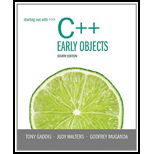
Starting Out with C++: Early Objects
8th Edition
ISBN: 9780133360929
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Question
Chapter 19, Problem 12RQE
Program Plan Intro
Binary tree:
- Binary tree is a non-linear data structure.
- Binary tree contains node such as root node that is pointed to two child nodes.
- A root node will have left reference node and right reference node.
- Binary tree will contain more than one self-referenced field.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
In Python
Implement a binary search tree and write a function to determine if it is a valid binary search tree.
Create a binary linked tree, and traverse the tree by using the recursive function.
The structure of the tree is as follow:
You should input the nodes in pre-order sequence. If a child of a node is NULL, input a space.
Write the function of create binary tree, pre-order to print the nodes, in-order to print the nodes and post-order to print the nodes.
Count the height of the tree.
Header file
typedef char ElemType;
typedef struct node//define the type of binary tree node
{
}BTnode;
Source file
#include <stdio.h>
#include <stdlib.h>
#include "tree.h"
BTnode * createTree()//create the binary tree,return the root
{
BTnode *tnode;// tnode is the root
char elem;
;//input the character
//if the input is a space,set the pointer as NULL
Else// if the input is not a space,generate the binary node and create its left sub-tree and right…
Chapter 19 Solutions
Starting Out with C++: Early Objects
Ch. 19.1 - Prob. 19.1CPCh. 19.1 - Prob. 19.2CPCh. 19.1 - Prob. 19.3CPCh. 19.1 - Prob. 19.4CPCh. 19.1 - Prob. 19.5CPCh. 19.1 - Prob. 19.6CPCh. 19.2 - Prob. 19.7CPCh. 19.2 - Prob. 19.8CPCh. 19.2 - Prob. 19.9CPCh. 19.2 - Prob. 19.10CP
Ch. 19.2 - Prob. 19.11CPCh. 19.2 - Prob. 19.12CPCh. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - Prob. 3RQECh. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - Prob. 6RQECh. 19 - Prob. 7RQECh. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - Prob. 17RQECh. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Prob. 6PCCh. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PC
Knowledge Booster
Similar questions
- 1. Give a recursive algorithm in pseudocode to compute the diameter of a binary tree. Recall that diameter is defined as the number of nodes on the longest path between any two nodes in the tree. Nodes have left and right references, but nothing else. You must use the height function, defined as follows, in your solution. Your solution will return the diameter of the tree as an integer. function height (Node n) 1. if n = null 2. return -1 3. return 1 + max (height (n.left), height (n.right)) Write your solution below. function diameter (Node n)arrow_forwardConstruct a Binary Search Tree (BST) using the data provided in shaded row and name it “BST-1”. 50 45 65 60 75 90 15 35 70 45 55 40 20 80 95 85 25 50 74 5 Provide answers to the following considering your constructed BST-1: Write a C++ code for the in-order traversal of BST-1. Also, dry run your code on it and elaborate its each step. Compute the total number of used and unused (null) references defined in BST-1. And construct an equivalent two-way in-order threaded BST-1 that has minimum number of null references.arrow_forwardGiven a reference to a binary tree t. I am trying to write a python function using recursion that will check if an integer called k is stored in a leaf node in the tree. if not stored it should return false but if it is I want it to return True. starting function: def in_leaf(t,k):arrow_forward
- Given a number and a sorted binary tree, write function that inserts the number into the tree. Given a list of numbers, write a function that inserts each number into the tree. Given a sorted tree, write a function that creates a sorted list.arrow_forwardCreate a binary linked tree, and traverse the tree by using the recursive function. The structure of the tree is as follows: //check pic// You should input the nodes in pre-order sequence. If a child of a node is NULL, input a space. Write the function of create binary tree, pre-order to print the nodes, in-order to print the nodes and post-order to print the nodes. Count the height of the tree. Hints: Header file typedef char ElemType; typedef struct node//define the type of binary tree node { }BTnode; Source file #include <stdio.h> #include <stdlib.h> #include "tree.h" BTnode * createTree()//create the binary tree,return the root { BTnode *tnode;// tnode is the root char elem; ;//input the character //if the input is a space,set the pointer as NULL Else// if the input is not a space,generate the binary node and create its left…arrow_forward4. a) The preorder traversal of a Binary Search Tree (BST) is given below. 75 55 45 50 60 65 95 85 110 100 Draw the BST and briefly explain the process you followed to build the tree. b) Write a function f1 that takes the root of a binary tree as a parameter and returns the sum of the nodes, which are the right child of another node. The root of the tree is not a child of any node. Consider the following class definitions while writing your code. class Node { public: int key; Node* left; Node* right; } ;arrow_forward
- 4. Write a recursive algorithm in pseudocode that finds the lowest common ancestor (LCA) of two given nodes in a binary tree T. The lowest common ancestor is defined between two nodes p and q as the lowest node in T that has both p and q as descendants (where we allow a node to be a descendant of itself). If either p or q is null, the LCA is null. For this problem, Nodes have left, right, and parent references as well as a field called level which stores the level of the node in the tree. In the sample tree below, node 5 is on level 0, while nodes 4 and 6 are on level 1. Write your solution here. 5 <- level 0 4 6 < level 1 function lowest_common_ancestor (Node P, Node q)arrow_forwardPLEASE PROVIDE ME THE CODE IN C LANGUAGE.arrow_forwardCreate a function that checks whether a binary tree is balanced. For the sake of this issue, a balanced tree is one in which the heights of the two subtrees of each node never deviate by more than one.arrow_forward
- Build a Binary Search Tree with the given input order. You must show step by step process of inserting the inputs including both recursive calls and tree diagrams for each step. [ refer to the Insertitem function of Lecture 10 slides to get an idea of doing this.] N.B: Unique Input orders for each student to build the tree are given on a separate file. Write down the input order on your answer script before answering the question. You must use the assigned input order. Do not change the order. TREE INPUT: 4,1,9,3,2,5,6,8,7arrow_forwardb. Given a BST whose root node is represented by the node root. Write a function findroots(Node * root) to traverse the BST and print the number of total parent (atleast have a child node) nodes of the BST. N.B: Print order doesn't matter Fig 1: An example Binary Search Tree. 5 For example: In this figure, there are total 4 parentsarrow_forwardJava code neededarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
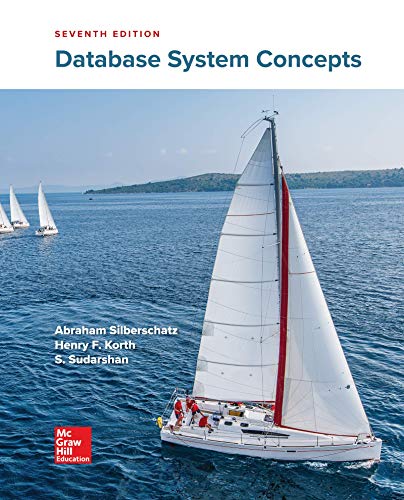
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
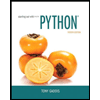
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
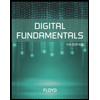
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
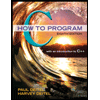
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
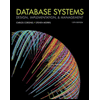
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
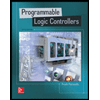
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education