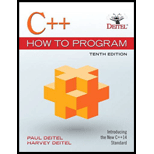
C++ How to Program (Early Objects Version)
10th Edition
ISBN: 9780134448824
Author: Paul Deitel; Harvey M. Deitel
Publisher: Pearson Education (US)
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 19.21E
Program Plan Intro
Program Plan:
This Program defines the following structure to represent a List Node in Linked List
structlist _node {
intdata ;
structlist _node *next; // pointer to next node in the list
}node;
To allow searching an element in a list following method is defined.
node * searchNode (node *head, int data);
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Topic: Singly Linked ListImplement the following functions in C++ program. Read the question carefully. Below are the "CODE THAT NEEDS TO BE IMPROVED" or "NOT FINAL CODE" (See attached photo for reference)
int add(int num)
This will add the element num into the linked list and return the position of the newly-added element. In the above example, having add(60) will return 5 as it is the fifth position in the list.
int remove(int num)
This will remove the first instance of the element and return the position of the removed element. In the above example, having remove(40) will return 3 as 40 was the third element in the linked list before having removed.
int get(int pos)
This method will get the element at the specified position. This will return -1 if the specified position is invalid.
int add(int num) {
addTail(num);
return index;
}
int remove(int num) {
node* currnode = head;
node* prevnode = NULL;…
C++ PROGRAMMING
(Linked list) complete the functions:
You have to continue on implementing your Array List namely the following functions:
Example ArrayList: [10, 30, 40, 50]
void addAt(int num, int pos)
This method will add the integer num to the posth position of the list.
Performing addAt(20, 2) in the example list will add 20 at the 2nd position and the array will now look like this: [10, 20, 30, 40, 50]
When the value of pos is greater than the size + 1 or less than one, output "Position value invalid"
void removeAt(int pos)
Removes the number in the posth position of the list.
Performing removeAt(3) in the example list will remove the 3rd element of the list and the updated array will be: [10, 30, 50]
When the value of pos is greater than the size or less than one, output "Position value invalid"
My incomplete code:
#include <cstdlib>#include <iostream>using namespace std;
class ArrayList : public List { // : means "is-a" / extend int* array; int index;…
Question Info:
The calculation "a" was used for loop to calculate the sum of squares of values in this list: 1, 7, 8, 6, 11 (The answer was 271)
Question To Answer:
(1) Create recursive function to do the same calculation a in the above. (The function input will be the list. Each recursion, you are going to send a sub-list with one less item from the list)
I need help doing this in python programming language.
Chapter 19 Solutions
C++ How to Program (Early Objects Version)
Ch. 19 - Prob. 19.6ECh. 19 - Prob. 19.7ECh. 19 - Prob. 19.8ECh. 19 - Prob. 19.9ECh. 19 - Prob. 19.10ECh. 19 - Prob. 19.11ECh. 19 - (Infix-to-Post fix conversion) Stacks are used by...Ch. 19 - Prob. 19.13ECh. 19 - Prob. 19.14ECh. 19 - Prob. 19.15E
Ch. 19 - Prob. 19.16ECh. 19 - Prob. 19.17ECh. 19 - (Duplicate Elimination) In this chapter, we saw...Ch. 19 - Prob. 19.19ECh. 19 - Prob. 19.20ECh. 19 - Prob. 19.21ECh. 19 - Prob. 19.22ECh. 19 - Prob. 19.23ECh. 19 - Prob. 19.24ECh. 19 - Prob. 19.25ECh. 19 - Prob. 19.26ECh. 19 - Prob. 19.27ECh. 19 - Prob. 19.28E
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- [ ] [] power In the cell below, you are to write a function "power(list)" that takes in a list as its input, and then returns the power set of that list. You may assume that the input will not have any duplicates (i.e., it's a set). After compiling the above cell, you should be able to compile the following cell and obtain the desired outputs. print (power ( ["A", "B"]), power ( ["A", "B", "C"])) + Code + Markdown This should return [[], ["A"], ["B"], ["A", "B"]] [[], ["A"], ["B"], ["C"], ["A", "B"], ["A", "C"], ["B", "C"], ["A", "B", "C"]] (the order in which the 'sets' appear does not matter, just that they are all there) Python Pythonarrow_forwardInformation to solve question: Use "a" for loop to calculate the sum of squares of values in this list: 1, 7, 8, 6, 11. (The answer should be 271) Create a recursive function to do the same calculation a in the previous question. (The function input will be the list. Each recursion, you are going to send a sub-list with one less item from the list) ----------------------------------------------------------------------------------- (1) Create a function to perform bubble sorting in python? The algorithm should stop when there are no swaps in the last iteration.arrow_forwardQuestion > Not complete Marked out of 1.50 Flag question Previous page Write a recursive function named get_palindromes (words) that takes a list of words as a parameter. The function should return a list of all the palindromes in the list. The function returns an empty list if there are no palindromes in the list or if the list is empty. For example, if the list is ["racecar", "hello", "noon", "goodbye"], the function should return ["racecar", "noon"]. A palindrome is a word that is spelled the same forwards and backwards. Note: The get_palindromes() function has to be recursive; you are not allowed to use loops to solve this problem. For example: Test words = ["racecar", "hello", "noon", "goodbye", "test", 'aibohphobia'] ['racecar', 'noon', 'aibohphobia'] print (get_palindromes (words)) print (get_palindromes ([])) print (get_palindromes (['this', 'is', 'test'])) Answer: (penalty regime: 0, 0, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 %) Result Precheck Check [] [] Next pagearrow_forward
- [] [] partite_sets In the cell below, you are to write a function "partite_sets (graph)" that takes in a BIPARTITE graph as its input, and then returns a single list whose two entries are the partite sets of the graph as lists (the order of the sets outputed does not matter). After compiling the above cell, you should be able to compile the following cell and obtain the desired outputs. print (partite_sets({"A" : ["B", "C"], "B" : partite_sets({"A" : ["B", "C"], "B" : ["A"], "C" : ["A"]}), ["A", "D"], "C" : ["A", "D"], "D" : ["B", "C"]})) This should return [["B","C"], ["A"]] [["A","D"], ["B","C"]] (the order in which the entries appear does not matter) Python Pythonarrow_forward(GREATEST COMMON DIVISOR) The greatest common divisor of integers x and y is the largest integer that evenly divides into both x and y. Write and test a recursive function gcd that returns the greatest common divisor of x and y. The gcd of x and y is defined recursively as follows: If y is equal to 0, then gcd (x, y) is x; otherwise, gcd (x, y) is gcd (y, x % y), where % is the remainder operator.arrow_forward16-Write a function called find_integer_with_most_divisors that receives a list of integers and returns the integer from the list with the most divisors .In the event of a tie, the first case with the most divisors returns .For example: If the list looks like this: [8, 12, 18, 6] In this list, the number 8 has four divisors, which are ;[1,2,4,8] :The number 12 has six divisors, which are ;[1,2,3,4,6,12] :The number 18 has six divisors, which are ;[1,2,3,6,9,18] :And the number 6 has four divisors, which are : [1,2,3,6]Note that both items 12 and 18 have the maximum number of divisors and are equal in number of divisors (both have 6 divisors) .Your function must return the first item with the maximum number of divisors ;Therefore it must return the following value: 12arrow_forward
- Complete my C++ program: Instructions: You have to continue on implementing your Array List namely the following functions: Example ArrayList: [10, 30, 40, 50] void addAt(int num, int pos) This method will add the integer num to the posth position of the list. Performing addAt(20, 2) in the example list will add 20 at the 2nd position and the array will now look like this: [10, 20, 30, 40, 50] When the value of pos is greater than the size + 1 or less than one, output "Position value invalid" void removeAt(int pos) Removes the number in the posth position of the list. Performing removeAt(3) in the example list will remove the 3rd element of the list and the updated array will be: [10, 30, 50] When the value of pos is greater than the size or less than one, output "Position value invalid" void removeAll(int num) Removes all instances of num in the array list. In this array [10, 10, 20, 30, 10], performing removeAll(10) will remove all 10's and the list will look like this: [20,…arrow_forwardCalculation "a" was used for loop to calculate the sum of squares of values in this list: 1, 7, 8, 6, 11 (The answer was 271) (Question 1) Create recursive function to do the same calculation "a" in the above. (The function input will be the list. Each recursion, you are going to send a sub-list with one less item from the list) (Hint:There are many ways to do sorting. Create a function to perform bubble sorting as descried in this tutorial: (https://www.geeksforgeeks.org/bubble-sort/) . The algorithm should stop when there are no swaps in the last iteration.)arrow_forward(True/False): Local variables are created by adding a positive value to the stack pointerarrow_forward
- Psecode code explaination of the problem C++arrow_forward(Recursive Greatest Common Divisor) The greatest common divisor of integers x and y isthe largest integer that evenly divides both x and y. Write a recursive function gcd that returns thegreatest common divisor of x and y. The gcd of x and y is defined recursively as follows: If y is equalto 0, then gcd(x, y) is x; otherwise gcd(x, y) is gcd(y, x % y), where % is the remainder operator.arrow_forward(Q6) This is a Data Structures problem and the programming language used is Lisp. Solve the question we detailed steps and make it concise and easy to understand. Please and thank you. Can you please explain this question specifically what you are doing in each step because it is a very confusing topic. Thanks!arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
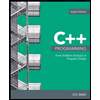
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning