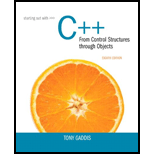
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 16, Problem 10PC
SortableVector Class Template
Write a class template named SortableVector. The class should be derived from the SimpleVector class presented in this chapter. It should have a member function that sorts the array elements in ascending order. (Use the sorting
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Q2/find the transfer function C/R for the system shown in the figure
Re
ད
Please original work
select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project
Please cite in text references and add weblinks
Please original work
What topic would be related to architectures or infrastructures.
How you would implement your chosen topic in a data warehouse project.
Please cite in text references and add weblinks
Chapter 16 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.1 - Prob. 16.5CPCh. 16.3 - Prob. 16.6CPCh. 16.3 - The following function accepts an i nt argument...Ch. 16.3 - Prob. 16.8CPCh. 16.3 - Prob. 16.9CPCh. 16.4 - Prob. 16.10CP
Ch. 16.4 - Prob. 16.11CPCh. 16 - Prob. 1RQECh. 16 - Prob. 2RQECh. 16 - Prob. 3RQECh. 16 - Prob. 4RQECh. 16 - What is unwinding the stack?Ch. 16 - What happens if an exception is thrown by a classs...Ch. 16 - How do you prevent a program from halting when the...Ch. 16 - Why is it more convenient to write a function...Ch. 16 - Why must you be careful when writing a function...Ch. 16 - Prob. 10RQECh. 16 - Prob. 11RQECh. 16 - Prob. 12RQECh. 16 - The line containing a throw statement is known as...Ch. 16 - Prob. 14RQECh. 16 - Prob. 15RQECh. 16 - Prob. 16RQECh. 16 - The beginning of a template is marked by a(n)...Ch. 16 - Prob. 18RQECh. 16 - Prob. 19RQECh. 16 - Prob. 20RQECh. 16 - Prob. 21RQECh. 16 - _____________ are pointer-like objects used to...Ch. 16 - Prob. 23RQECh. 16 - Write a function that searches a numeric array for...Ch. 16 - Write a function that dynamically allocates a...Ch. 16 - Make the function you wrote in Question 17 a...Ch. 16 - Write a template for a function that displays the...Ch. 16 - Prob. 28RQECh. 16 - Prob. 29RQECh. 16 - Prob. 30RQECh. 16 - Prob. 31RQECh. 16 - Prob. 32RQECh. 16 - Prob. 33RQECh. 16 - Prob. 34RQECh. 16 - T F All type parameters defined in a function...Ch. 16 - Prob. 36RQECh. 16 - T F A class object passed to a function template...Ch. 16 - Prob. 38RQECh. 16 - Prob. 39RQECh. 16 - Prob. 40RQECh. 16 - Prob. 41RQECh. 16 - T F A class template may not be derived from...Ch. 16 - T F A class template may not be used as a base...Ch. 16 - Prob. 44RQECh. 16 - Prob. 45RQECh. 16 - Prob. 46RQECh. 16 - Prob. 47RQECh. 16 - try { quotient = divide(num1, num2); } cout The...Ch. 16 - template class T T square(T number) { return T T;...Ch. 16 - template class T int square(int number) { return...Ch. 16 - Prob. 51RQECh. 16 - Assume the following definition appears in a...Ch. 16 - Assume the following statement appears in a...Ch. 16 - Prob. 1PCCh. 16 - Prob. 2PCCh. 16 - Prob. 3PCCh. 16 - Prob. 4PCCh. 16 - Prob. 5PCCh. 16 - IntArray Class Exception Chapter 14 presented an...Ch. 16 - TestScores Class Write a class named TestScores....Ch. 16 - Prob. 9PCCh. 16 - SortableVector Class Template Write a class...Ch. 16 - Inheritance Modification Assuming you have...Ch. 16 - Prob. 12PCCh. 16 - Prob. 13PCCh. 16 - 14. Test Scores vector
Modify Programming...Ch. 16 - Prob. 15PCCh. 16 - Prob. 16PCCh. 16 - Prob. 17PC
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Determine the resultant internal normal force, shear force, and bending moment at point C in the beam.
Mechanics of Materials (10th Edition)
Explain what must be done when fully replicating a database but allowing only one computer to process updates.
Database Concepts (8th Edition)
Each byte is assigned a unique a. address b. CPU c. bit d. variable
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
When displaying a Java applet, the browser invokes the _____ to interpret the bytecode into the appropriate mac...
Web Development and Design Foundations with HTML5 (8th Edition)
Sales Report Brewster's Used Cars, Inc. employs several salespeople. Brewster, the owner of the company, has pr...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- What is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forwardDiscuss with appropriate examples the types of relationships in a database. Give one reference with your answer.arrow_forward
- Determine the velocity error constant (k,) for the system shown. + R(s)- K G(s) where: K=1.6 A(s+B) G(s) = as²+bs C(s) where: A 14, B =3, a =6. and b =10arrow_forward• Solve the problem (pls refer to the inserted image)arrow_forwardWrite .php file that saves car booking and displays feedback. There are 2 buttons, which are <Book it> <Select a date>. <Select a date> button gets an input from the user, start date and an end date. Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, it books cars for specific dates, with bookings saved. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. In the booking.json file, if the Car ID and start date and end date matches, it fails Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert).arrow_forward
- Write .php file with the html that saves car booking and displays feedback. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. There are 2 buttons, which are <Book it> <Select a date> Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, book cars for specific dates, with bookings saved. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert). And then add an additional feature that only free dates are selectable (e.g., calendar view).arrow_forward• Solve the problem (pls refer to the inserted image) and create line graph.arrow_forwardwho started the world wide webarrow_forward
- Question No 1: (Topic: Systems for collaboration and social business The information systems function in business) How does Porter's competitive forces model help companies develop competitive strategies using information systems? • List and describe four competitive strategies enabled by information systems that firms can pursue. • Describe how information systems can support each of these competitive strategies and give examples.arrow_forwardData communıcatıon digital data is transmitted via analog ASK and PSK are used together to increase the number of bits transmitted a)For m=8,suggest a solution and define signal elements , and then draw signals for the following sent data data = 0 1 0 1 1 0 0 0 1 0 1 1arrow_forwardDatacommunicationData = 1 1 0 0 1 0 0 1 0 1 1 1 1 0 0a) how many bıts can be detected and corrected by this coding why prove?b)what wıll be the decision of the reciever if it recieve the following codewords why?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
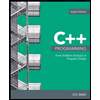
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
1.1 Arrays in Data Structure | Declaration, Initialization, Memory representation; Author: Jenny's lectures CS/IT NET&JRF;https://www.youtube.com/watch?v=AT14lCXuMKI;License: Standard YouTube License, CC-BY
Definition of Array; Author: Neso Academy;https://www.youtube.com/watch?v=55l-aZ7_F24;License: Standard Youtube License