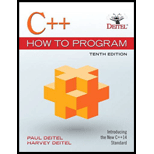
C++ How to Program (Early Objects Version)
10th Edition
ISBN: 9780134448824
Author: Paul Deitel; Harvey M. Deitel
Publisher: Pearson Education (US)
expand_more
expand_more
format_list_bulleted
Want to see more full solutions like this?
Subscribe now to access step-by-step solutions to millions of textbook problems written by subject matter experts!
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Topic: Singly Linked ListImplement the following functions in C++ program. Read the question carefully. Below are the "CODE THAT NEEDS TO BE IMPROVED" or "NOT FINAL CODE" (See attached photo for reference)
int add(int num)
This will add the element num into the linked list and return the position of the newly-added element. In the above example, having add(60) will return 5 as it is the fifth position in the list.
int remove(int num)
This will remove the first instance of the element and return the position of the removed element. In the above example, having remove(40) will return 3 as 40 was the third element in the linked list before having removed.
int get(int pos)
This method will get the element at the specified position. This will return -1 if the specified position is invalid.
int add(int num) {
addTail(num);
return index;
}
int remove(int num) {
node* currnode = head;
node* prevnode = NULL;…
TOPIC: Singly Linked ListImplement the following functions using C++
int add(int num)
This will add the element num into the linked list and return the position of the newly-added element. In the above example, having add(60) will return 5 as it is the fifth position in the list.
int remove(int num)
This will remove the first instance of the element and return the position of the removed element. In the above example, having remove(40) will return 3 as 40 was the third element in the linked list before having removed.
int get(int pos)
This method will get the element at the specified position. This will return -1 if the specified position is invalid.
bool addAt(int num, int pos)
This method will add the integer num to the posth position of the list and returns true if the value of pos is valid.
Performing addAt(20, 2) in the example list will add 20 at the 2nd position and the linked list will now look like this: 10 -> 20 -> 30 -> 40 -> 50.
When the value of pos is…
Topic: Singly Linked ListImplement the following functions in C++ program. Read the question carefully. Below is the "CODE THAT NEEDS TO BE IMPROVED" or "NOT FINAL CODE" (See attached photo for reference)
bool addAt(int num, int pos)
This method will add the integer num to the posth position of the list and returns true if the value of pos is valid.
Performing addAt(20, 2) in the example list will add 20 at the 2nd position and the linked list will now look like this: 10 -> 20 -> 30 -> 40 -> 50.
When the value of pos is invalid i.e. greater than the size + 1 or less than one, return false.
bool addAt(int num, int pos) {
if (pos == 1) { // case 1: addHead
addHead(num);
return true;
}
if (pos == index + 1) { // case 3: addTail
addTail(num);
return true;
}
node* currnode = head; //addAt(20, 3)
int count = 0;
while (currnode…
Chapter 19 Solutions
C++ How to Program (Early Objects Version)
Ch. 19 - Prob. 19.6ECh. 19 - Prob. 19.7ECh. 19 - Prob. 19.8ECh. 19 - Prob. 19.9ECh. 19 - Prob. 19.10ECh. 19 - Prob. 19.11ECh. 19 - (Infix-to-Post fix conversion) Stacks are used by...Ch. 19 - Prob. 19.13ECh. 19 - Prob. 19.14ECh. 19 - Prob. 19.15E
Ch. 19 - Prob. 19.16ECh. 19 - Prob. 19.17ECh. 19 - (Duplicate Elimination) In this chapter, we saw...Ch. 19 - Prob. 19.19ECh. 19 - Prob. 19.20ECh. 19 - Prob. 19.21ECh. 19 - Prob. 19.22ECh. 19 - Prob. 19.23ECh. 19 - Prob. 19.24ECh. 19 - Prob. 19.25ECh. 19 - Prob. 19.26ECh. 19 - Prob. 19.27ECh. 19 - Prob. 19.28E
Knowledge Booster
Similar questions
- ( JAVA LANGUAGE ) Create and print a linked list with four (4) elements. Ice cream flavors will be the basis of the required values for the linked listarrow_forwardscheme: Q9: Sub All Write sub-all, which takes a list s, a list of old words, and a list of new words; the last two lists must be the same length. It returns a list with the elements of s, but with each word that occurs in the second argument replaced by the corresponding word of the third argument. You may use substitute in your solution. Assume that olds and news have no elements in common. (define (sub-all s olds news) 'YOUR-CODE-HERE )arrow_forward(Q5) This is a Data Structures problem and the programming language used is Lisp. Solve the question we detailed steps and make it concise and easy to understand. Please and thank you.arrow_forward
- (Don't use C++) Only use Carrow_forwardC++ Programming. Theme: Standard string manipulation functions - string concatenation, comparison, character search, string search, replacement and deletion. Task : Given a line(string) of type String . Write a program that arranges its elements in an array of type char A in alphabetical order and in an array of type byte B in ascending order.arrow_forward(Difficulty Level 3)Write a function frequency_match(text, chars) that receives a string text and a dictionary chars that has characters as keys and numbers as values. The function must return a list with all the characters that occur in the text as many times as their associated value in the char dictionary. Not all the chars in the text will be present in the dictionary, but you may assume that all the chars in the dictionary are in the text. You are allowed to change the values in the dictionary. solve using pythonarrow_forward
- [ ] [] power In the cell below, you are to write a function "power(list)" that takes in a list as its input, and then returns the power set of that list. You may assume that the input will not have any duplicates (i.e., it's a set). After compiling the above cell, you should be able to compile the following cell and obtain the desired outputs. print (power ( ["A", "B"]), power ( ["A", "B", "C"])) + Code + Markdown This should return [[], ["A"], ["B"], ["A", "B"]] [[], ["A"], ["B"], ["C"], ["A", "B"], ["A", "C"], ["B", "C"], ["A", "B", "C"]] (the order in which the 'sets' appear does not matter, just that they are all there) Python Pythonarrow_forward(True/False): Arrays are passed by reference to avoid copying them onto the stackarrow_forward16-Write a function called find_integer_with_most_divisors that receives a list of integers and returns the integer from the list with the most divisors .In the event of a tie, the first case with the most divisors returns .For example: If the list looks like this: [8, 12, 18, 6] In this list, the number 8 has four divisors, which are ;[1,2,4,8] :The number 12 has six divisors, which are ;[1,2,3,4,6,12] :The number 18 has six divisors, which are ;[1,2,3,6,9,18] :And the number 6 has four divisors, which are : [1,2,3,6]Note that both items 12 and 18 have the maximum number of divisors and are equal in number of divisors (both have 6 divisors) .Your function must return the first item with the maximum number of divisors ;Therefore it must return the following value: 12arrow_forward
- PYTHON Problem Statement Given a list of numbers (nums), for each element in nums, calculate how many numbers in the list are smaller than it. Write a function that does the calculation and returns the result (as a list). For example, if you are given [6,5,4,8], your function should return [2, 1, 0, 3] because there are two numbers less than 6, one number less than 5, zero numbers less than 4, and three numbers less than 8. Sample Input smaller_than_current([6,5,4,8]) Sample Output [2, 1, 0, 3]arrow_forward(Q6) This is a Data Structures problem and the programming language used is Lisp. Solve the question we detailed steps and make it concise and easy to understand. Please and thank you. Can you please explain this question specifically what you are doing in each step because it is a very confusing topic. Thanks!arrow_forward(Online Address Book revisited) Programming Exercise 5 in Chapter 11 could handle a maximum of only 500 entries. Using linked lists, redo the program to handle as many entries as required. Add the following operations to your program: Add or delete a new entry to the address book. Allow the user to save the data in the address book.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
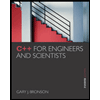
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr