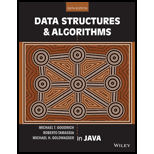
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 2, Problem 19C
Modify the PredatoryCreditCard class so that a customer is assigned a minimum monthly payment, as a percentage of the balance, and so that a late fee is assessed if the customer does not subsequently pay that minimum amount before the next monthly cycle.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Program this
For this exercise, we are going to do a variation on our Student class by looking at a StudentTest class. The StudentTest class stores math and ELA test scores for a student.
We will then extend this class in the HSStudent class, which will check the math and ELA scores to determine if the student has passed. Combined with service hours, the HSStudent class will also check to see if the student has qualified for graduation.
Details for each class are outlined in the starter code for each class.
In the StudentTester class, you will prompt the user for test scores and service hours, then use these to create a HSStudent object. After creating the object, print out the results.
Please enter the student name: Ryan
Please enter the Math Score: 500
Please enter the ELA Score: 600
Please enter the Service Hours: 100
Pass Math? false
Pass ELA? true
Completed Service Hours? true
Ryan has not yet qualified for graduation.
import java.util.Scanner;
public class StudentTester{public static…
describe MobilePhone class. You are required to apply good
principle in designing the class. Using knowledge in Single Responsibility
Principle (SRP), Multiple Responsibly Principle (MRP) and Don't Repeat
yourself (DRY), answer the following question.
MobilePhone
phoneNo: long
getPhoneNo0: long
makeCall(long)
rcvCall): Íong
createTxt(): String
sendTxt(String)
readTxt(String)
takePix)
sendPix(Picture)
rcvPix(Picture)
browse)
initialize()
connectToNetwork(long)
: MobilePhone class
Differentiate between Single Responsibility (SRP) and Multiple
Responsibly (MRP).
Apply SRP analysis technique to the current MobilePhone class.
Design new MobilePhone class after modified using SRP analysis
technique.
Chapter 2 Solutions
Data Structures and Algorithms in Java
Ch. 2 - Give three examples of life-critical software...Ch. 2 - Give an example of a software application in which...Ch. 2 - Prob. 3RCh. 2 - Prob. 4RCh. 2 - Prob. 5RCh. 2 - Give a short fragment of Java code that uses the...Ch. 2 - Prob. 7RCh. 2 - Prob. 8RCh. 2 - Prob. 9RCh. 2 - Prob. 10R
Ch. 2 - Prob. 11RCh. 2 - Draw a class inheritance diagram for the following...Ch. 2 - Prob. 13RCh. 2 - Prob. 14RCh. 2 - If the parameter to the makePayment method of the...Ch. 2 - Prob. 16CCh. 2 - Most modern Java compilers have optimizers that...Ch. 2 - The PredatoryCreditCard class provides a...Ch. 2 - Modify the PredatoryCreditCard class so that a...Ch. 2 - Prob. 20CCh. 2 - Write a program that consists of three classes, A,...Ch. 2 - Prob. 22CCh. 2 - Prob. 23CCh. 2 - Write a Java class that extends the Progression...Ch. 2 - Redesign the Progression class to be abstract and...Ch. 2 - Use a solution to Exercise C-2.25 to create a new...Ch. 2 - Use a solution to Exercise C-2.25 to reimplement...Ch. 2 - Write a set of Java classes that can simulate an...Ch. 2 - Write a Java program that inputs a polynomial in...Ch. 2 - Write a Java program that inputs a document and...Ch. 2 - Prob. 31PCh. 2 - Write a Java program that simulates a system that...Ch. 2 - Define a Polygon interface that has methods area()...Ch. 2 - Prob. 35PCh. 2 - Write a Java program that can make change. Your...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Porter’s competitive forces model: The model is used to provide a general view about the firms, the competitors...
Management Information Systems: Managing The Digital Firm (16th Edition)
On the basis of a computer system with which you are familiar, identify two units of application software and t...
Computer Science: An Overview (12th Edition)
What is an object? What is a control?
Starting Out With Visual Basic (8th Edition)
Write a program that uses a HashMap to compute a histogram of positive numbers entered by the user. The HashMap...
Java: An Introduction to Problem Solving and Programming (7th Edition)
When a 1 is on the input of an inverter, what is the output?
Digital Fundamentals (11th Edition)
Rewrite the following program segment using a single case statement instead of nested if-else statements. if (W...
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Now we are going to use the design pattern for collecting objects. We are going to create two classes, a class AmazonOrder that models Amazon orders and a class Item that models items in Amazon orders. An item has a name and a price, and the name is unique. The Item class has a constructor that takes name and price, in that order. The class also has getters and setters for the instance variables. This is the design pattern for managing properties of objects. The setName() method should do nothing if the parameter is the empty string, and the setPrice() method should do nothing if the parameter is not positive. The class also has a toString() method that returns a string representation for the item in the format “Item[Name:iPad,Price:399.99]”. For simplicity, we assume an Amazon order can have at most 5 items, and class AmazonOrder has two instance variables, an array of Item with a length of 5 and an integer numOfItems to keep track of the number of items in the…arrow_forwardFor this problem, we are going to revisit the Online Company exercise from lesson 3. In lesson 3, we created 3 classes, a superclass Company, a subclass OnlineCompany and a runner class CompanyTester. You can take your solutions from lesson 3 for the Company and OnlineCompany, but we are going to redesign the CompanyTester in this exercise. Your task is to create a loop that will allow users to enter companies that will then get stored in an ArrayList. You should first prompt users for the company name. If the user enters exit, then the program should exit and print the object using the toString. After prompting for the name, you prompt the user if it is an online company. If so, ask for a website address, otherwise ask for the street address, city, and state. You will then create the Company or OnlineCompany object and insert it into the ArrayList. Sample output: Please enter a company name, enter 'exit' to quit: CodeHS Is this an online company, 'yes' or 'no': yes Please enter the…arrow_forwardAfter implementing the required classes, design and implement a testing class to test them as follows: Create at least 7 groups of at least 3 sports and add them to the collection that stores the whole data then add and remove some kids from them. Try to violate the state of the objects and show that your code prevents all violations. Show that the other operations that happen frequently are working fine. At the end, the whole data should be saved into a text file and this file should be saved automatically inside the folder contains your Java project.arrow_forward
- It's messy that in the Balrog class's getDamage() function and the Cyberdemon class's getDamage() function we have to write the name of the species before calling the Demon class's getDamage() function. It would be better if the Demon class's getDamage() function could print the name of the species. Taking this a step further, it would be even better if we didn't have to repeat the cout statement "The <whatever> attacks for ?? points!" in every class's getDamage() function. It would be better if that cout statement could occur just once, in the Creature class's getDamage() function. In the Creature class's getDamage() function, insert the following statement: cout << "The " << getSpecies() << " attacks for " << damage << " points!" << endl; Delete (or, if you prefer, comment out) the similar cout statements that appear in the getDamage() function of each of the 5 derived classes. (There will be one such cout statement to delete in each of…arrow_forwardIt's messy that in the Balrog class's getDamage() function and the Cyberdemon class's getDamage() function we have to write the name of the species before calling the Demon class's getDamage() function. It would be better if the Demon class's getDamage() function could print the name of the species. Taking this a step further, it would be even better if we didn't have to repeat the cout statement "The <whatever> attacks for ?? points!" in every class's getDamage() function. It would be better if that cout statement could occur just once, in the Creature class's getDamage() function. In the Creature class's getDamage() function, insert the following statement:cout << "The " << getSpecies() << " attacks for " << damage << " points!" << endl; Delete (or, if you prefer, comment out) the similar cout statements that appear in the getDamage() function of each of the 5 derived classes. (There will be one such cout statement to delete in each of the 5…arrow_forwardHelp, I am making a elevator simulation using polymorphism. I am not sure to do now. Any help would be appreciated. My code is at the bottom, that's all I could think off. There are 4 types of passengers in the system:Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements.VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators.Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators.Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. public abstract class Passenger { public static int passangerCounter = 0; private String passengerID; protected…arrow_forward
