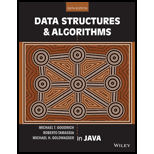
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 2, Problem 31P
Program Plan Intro
Ecosystem
Program plan:
- Import the required java packages.
- Create the class Animal,
- Declare the array variable to fill the river.
- Declare the constructor.
- Define the print() function to read the size of river.
- Define the printl() function to read the length of array.
- Define the leftMove() function to fill ecosystem of river using bear, fish and null values with the help of random function.
- Define the rightMove() function to fill ecosystem of river using bear, fish and null values with the help of random function.
- Define the method check() to check the length of array elements and based on the value it calls the function to fill the ecosystem.
- Define the main() function,
- Declare the object for random() function.
- Declare the object for Animal class.
- Read the input using scanner class.
- Fill the ecosystem with bear, fish and null values using the random() function.
- Read the number of time steps.
- Print the simulation ecosystem after each step.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I need help to solve the following case, thank you
hi I would like to get help to resolve the following case
Could you help me to know features of the following concepts:
- defragmenting.
- dynamic disk.
- hardware RAID
Chapter 2 Solutions
Data Structures and Algorithms in Java
Ch. 2 - Give three examples of life-critical software...Ch. 2 - Give an example of a software application in which...Ch. 2 - Prob. 3RCh. 2 - Prob. 4RCh. 2 - Prob. 5RCh. 2 - Give a short fragment of Java code that uses the...Ch. 2 - Prob. 7RCh. 2 - Prob. 8RCh. 2 - Prob. 9RCh. 2 - Prob. 10R
Ch. 2 - Prob. 11RCh. 2 - Draw a class inheritance diagram for the following...Ch. 2 - Prob. 13RCh. 2 - Prob. 14RCh. 2 - If the parameter to the makePayment method of the...Ch. 2 - Prob. 16CCh. 2 - Most modern Java compilers have optimizers that...Ch. 2 - The PredatoryCreditCard class provides a...Ch. 2 - Modify the PredatoryCreditCard class so that a...Ch. 2 - Prob. 20CCh. 2 - Write a program that consists of three classes, A,...Ch. 2 - Prob. 22CCh. 2 - Prob. 23CCh. 2 - Write a Java class that extends the Progression...Ch. 2 - Redesign the Progression class to be abstract and...Ch. 2 - Use a solution to Exercise C-2.25 to create a new...Ch. 2 - Use a solution to Exercise C-2.25 to reimplement...Ch. 2 - Write a set of Java classes that can simulate an...Ch. 2 - Write a Java program that inputs a polynomial in...Ch. 2 - Write a Java program that inputs a document and...Ch. 2 - Prob. 31PCh. 2 - Write a Java program that simulates a system that...Ch. 2 - Define a Polygon interface that has methods area()...Ch. 2 - Prob. 35PCh. 2 - Write a Java program that can make change. Your...
Knowledge Booster
Similar questions
- what is a feature in the Windows Server Security Compliance Toolkit, thank you.arrow_forwardYou will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forward
- Show all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forwardQuestion 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
- Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forwardI need help to solve the following, thank youarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
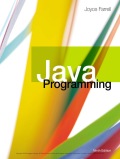
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
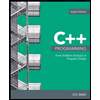
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
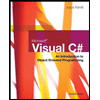
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
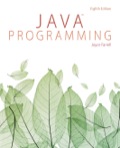
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
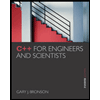
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr