.5.4CPCh. 20.5 - Prob. 20.5.5CPCh. 20.6 - Prob. 20.6.1CPCh. 20.6 - Prob. 20.6.2CPCh. 20.6 - Write a lambda expression to create a comparator...Ch. 20.6 - Prob. 20.6.4CPCh. 20.6 - Write a statement that sorts an array of Point2D...Ch. 20.6 - Write a statement that sorts an ArrayList of...Ch. 20.6 - Write a statement that sorts a two-dimensional...Ch. 20.6 - Write a statement that sorts a two-dimensional...Ch. 20.7 - Are all the methods in the Collections class...Ch. 20.7 - Prob. 20.7.2CPCh. 20.7 - Show the output of the following code: import...Ch. 20.7 - Prob. 20.7.4CPCh. 20.7 - Prob. 20.7.5CPCh. 20.7 - Prob. 20.7.6CPCh. 20.8 - Prob. 20.8.1CPCh. 20.8 - Prob. 20.8.2CPCh. 20.8 - Prob. 20.8.3CPCh. 20.9 - How do you create an instance of Vector? How do...Ch. 20.9 - How do you create an instance of Stack? How do you...Ch. 20.9 - Prob. 20.9.3CPCh. 20.10 - Prob. 20.10.1CPCh. 20.10 - Prob. 20.10.2CPCh. 20.10 - Prob. 20.10.3CPCh. 20.11 - Can the EvaluateExpression program evaluate the...Ch. 20.11 - Prob. 20.11.2CPCh. 20.11 - If you enter an expression "4 + 5 5 5", the...Ch. 20 - (Display words in ascending alphabetical order)...Ch. 20 - (Store numbers in a linked list) Write a program...Ch. 20 - (Guessing the capitals) Rewrite Programming...Ch. 20 - (Sort points in a plane) Write a program that...Ch. 20 - (Combine colliding bouncing balls) The example in...Ch. 20 - (Game: lottery) Revise Programming Exercise 3.15...Ch. 20 - Prob. 20.9PECh. 20 - Prob. 20.10PECh. 20 - (Match grouping symbols) A Java program contains...Ch. 20 - Prob. 20.12PECh. 20 - Prob. 20.14PECh. 20 - Prob. 20.16PECh. 20 - (Directory size) Listing 18.10,...Ch. 20 - Prob. 20.20PECh. 20 - (Nonrecursive Tower of Hanoi) Implement the...Ch. 20 - Evaluate expression Modify Listing 20.12,...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Declare a MyStruct variable and initialize the second field to an array containing all zeros.arrow_forwardGIven the statement below: intA=(3,6,9}, A.length can't be used to display the number of elements in the array True Falsearrow_forwardYou can copy all elements of one array into one another with an assignment statement. True or Falsearrow_forward
- 1. Create a struct called Booking that consists of a 3 digit flight number (e.g. 234), type of seat (E or B), the priceof a seat in economic class and the number of seats booked.Declare an array to store at least 30 Booking structs. (4)2. The user must be able to enter the information for a number of bookings from the keyboard. Ask whether abooking must be made (Y or N). If a booking must be made, a random 3 digit flight number must be generated.The user must be asked to enter the type of seat, the price per seat and the number of seats to book.3. Write code to display a numbered list with all the information of all the bookings. The price per ticket must bedisplayed with 2 decimal places. The output must be displayed with a heading and subheading 4. Write code to calculate and display the income earned per booking. Display headings and subheadings.Also count and display the number of business seats and economics seats booked as well as the incomeearned from each of these types of…arrow_forwardDescribe how to make an array of struct elements.arrow_forward1. Create a struct called Booking that consists of a 3 digit flight number (e.g. 234), type of seat (E or B), the priceof a seat in economic class and the number of seats booked.Declare an array to store at least 30 Booking structs. 2. The user must be able to enter the information for a number of bookings from the keyboard. Ask whether abooking must be made (Y or N). If a booking must be made, a random 3 digit flight number must be generated.The user must be asked to enter the type of seat, the price per seat and the number of seats to book.Example of input:arrow_forward
- Write the statement that declares and instantiates a two dimensional array with 12 rows and 5 columns of type int using the identifier fiveYearPlan.arrow_forwardAn array definition reserves space for the array. true or falsearrow_forwardWrite a statement that assigns the number of elements in the intOrders array to the intNum variable.arrow_forward
- An array's index type may be any form of data. Do you think this is true or not?arrow_forwardint[] F = new int[25];Which of the following is not true in the above declaration? a. The index value of 10th element is 9 b. The index value of last element 25 c. Maximum number of elements in this array is 25 d. The length of the array is 25arrow_forwardint Marks [20] = { 70,85, 60, 55,90,100,50}; For the above code, answer the following questions in the space provided below: 1. Find the size of Marks array. 2. How many number of elements are present in Marks array ? 3. Find the index value for last element in array 4. Find the index value for the element 60 5. Find the value of Marks[2] + Marks[0] in Marks array.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
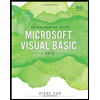
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
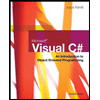
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
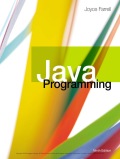
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT