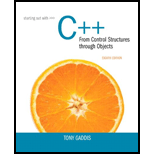
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16, Problem 9PC
Program Plan Intro
Modification of “SearchableVector” class
Program plan:
SimpleVector.h:
- Include the required header files in the program.
- Define the template class “SimpleVector”:
- Declare the required class data members and exception function under “private” access specifier.
- Declare the required class member functions and class constructor under “public” access specifier.
- Define the “SimpleVector” function:
- Get the array size and allocate the memory for corresponding array size
- If allocation gets fail, then call the “memError()” function.
- Else initialize the array elements.
- Define the copy constructor of the “SimpleVector” function:
- Copy the array size and allocate the memory for corresponding array size
- If allocation gets fail, then call the “memError()” function.
- Else copy the array elements.
- Define the “~SimpleVector” class destructor:
- Delete the allocated memory for the array.
- Define the “getElement” function:
- Get the array subscript and check it is in the array range.
- If not then call the exception function.
- Return the subscript.
- Define the operator overload for “[]” operator
- Get the array subscript and check it is in the array range.
- If not then call the exception function.
- Return the subscript.
- Define the “push_back()” function:
- Get the element which is going to insert.
- Create a new array and allocate the memory for the array which is greater than 1 from an old array.
- Copy the elements from old array to new array.
- Insert the new element at the last of the array.
- Delete the old array and make the pointer points the new array
- Finally adjust the array size.
- Define the “pop_back()” function:
- Save the last element which is going to delete.
- Create a new array and allocate the memory for the array which is lesser than 1 from an old array.
- Copy the elements from old array to new array.
- Delete the old array and make the pointer points the new array
- Finally adjust the array size.
- Return the deleted value.
SearchableVector.h:
- Include the required header files in the program.
- Define the template class “SearchableVector”, which is derived from the above class template “SimpleVector”:
- Declare the required class member functions and class constructor under “public” access specifier.
- Define the copy constructor of the “SearchableVector” function:
- Call the base class copy constructor for copying the array size and allocate the memory for corresponding array size.
- Copy the array elements.
- Define the function “findItem”
- Declare the required variables in the function.
- Get the element from the function call.
- Using while loop
- Find the middle element of the array.
- Check the middle element is the searching element.
- If so, then return the array subscript.
- Else, check the middle element is greater than the searching element.
- If so, decrease the last index value.
- Else, increase the starting index value.
- If the element is not found, then return -1 as the array subscript.
Main.cpp:
- Include the required header files in the program.
- Create an object for the class “SearchableVector” for integer type.
- Create an object for the class “SearchableVector” for double type.
- Use for loop to iterate array elements
- Insert the integer value on integer array.
- Insert the double value on double array.
- Using for loop, display an integer array on the output screen.
- Using for loop, display the double array on the output screen.
- Call the function “findItem” using the searching element which is integer.
- Compare the result of the function and display the message according to the condition.
- Do the same again using double value.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
member and non-member functions
c++
Computer Science
//iterator() creates a new Iterator over this list. It will//initially be referring to the first value in the list, unless the//list is empty, in which case it will be considered both "past start"//and "past end".
template <typename ValueType>typename DoublyLinkedList<ValueType>::Iterator DoublyLinkedList<ValueType>::iterator(){//return iterator(head);}
//constIterator() creates a new ConstIterator over this list. It will//initially be referring to the first value in the list, unless the//list is empty, in which case it will be considered both "past start"//and "past end".
template <typename ValueType>typename DoublyLinkedList<ValueType>::ConstIterator DoublyLinkedList<ValueType>::constIterator() const{//return constIterator(head);}
//Initializes a newly-constructed IteratorBase to operate on//the given list. It will initially be referring to the first//value in the list, unless the list is empty, in which case//it will be…
Data Structure & Algorithum java program
Do the following:
1) Add a constructor to the class "LList" that creates a list from a given array of objects.2) Add a method "addAll" to the "LList" class that adds an array of items to the end of the list. The header of the method is as follows, where "T" is the generic type of the objects in the list. 3) Write a Test/Driver program that thoroughly tests all the methods in the class "LList".
Chapter 16 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.1 - Prob. 16.5CPCh. 16.3 - Prob. 16.6CPCh. 16.3 - The following function accepts an i nt argument...Ch. 16.3 - Prob. 16.8CPCh. 16.3 - Prob. 16.9CPCh. 16.4 - Prob. 16.10CP
Ch. 16.4 - Prob. 16.11CPCh. 16 - Prob. 1RQECh. 16 - Prob. 2RQECh. 16 - Prob. 3RQECh. 16 - Prob. 4RQECh. 16 - What is unwinding the stack?Ch. 16 - What happens if an exception is thrown by a classs...Ch. 16 - How do you prevent a program from halting when the...Ch. 16 - Why is it more convenient to write a function...Ch. 16 - Why must you be careful when writing a function...Ch. 16 - Prob. 10RQECh. 16 - Prob. 11RQECh. 16 - Prob. 12RQECh. 16 - The line containing a throw statement is known as...Ch. 16 - Prob. 14RQECh. 16 - Prob. 15RQECh. 16 - Prob. 16RQECh. 16 - The beginning of a template is marked by a(n)...Ch. 16 - Prob. 18RQECh. 16 - Prob. 19RQECh. 16 - Prob. 20RQECh. 16 - Prob. 21RQECh. 16 - _____________ are pointer-like objects used to...Ch. 16 - Prob. 23RQECh. 16 - Write a function that searches a numeric array for...Ch. 16 - Write a function that dynamically allocates a...Ch. 16 - Make the function you wrote in Question 17 a...Ch. 16 - Write a template for a function that displays the...Ch. 16 - Prob. 28RQECh. 16 - Prob. 29RQECh. 16 - Prob. 30RQECh. 16 - Prob. 31RQECh. 16 - Prob. 32RQECh. 16 - Prob. 33RQECh. 16 - Prob. 34RQECh. 16 - T F All type parameters defined in a function...Ch. 16 - Prob. 36RQECh. 16 - T F A class object passed to a function template...Ch. 16 - Prob. 38RQECh. 16 - Prob. 39RQECh. 16 - Prob. 40RQECh. 16 - Prob. 41RQECh. 16 - T F A class template may not be derived from...Ch. 16 - T F A class template may not be used as a base...Ch. 16 - Prob. 44RQECh. 16 - Prob. 45RQECh. 16 - Prob. 46RQECh. 16 - Prob. 47RQECh. 16 - try { quotient = divide(num1, num2); } cout The...Ch. 16 - template class T T square(T number) { return T T;...Ch. 16 - template class T int square(int number) { return...Ch. 16 - Prob. 51RQECh. 16 - Assume the following definition appears in a...Ch. 16 - Assume the following statement appears in a...Ch. 16 - Prob. 1PCCh. 16 - Prob. 2PCCh. 16 - Prob. 3PCCh. 16 - Prob. 4PCCh. 16 - Prob. 5PCCh. 16 - IntArray Class Exception Chapter 14 presented an...Ch. 16 - TestScores Class Write a class named TestScores....Ch. 16 - Prob. 9PCCh. 16 - SortableVector Class Template Write a class...Ch. 16 - Inheritance Modification Assuming you have...Ch. 16 - Prob. 12PCCh. 16 - Prob. 13PCCh. 16 - 14. Test Scores vector
Modify Programming...Ch. 16 - Prob. 15PCCh. 16 - Prob. 16PCCh. 16 - Prob. 17PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Concatenate Map This function will be given a single parameter known as the Map List. The Map List is a list of maps. Your job is to combine all the maps found in the map list into a single map and return it. There are two rules for addingvalues to the map. You must add key-value pairs to the map in the same order they are found in the Map List. If the key already exists, it cannot be overwritten. In other words, if two or more maps have the same key, the key to be added cannot be overwritten by the subsequent maps. Signature: public static HashMap<String, Integer> concatenateMap(ArrayList<HashMap<String, Integer>> mapList) Example: INPUT: [{b=55, t=20, f=26, n=87, o=93}, {s=95, f=9, n=11, o=71}, {f=89, n=82, o=29}]OUTPUT: {b=55, s=95, t=20, f=26, n=87, o=93} INPUT: [{v=2, f=80, z=43, k=90, n=43}, {d=41, f=98, y=39, n=83}, {d=12, v=61, y=44, n=30}]OUTPUT: {d=41, v=2, f=80, y=39, z=43, k=90, n=43} INPUT: [{p=79, b=10, g=28, h=21, z=62}, {p=5, g=87, h=38}, {p=29,…arrow_forwardFunction 1: draw_subregion Complete the implementation of draw_subregion. This function has the following parameters: my_turtle: A turtle object (which will do the drawing) polygon_points: A list of (x, y) points (i.e. a list of tuples) that defines the points of a polygon. This function should make the turtle draw the polygon given by the points. Make sure that you lift your pen before heading to the first point. You should also make sure you return to the very first point at the end (i.e. you will go to the first point in the list two times: once at the beginning, and once at the end). Language Pythonarrow_forwardUnordered Sets |As explained in this chapter, a set is a collection of distinct elements of the same type. Design the class unorderedSetType, derived from the class unorderedArrayListType, to manipulate sets. Note that you need to redefine only the functions insertAt, insertEnd, and replaceAt. If the item to be inserted is already in the list, the functions insertAt and insertEnd output an appropriate message, such as 13 is already in the set. Similarly, if the item to be replaced is already in the list, the function replaceAt outputs an appropriate message. Also, write a program to test your class.arrow_forward
- Fill in the blanksarrow_forwardExercise, maxCylinderVolume F# system function such as min or methods in the list module such as List.map are not allowed Write a function maxCylinderVolume that takes a list of floating-point tuples that represent dimensions of a cylinder and returns the volume of the cylinder that has the largest volume. Each tuple has two floating point values that are both greater than zero. The first value is the radius r and the second value is the height h. The volume of the cylinder is computed using ??2h. The value π is represented in F# with System.Math.PI. If the list is empty, return 0.0. Examples: > maxCylinderVolume [(2.1, 3.4); (4.7, 2.8); (0.9, 6.1); (3.2, 5.4)];;val it : float = 194.3137888> maxCylinderVolume [(0.33, 0.66)];;val it : float = 0.2257988304arrow_forwardRemove is a function that has been defined.arrow_forward
- Reference-based Linked Lists: Select all of the following statements that are true. As a singly linked list's node references both its predecessor and its successor, it is easily possible to traverse such a list in both directions. According to the terminology introduced in class, the head reference variable in a singly linked list object references the list's first node. According to the terminology introduced in class, in a doubly linked list, each node references both the head and tail node. In a double-ended singly linked list, the tail reference variable provides access to the entire list. In a circular linked list, the last node references the first node.arrow_forwardProgramming Language: C++ I need the codes for arrayListType.h, main.cpp, myString.cpp, myString.harrow_forward4. Absolute Value Template Write a function template that accepts an argument and returns its absolute value. The absolute value of a number is its value with no sign. For example, the absolute value of -5 is 5, and the absolute value of 2 is 2. Test the template in a simple driver program being sure to send the template short, int, double, float, and long data values. SAMPLE RUN #4: ./AbsoluteValueTemplate Hide Invisibles Highlight: None Show Highlighted Only O Interactive Session Enter.a short.or.just.enter to-move.to.next.data type:-124 The absolute. value of.-12.is: 124 Enter.a short.or.just.enter to-move to. next data type:-11- The absolute value of.-11·is: 11e Enter.a short.or.just.enter to-move.to.next.data -type:-84 The absolute value of. -8.is: 84 Enter.a short.or.just.enter to-move to-next.data type:88e The absolute value of. 88 -is: 88e Enter.a short.or.just.enter to-move.to.next data type:45e The absolute value of.45.is: 45- Enter.a short.or.just.enter to-move -to-next.data…arrow_forward
- getting error please helparrow_forwardFollowing is the member function clear() in the class template List, please complete this function with a single looping statement. template void List::clear() { }arrow_forwardC++ Format Please Write a function (named "Lookup") that takes two const references to vectors. The first vector is a vector of strings. This vector is a list of words. The second parameter is a list of ints. Where each int denotes an index in the first vector. The function should return a string formed by concatenated the words (separated by a space) of the first vector in the order denoted by the second vector. Input of Test Case provided in PDF:arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
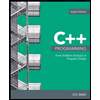
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning