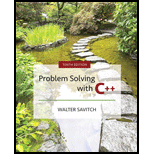
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 2.3, Problem 16STE
Explanation of Solution
Program:
//Header file
#include <iostream>
//For standard input and output
using namespace std;
//Main function
int main()
{
//Assign the variables "a" to character 'b'
char a = 'b';
// Assign the variables "b" to character 'c'
char b = 'c';
//Set a variables "a" to "c"
char c = a;
//Display the given result
cout << a << b << c << 'c';
return 0;
}
Explanation:
The given code is used to display the character in the given initialization.
- Define main function.
- First, assign the “char” variable “a” to character ‘b’.
- Assign the “char” variable “b” to character ‘c’...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Show all the work
Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.
Show all the work
Chapter 2 Solutions
Problem Solving with C++ (10th Edition)
Ch. 2.1 - Give the declaration for two variables called feet...Ch. 2.1 - Give the declaration for two variables called...Ch. 2.1 - Give a C++ statement that will change the value of...Ch. 2.1 - Give a C++ statement that will increase the value...Ch. 2.1 - Give a C++ statement that will change the value of...Ch. 2.1 - Prob. 6STECh. 2.1 - Prob. 7STECh. 2.2 - Give an output statement that will produce the...Ch. 2.2 - Give an input statement that will fill the...Ch. 2.2 - Prob. 10STE
Ch. 2.2 - Write a complete C++ program that writes the...Ch. 2.2 - Write a complete C++ program that reads in two...Ch. 2.2 - Prob. 13STECh. 2.2 - Write a short program that declares and...Ch. 2.3 - Convert each of the following mathematical...Ch. 2.3 - Prob. 16STECh. 2.3 - What is the output of the following program lines...Ch. 2.3 - Write a complete C++ program that reads two whole...Ch. 2.3 - Given the following fragment that purports to...Ch. 2.3 - What is the output of the following program lines...Ch. 2.4 - Write an if-else statement that outputs the word...Ch. 2.4 - Suppose savings and expenses are variables of type...Ch. 2.4 - Write an if-else statement that outputs the word...Ch. 2.4 - Write an if-else statement that outputs the word...Ch. 2.4 - Consider a quadratic expression, say x2 x 2...Ch. 2.4 - Consider the quadratic expression x2 4x + 3...Ch. 2.4 - What is the output of the following cout...Ch. 2.4 - What is the output produced by the following (when...Ch. 2.4 - What output would be produced in the previous...Ch. 2.4 - What is the output produced by the following (when...Ch. 2.4 - What is the output produced by the following (when...Ch. 2.4 - What is the most important difference between a...Ch. 2.4 - What is the output produced by the following (when...Ch. 2.4 - Write a complete C++ program that outputs the...Ch. 2.5 - The following if-else statement will compile and...Ch. 2.5 - Prob. 36STECh. 2.5 - Write a complete C++ program that asks the user...Ch. 2 - A metric ton is 35,273.92 ounces. Write a program...Ch. 2 - The Babylonian algorithm to compute the square...Ch. 2 - Many treadmills output the speed of the treadmill...Ch. 2 - Write a program that plays the game of Mad Lib....Ch. 2 - The following is a short program that computes the...Ch. 2 - A government research lab has concluded that an...Ch. 2 - Workers at a particular company have won a 7.6%...Ch. 2 - Modify your program from Programming Project 2 so...Ch. 2 - Negotiating a consumer loan is not always...Ch. 2 - Write a program that determines whether a meeting...Ch. 2 - Prob. 6PPCh. 2 - It is difficult to make a budget that spans...Ch. 2 - You have just purchased a stereo system that cost...Ch. 2 - Write a program that reads in ten whole numbers...Ch. 2 - Modify your program from Programming Project 9 so...Ch. 2 - Sound travels through air as a result of...Ch. 2 - Prob. 12PPCh. 2 - The HarrisBenedict equation estimates the number...Ch. 2 - Write a program that calculates the total grade...Ch. 2 - It is important to consider the effect of thermal...Ch. 2 - Prob. 16PP
Knowledge Booster
Similar questions
- Show all the workarrow_forwardShow all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forward
- Question 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forwardGenerate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forward
- I need help to solve the following, thank youarrow_forwardreminder it an exercice not a grading work GETTING STARTED Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website. Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”. If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically. With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet. If cell B6 does not display your name, delete the file and download a new copy from the SAM website. Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…arrow_forwardNeed help completing this algorithm here in coding! 2arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
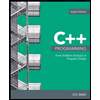
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
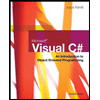
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
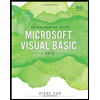
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
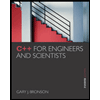
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
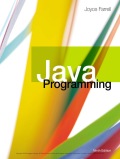
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT