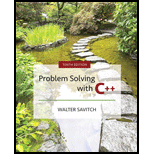
Concept explainers
A metric ton is 35,273.92 ounces. Write a

Weight of breakfast package
Program Plan:
- Include required header files.
- Initializes “const” variable “OUNCES_PER_METRICTON” to “35273.92”.
- Define main function,
- Declare three variables in “double” datatype.
- Declare a “char” variable for user option.
- Then performs “do-while” loop.
- Prompt statement for read the weight of packages in ounces.
- Read the weight of packages from user.
- Compute the weight in metric tons using “ounces/OUNCES_PER_METRICTON” and then store it in a variable “weightMetricTon”.
- Compute the number of boxes required to produce “1” metric ton using “OUNCES_PER_METRICTON/ounces” and then store it in a variable “no_of_boxes”.
- Display the weight in metric tons and number of boxes.
- Prompt statement for read the user option to continue the calculation.
- Read the user option.
- Check the user option using “while” loop. If the user entered option is equal to “y” or “Y” then continue the above process.
- Otherwise, the program terminated.
The below program is used to compute the weight of package of breakfast cereal in metric tons and number of boxes from the given weight of package in ounces
Explanation of Solution
Program:
//Header file
#include <iostream>
//For standard input and output
using namespace std;
/* Initializes the const variable "OUNCES_PER_METRICTON" to "35273.92" */
const double OUNCES_PER_METRICTON = 35273.92;
//Define main function
int main()
{
//Declare variables in type of "double"
double ounces, weightMetricTon, no_of_boxes;
//Declare variable in type of "char"
char option;
//Check the condition using "do-while" loop
do
{
/* Prompt statement for read the weight of package in ounces */
cout << "Enter the weight of package of breakfast cereal in ounces: ";
//Read the weight in ounces from user
cin>>ounces;
//Compute the given weight in metric ton
weightMetricTon = ounces/OUNCES_PER_METRICTON;
/* Compute the number of boxes required to produce "1" metric ton */
no_of_boxes = OUNCES_PER_METRICTON/ounces;
//Display the weight in metric tons
cout << "Weight of packets in metric tons: " << weightMetricTon << endl;
//Display the number of boxes
cout << "Number of boxes needed to yield 1 metric ton of cereal: " << no_of_boxes << endl;
//Prompt statement for asking the user choice
cout << "Enter 'y' or 'Y' to continue (OR) Enter any character to terminate: ";
//Read option from user
cin >> option;
}
/* If the user entered option is "y" or "Y", then continue the above process. Otherwise program terminated */
while (option == 'y' || option == 'Y');
return 0;
}
Output:
Enter the weight of package of breakfast cereal in ounces: 4000
Weight of packets in metric tons: 0.113398
Number of boxes needed to yield 1 metric ton of cereal: 8.81848
Enter 'y' or 'Y' to continue (OR) Enter any character to terminate: y
Enter the weight of package of breakfast cereal in ounces: 4820
Weight of packets in metric tons: 0.136645
Number of boxes needed to yield 1 metric ton of cereal: 7.31824
Enter 'y' or 'Y' to continue (OR) Enter any character to terminate: n
Want to see more full solutions like this?
Chapter 2 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Database Concepts (8th Edition)
Degarmo's Materials And Processes In Manufacturing
Starting Out with C++ from Control Structures to Objects (9th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
- Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forwardQuestion: Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forward23:12 Chegg content://org.teleg + 5G 5G 80% New question A feed of 60 mol% methanol in water at 1 atm is to be separated by dislation into a liquid distilate containing 98 mol% methanol and a bottom containing 96 mol% water. Enthalpy and equilibrium data for the mixture at 1 atm are given in Table Q2 below. Ask an expert (a) Devise a procedure, using the enthalpy-concentration diagram, to determine the minimum number of equilibrium trays for the condition of total reflux and the required separation. Show individual equilibrium trays using the the lines. Comment on why the value is Independent of the food condition. Recent My stuff Mol% MeOH, Saturated vapour Table Q2 Methanol-water vapour liquid equilibrium and enthalpy data for 1 atm Enthalpy above C˚C Equilibrium dala Mol% MeOH in Saturated liquid TC kJ mol T. "Chk kot) Liquid T, "C 0.0 100.0 48.195 100.0 7.536 0.0 0.0 100.0 5.0 90.9 47,730 928 7,141 2.0 13.4 96.4 Perks 10.0 97.7 47,311 87.7 8,862 4.0 23.0 93.5 16.0 96.2 46,892 84.4…arrow_forward
- You are working with a database table that contains customer data. The table includes columns about customer location such as city, state, and country. You want to retrieve the first 3 letters of each country name. You decide to use the SUBSTR function to retrieve the first 3 letters of each country name, and use the AS command to store the result in a new column called new_country. You write the SQL query below. Add a statement to your SQL query that will retrieve the first 3 letters of each country name and store the result in a new column as new_country.arrow_forwardWe are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7). A code breaker manages to factories 247 = 13 x 19 Determine Alice's secret key. To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.arrow_forwardConsider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.arrow_forward
- Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forward4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
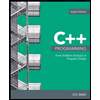
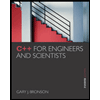
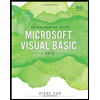
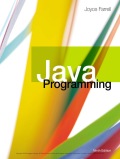
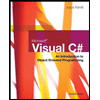