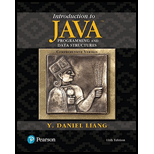
(Occurrences of a specified character in a string) Write a recursive method that finds the number of occurrences of a specified letter in a string using the following method header:
public static int count(String str, char a)
For example, count(“Welcome”, ‘e’) returns 2. Write a test

Want to see the full answer?
Check out a sample textbook solution
Chapter 18 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Objects (6th Edition)
Programming in C
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Starting out with Visual C# (4th Edition)
Starting Out with Python (4th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- public static String pancakeScramble(String text) This nifty little problem is taken from the excellent Wolfram Challenges problem site where you can also see examples of what the result should be for various arguments. Given a text string, construct a new string by reversing its first two characters, then reversing the first three characters of that, and so on, until the last round where you reverse your entire current string.This problem is an exercise in Java string manipulation. For some mysterious reason, the Java String type does not come with a reverse method. The canonical way to reverse a Java string str is to first convert it to mutable StringBuilder, reverse its contents, and convert the result back to an immutable string, that is,str = new StringBuilder(str).reverse().toString(); Here's the tester it must pass: @Test public void testPancakeScramble() throws IOException {// Explicit test casesassertEquals("", P2J3.pancakeScramble(""));assertEquals("alu",…arrow_forwardData Structures the hasBalancedParentheses () method. Note: this can be done both iteratively or recursively, but I believe most people will find the iterative version much easier. C++: We consider the empty string to have balanced parentheses, as there is no imbalance. Your program should accept as input a single string containing only the characters ) and (, and output a single line stating true or false. The functionality for reading and printing answers is written in the file parentheses.checker.cpp; your task is to complete the has balanced.parentheses () function. **Hint: There's a pattern for how to determine if parentheses are balanced. Try to see if you can find that pattern first before coding this method up.arrow_forward# Data conversion with format method(PYTHON) 1- As discussed in the chapter string formatting could be used to simplify the dataconvert2.py Redo this program making use of the string-formatting method. Please explain your answer I’m really confused??arrow_forward
- CodeWorkout Gym Course Search exercises... Q Search kola shreya@columbus X275: Recursion Programming Exercise: Check Palindrome X275: Recursion Programming Exercise: Check Palindrome Write a recursive function named checkPalindrome that takes a string as input, and returns true if the string is a palindrome and false if it is not a palindrome. A string is a palindrome if it reads the same forwards or backwards. Recall that str.charAt(a) will return the character at position a in str. str.substring(a) will return the substring of str from position a to the end of str,while str.substring(a, b) will return the substring of str starting at position a and continuing to (but not including) the character at position b. Examples: checkPalindrome ("madam") -> true Your Answer: 1 public boolean checkPalindrome (String s) { 4 CodeWorkout © Virginia Tech About License Privacy Contactarrow_forward7bact1 Please help me answer this in python programming.arrow_forward2..arrow_forward
- Consider the following pseudo code, Method func() { PRINT “This is recursive function" func() } Method main( { func() } What will happen when the above snippet is executed?arrow_forwardWrite the countVowels function which counts the number of vowels using the string class as follows: int countVowels(const string& s) Write a test program that prompts the user to enter a string, invokes the countVowels function and displays the total number of vowels in the string.arrow_forwardJava coding Strings as argumentsarrow_forward
- c# onlyarrow_forwardPalindromes - “A palindrome” is a string that reads the same from both directions. For example: the word "mom" is a palindrome. Also, the string "Murder for a jar of red rum" is a palindrome. - So, you need to implement a Boolean function that takes as input a string and its return is true (1) in case the string is a palindrome and false (0) otherwise. - There are many ways to detect if a phrase is a palindrome. The method that you will implement in this task is by using two stacks. This works as follows. Push the left half of the characters to one stack (from left to right) and push the second half of the characters (from right to left) to another stack. Pop from both stacks and return false if at any time the two popped characters are different. Otherwise, you return true after comparing all the elements. Phrases of odd length have to be treated by skipping the middle element like the word "mom", your halves are "m" and "m". - Hint: (without using STL)arrow_forwardPalindromes - “A palindrome” is a string that reads the same from both directions. For example: the word "mom" is a palindrome. Also, the string "Murder for a jar of red rum" is a palindrome. - So, you need to implement a Boolean function that takes as input a string and its return is true (1) in case the string is a palindrome and false (0) otherwise. - There are many ways to detect if a phrase is a palindrome. The method that you will implement in this task is by using two stacks. This works as follows. Push the left half of the characters to one stack (from left to right) and push the second half of the characters (from right to left) to another stack. Pop from both stacks and return false if at any time the two popped characters are different. Otherwise, you return true after comparing all the elements. Phrases of odd length have to be treated by skipping the middle element like the word "mom", your halves are "m" and "m". - Hint: (without using STL)arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
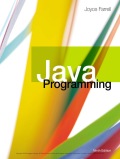
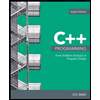