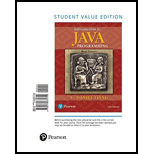
Explanation of Solution
Create list from array of objects:
asList() method is used to create list from a array of objects.
- asList() returns the list of the specified array.
- This method is used to act as the bridge between array elements and collections API (Application
Programming interface) such as ArrayList and Linked List.
Syntax for asList() method:
asList(objectName)
Sample Program:
//Import the required packages
import java.util.ArrayList;
import java.util.Arrays;
import java.util.LinkedList;
import java.util.List;
//Class definition
public class ListfromArrayobjects
{
//Main method
public static void main(String[] args)
{
// Creating String ArrayObject
String[] ArrayObj = new String[]{"Example","for","asList"};
System.out.println("Before Array object converted to ArrayList:");
/*Printing Arrayobjects before converting it to ArrayList */
for(String s: ArrayObj)
System.out.println(s);
/*Converting Array Object to ArrayList using asList */
ArrayList<String> AL = new ArrayList<String>(Arrays...

Want to see the full answer?
Check out a sample textbook solution
Chapter 20 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
- Lab Goal : This lab was designed to teach you more about list processing and algorithms.Lab Description : Write a program that will search a list to find the first odd number. If an odd number is found, then find the first even number following the odd number. Return the distance between the first odd number and the LAST even number. Return -1 if no odd numbers are found or there are no even numbers following an odd numberarrow_forwardAn array's index type may be any form of data. Do you think this is true or not?arrow_forwardWhat is difference between Array and Array List? When will you use Array over Array List?arrow_forward
- flip_matrix(mat:list)->list You will be given a single parameter a 2D list (A list with lists within it) this will look like a 2D matrix when printed out, see examples below. Your job is to flip the matrix on its horizontal axis. In other words, flip the matrix horizontally so that the bottom is at top and the top is at the bottom. Return the flipped matrix. To print the matrix to the console: print('\n'.join([''.join(['{:4}'.format(item) for item in row]) for row in mat])) Example: Matrix: W R I T X H D R L G L K F M V G I S T C W N M N F Expected: W N M N F G I S T C L K F M V H D R L G W R I T X Matrix: L C S P Expected: S P L C Matrix: A D J A Q H J C I Expected: J C I A Q H A D Jarrow_forwardHomework 3: Create Array List with the following: 1. Name : Cars from type String. 2. Add these elements to the array List: BMW, Toyota, Lexus, Broche. 3. Write statement to check if Honda in the list or not. 4. Write statement to return the size of the list.arrow_forwardHow do you keep tabs on the elements with data in an array that is only partially filled?arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
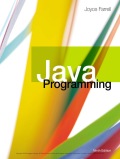