- The Fraction Class – Immutable Classes In this section, we’ll build another Fraction class that is unchangeable once initialized and uses the keyword final for its numerator and denominator. Such a Fraction object will have all of its data declared final, and is our first example of building an immutable class. Once a Fraction object is built, its data items will never change for the lifetime of the object. Another way to view this is that the object is completely read-only. As a result, its data will also be declared public, which is the only example of public data you’ll find in this quarter. If you wish to change a fraction object’s numerator or denominator, the old object must be discarded and a new object created in its place. Again, this object’s data will be immutable, constant, non-variable, unchangeable, non-editable, or read-only. So, to add two fractions, our add function will return a new Fraction object that is the sum of the two previous (unchangeable) fractions we wish…arrow_forwardYou are to write an Ant class to simulate Langton's Ant. In this simulation, a virtual "ant" moves about a two-dimensional square matrix, whose spaces can be designated white or black, according to the rules below: If the ant is on a white space, it will turn right 90 degrees relative to the directions it is facing, move forward one step, and change the space it left to black. If the ant is on a black space, it will turn left 90 degrees relative to the directions it is facing, move forward one step, and change the space it left to white. When the ant hits the edge of the board and is going to go out of the bounds, wrap the board around so the ant will appear on the other side. The matrix is initially composed of all white spaces. The user will specify the size of the square matrix. The user will also specify the number of steps that the ant will move and the starting location and direction of the ant. After the simulation, the final board with the ant position will be displayed to…arrow_forwardRisking telling the obvious, you will need to make all necessary modifications to the files, including adapting the documentation and removing any parts that are irrelevant. I suggest that you pattern after the way the suggested book authors document a template class (such as for bag4) when adapting the documentation.arrow_forward
- Write a program that defines a class with a data member to holds a “serial number” for each object created from the class. That is, the first object created will be numbered 1, the second 2, and so on. To do this, you’ll need another data member that records a count of how many objects have been created so far. (This member should apply to the class as a whole; not to individual objects. What keyword specifies this?) Then, as each object is created, its constructor can examine this count member variable to determine the appropriate serial number for the new object. Add a member function that permits an object to report its own serial number. Then write a main() program that creates three objects and queries each about its serial number. They should respond I am object number 2, and so on. This question related to Object oriented programming in c++arrow_forwardConsider the following class Certification with following data members Id (int), name (String) , hours(int), level (String), costPerHr (double). You are required to do the following Provide a class StudentCertification that extends Certification . This class has an additional data member rebate (double) o Override the calculateFee method so that the total cost is calculated by getting the total for the training hours @costPerHr and applying the rebate (%). E.g. if the costPerHr is 200 and 50 hours training and 0.2 rebate then the calculateFee should return 10000 – 0.2 *10000 = 8000 Provide another sub class ProfessionalCertification that extends Certification. This class no additional data member. o It overrides the calculateFee method so that the total cost is returned as the product of hours and cost per hour plus 15% tax of the total hours cost. Also the ProfessionalCertification implements the Extendible Interface. You will also have to provide the implementation of method…arrow_forwardThis discussion assignment examines variable scope. For this assignment, we will be examining the Beverage2 class (an updated version of the Beverage class from discussion 1), its subclass Espresso, and the TestBeverage2 class. This time, assume that all source files are in the same package. Note that some lines may wrap, but are still considered on one line. Examine the code below. Recall that variables have four kinds of scope: static, instance, local, and block. For each variable listed below, identify its scope (static, instance, local, or block) and describe the scope using complete sentences. An example description is provided, after the code listing, for the ounces variable in the Beverage2 class. Please describe the scope for the following variables: The num variable in the Beverage2 class The price variable in the Beverage2 class The shots variable in the Espresso class The b variable in the TestBeverage2 class The j variable in the TestBeverage2 class public abstract…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
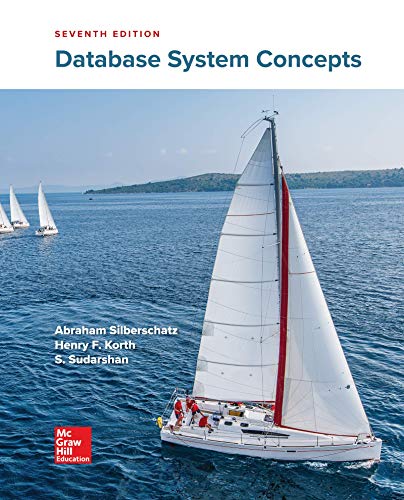
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
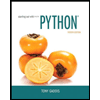
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
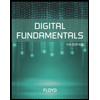
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
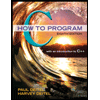
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
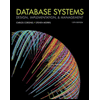
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
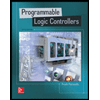
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
6 Stages of UI Design; Author: DesignerUp;https://www.youtube.com/watch?v=_6Tl2_eM0DE;License: Standard Youtube License