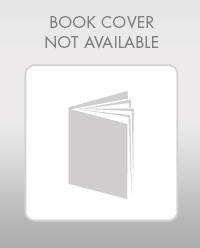
Concept explainers
Write assignment statements that perform the following operations with int variable i, double variables d1 and d2, and char variable c.
A) Subtract 8.5 from d2 and store the result in d1.
B) Divide d1 by 3.14 and store the result in d2.
C) Store the ASCII code for the character 'F' in c.
D) Add 1 to i and store the new value back in i.
E) Add d1 to the current value of d2 and store the result back in d2 as its new value.

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Starting Out With C++: Early Objects (10th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Programming in C
Starting Out With Visual Basic (7th Edition)
Artificial Intelligence: A Modern Approach
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
- (Numerical) Using the srand() and rand() C++ library functions, fill an array of 1000 floating-point numbers with random numbers that have been scaled to the range 1 to 100. Then determine and display the number of random numbers having values between 1 and 50 and the number having values greater than 50. What do you expect the output counts to be?arrow_forwardB- Assume (n, d, m, f, k, e) are variables with integer data type in which n=1, d=m=f=2, e=9. Calculate the value of d, n, k and m for the following statement. Du d++ + --n+(k=e/2);arrow_forward62. State true or false: If I = read(Q) and J = write(Q) then the order of I and J does not matter. a. True b. False c. May be d. Can't sayarrow_forward
- Factorial) The factorial of a nonnegative integer n is written n! (pronounced “n factorial”) and is defined as follows:For example, 5!= 5.4.3.2.1 , which is 120. Use while statements in each of the following:A. Write a program that reads a nonnegative integer and computes and prints its factorial.B. Write a program that estimates the value of the mathematical constant e by using the formula:Prompt the user for the desired accuracy of e (i.e., the number of terms in the summation).C. Write a program that computes the value of by using the formula Prompt the user for the desired accuracy of e (i.e., thenumber of terms in the summation).arrow_forwardQ2) Write a program that reads a one-line sentence as input and then displays the following response: If the sentence ends with a question mark (?) and the input contains an even number of characters, display the word "Yes". If the sentence ends with a question mark and the input contains an odd number of characters, display the word "No". If the sentence ends with an exclamation point (!), display the word "Wow". In all other cases, display the words " you always say "followed by the input string enclosed in quotes" ". Your output should all be on one line. Be sure to note that in the last case, your output must include quotation marks around the echoed input string. In all other cases, there are no quotes in the output. Your program does not have to check the input to see that the user has entered a legitimate sentence. Sample Input#1: Please enter a one line question or statement. How are you? Sample Output#1: Yes Sample Input #2: Please enter a one line…arrow_forwarddef f(x: float) -> int: return int(x) def g(x: str) -> float: return float(x) y = f(g("3.14")) Select all of the following statements that are true: y's inferred data type is float O y 's inferred data type is str O y's inferred data type is int There is a type error in this program None of the above are truearrow_forward
- Write code that performs the following input operations: Read an int from the keyboard and assign it to a variable named k . (Do not print a prompt. Use the input() function without a prompt-string to read the input.) Read a float from the keyboard and assign it to a variable named d . (Do not print a prompt. Use the input() function without a prompt-string to read the input.) Read a string from the keyboard and assign it to a variable named s . (Do not print a prompt. Use the input() function without a prompt-string to read the input.) After you have performed the input, on one line, print these variables in reverse order ( s followed by d , followed by k ) with exactly one space in between each. On a second line, print them in the original order ( k followed by d , followed by s ) with one space in between them.arrow_forward61. State true or false: If I = read(Q) and J = read(Q) then the order of I and J does not matter. a. True b. False c. May be d. Can't sayarrow_forwardDeclare int variables x and y. Initialize x to 25 and y to 18.arrow_forward
- 1- Write a program that converts inches to centimeters. a) Prompt the user to enter a value in inches. I b) Write a set of statements that convert and store the value of inch to cm. The conversion factor is 2.54 cm/inch. c) Write statement that displays the results like below: 17.45 inches = 44.32 centimeters 2- Prompt the user for their first name initial. Then convert their initial to the ASCII code and display it as their secret code!arrow_forwarddo itarrow_forwardCODE FOR SINGLE-DIGIT CALCULATOR USING EMU8086 Write a program that would accept 2 single-digit numbers num1 and num2 (0-9) and an arithmetic operator (+, - , * and /). Perform arithmetic operations entered with num1 and num2. If the operator is invalid, display "Operation Error".arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
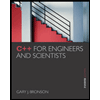
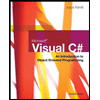