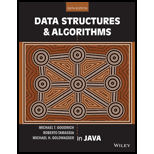
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 2, Problem 23C
Program Plan Intro
Fibonacci series
Program plan:
- Create a main class Progression that prints out the Fibonacci series.
- In main() function,
- Create the object for Progression class.
- Using the object, it prints the value of Fibonacci series starting with 0.
- Declare the default constructor with no parameter.
- Define the parameterized constructor to start the Fibonacci series.
- Define a method nextValue() to return the next value of the progression.
- Define a method advance() that advances the current value to the next value of the progression.
- Finally print the values of progression with spaces using printProgression() method.
- In the FibonacciProgression class,
- Derive the FibonacciProgression class from Progression class.
- Create a default constructor to build up the traditional Fibonacci.
- Define a parameterized constructor to construct a generalized Fibonacci, with the first and second values
- Create a method advance() to replace previous and current values.
- In main() function,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
What are the major threats of using the internet? How do you use it? How do children use it? How canwe secure it? Provide four references with your answer. Two of the refernces can be from an article and the other two from websites.
Assume that a string of name & surname is saved in S. The alphabetical characters in S can be in
lowercase and/or uppercase letters. Name and surname are assumed to be separated by a space
character and the string ends with a full stop "." character.
Write an assembly language program that will copy the name to NAME in lowercase and the surname
to SNAME in uppercase letters. Assume that name and/or surname cannot exceed 20 characters.
The program should be general and work with every possible string with name & surname. However,
you can consider the data segment definition given below in your program.
.DATA
S DB 'Mahmoud Obaid."
NAME DB 20 DUP(?)
SNAME DB 20 DUP(?)
Hint: Uppercase characters are ordered between 'A' (41H) and 'Z' (5AH) and lowercase characters
are ordered between 'a' (61H) and 'z' (7AH) in the in the ASCII Code table. For lowercase letters,
bit 5 (d5) of the ASCII code is 1 where for uppercase letters it is 0. For example,
Letter
'h'
Binary
ASCII
01101000
68H
'H'…
What did you find most interesting or surprising about the scientist Lavoiser?
Chapter 2 Solutions
Data Structures and Algorithms in Java
Ch. 2 - Give three examples of life-critical software...Ch. 2 - Give an example of a software application in which...Ch. 2 - Prob. 3RCh. 2 - Prob. 4RCh. 2 - Prob. 5RCh. 2 - Give a short fragment of Java code that uses the...Ch. 2 - Prob. 7RCh. 2 - Prob. 8RCh. 2 - Prob. 9RCh. 2 - Prob. 10R
Ch. 2 - Prob. 11RCh. 2 - Draw a class inheritance diagram for the following...Ch. 2 - Prob. 13RCh. 2 - Prob. 14RCh. 2 - If the parameter to the makePayment method of the...Ch. 2 - Prob. 16CCh. 2 - Most modern Java compilers have optimizers that...Ch. 2 - The PredatoryCreditCard class provides a...Ch. 2 - Modify the PredatoryCreditCard class so that a...Ch. 2 - Prob. 20CCh. 2 - Write a program that consists of three classes, A,...Ch. 2 - Prob. 22CCh. 2 - Prob. 23CCh. 2 - Write a Java class that extends the Progression...Ch. 2 - Redesign the Progression class to be abstract and...Ch. 2 - Use a solution to Exercise C-2.25 to create a new...Ch. 2 - Use a solution to Exercise C-2.25 to reimplement...Ch. 2 - Write a set of Java classes that can simulate an...Ch. 2 - Write a Java program that inputs a polynomial in...Ch. 2 - Write a Java program that inputs a document and...Ch. 2 - Prob. 31PCh. 2 - Write a Java program that simulates a system that...Ch. 2 - Define a Polygon interface that has methods area()...Ch. 2 - Prob. 35PCh. 2 - Write a Java program that can make change. Your...
Knowledge Booster
Similar questions
- 1. Complete the routing table for R2 as per the table shown below when implementing RIP routing Protocol? (14 marks) 195.2.4.0 130.10.0.0 195.2.4.1 m1 130.10.0.2 mo R2 R3 130.10.0.1 195.2.5.1 195.2.5.0 195.2.5.2 195.2.6.1 195.2.6.0 m2 130.11.0.0 130.11.0.2 205.5.5.0 205.5.5.1 R4 130.11.0.1 205.5.6.1 205.5.6.0arrow_forwardAnalyze the charts and introduce each charts by describing each. Identify the patterns in the given data. And determine how are the data points are related. Refer to the raw data (table):arrow_forward3A) Generate a hash table for the following values: 11, 9, 6, 28, 19, 46, 34, 14. Assume the table size is 9 and the primary hash function is h(k) = k % 9. i) Hash table using quadratic probing ii) Hash table with a secondary hash function of h2(k) = 7- (k%7) 3B) Demonstrate with a suitable example, any three possible ways to remove the keys and yet maintaining the properties of a B-Tree. 3C) Differentiate between Greedy and Dynamic Programming.arrow_forward
- What are the charts (with their title name) that could be use to illustrate the data? Please give picture examples.arrow_forwardA design for a synchronous divide-by-six Gray counter isrequired which meets the following specification.The system has 2 inputs, PAUSE and SKIP:• While PAUSE and SKIP are not asserted (logic 0), thecounter continually loops through the Gray coded binarysequence {0002, 0012, 0112, 0102, 1102, 1112}.• If PAUSE is asserted (logic 1) when the counter is onnumber 0102, it stays here until it becomes unasserted (atwhich point it continues counting as before).• While SKIP is asserted (logic 1), the counter misses outodd numbers, i.e. it loops through the sequence {0002,0112, 1102}.The system has 4 outputs, BIT3, BIT2, BIT1, and WAITING:• BIT3, BIT2, and BIT1 are unconditional outputsrepresenting the current number, where BIT3 is the mostsignificant-bit and BIT1 is the least-significant-bit.• An active-high conditional output WAITING should beasserted (logic 1) whenever the counter is paused at 0102.(a) Draw an ASM chart for a synchronous system to providethe functionality described above.(b)…arrow_forwardS A B D FL I C J E G H T K L Figure 1: Search tree 1. Uninformed search algorithms (6 points) Based on the search tree in Figure 1, provide the trace to find a path from the start node S to a goal node T for the following three uninformed search algorithms. When a node has multiple successors, use the left-to-right convention. a. Depth first search (2 points) b. Breadth first search (2 points) c. Iterative deepening search (2 points)arrow_forward
- We want to get an idea of how many tickets we have and what our issues are. Print the ticket ID number, ticket description, ticket priority, ticket status, and, if the information is available, employee first name assigned to it for our records. Include all tickets regardless of whether they have been assigned to an employee or not. Sort it alphabetically by ticket status, and then numerically by ticket ID, with the lower ticket IDs on top.arrow_forwardFigure 1 shows an ASM chart representing the operation of a controller. Stateassignments for each state are indicated in square brackets for [Q1, Q0].Using the ASM design technique:(a) Produce a State Transition Table from the ASM Chart in Figure 1.(b) Extract minimised Boolean expressions from your state transition tablefor Q1, Q0, DISPATCH and REJECT. Show all your working.(c) Implement your design using AND/OR/NOT logic gates and risingedgetriggered D-type Flip Flops. Your answer should include a circuitschematic.arrow_forwardA controller is required for a home security alarm, providing the followingfunctionality. The alarm does nothing while it is disarmed (‘switched off’). It canbe armed (‘switched on’) by entering a PIN on the keypad. Whenever thealarm is armed, it can be disarmed by entering the PIN on the keypad.If motion is detected while the alarm is armed, the siren should sound AND asingle SMS message sent to the police to notify them. Further motion shouldnot result in more messages being sent. If the siren is sounding, it can only bedisarmed by entering the PIN on the keypad. Once the alarm is disarmed, asingle SMS should be sent to the police to notify them.Two (active-high) input signals are provided to the controller:MOTION: Asserted while motion is detected inside the home.PIN: Asserted for a single clock cycle whenever the PIN has beencorrectly entered on the keypad.The controller must provide two (active-high) outputs:SIREN: The siren sounds while this output is asserted.POLICE: One SMS…arrow_forward
- 4G+ Vo) % 1.1. LTE1 : Q B NIS شوز طبي ۱:۱۷ کا A X حاز هذا على إعجاب Mohamed Bashar. MEDICAL SHOE شوز طبي ممول . اقوى عرض بالعراق بلاش سعر القطعة ١٥ الف سعر القطعتين ٢٥ الف سعر 3 قطع ٣٥ الف القياسات : 40-41-42-43-44- افحص وكدر ثم ادفع خدمة التوصيل 5 الف لكافة محافظات العراق ופרסם BNI SH ופרסם DON JU WORLD DON JU MORISO DON JU إرسال رسالة III Messenger التواصل مع شوز طبي تعليق باسم اواب حمیدarrow_forwardA manipulator is identified by the following table of parameters and variables:a. Obtain the transformation matrices between adjacent coordinate frames and calculate the global transformation matrix.arrow_forwardWhich tool takes the 2 provided input datasets and produces the following output dataset? Input 1: Record First Last Output: 1 Enzo Cordova Record 2 Maggie Freelund Input 2: Record Frist Last MI ? First 1 Enzo Last MI Cordova [Null] 2 Maggie Freelund [Null] 3 Jason Wayans T. 4 Ruby Landry [Null] 1 Jason Wayans T. 5 Devonn Unger [Null] 2 Ruby Landry [Null] 6 Bradley Freelund [Null] 3 Devonn Unger [Null] 4 Bradley Freelund [Null] OA. Append Fields O B. Union OC. Join OD. Find Replace Clear selectionarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
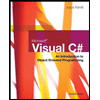
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
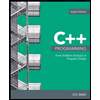
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
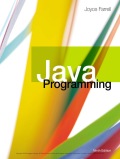
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
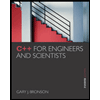
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
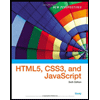
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning