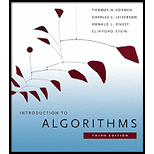
(a)
To discuss the greedy
(a)

Explanation of Solution
To prove that the changing problem of coin has an optimal solution, take ' n ' cent and suppose, for these ' n ' cent changes, there is an optimal solution. The solution takes a coin with value ' c ' and uses ' k ' coins. If the solution of ' n-c ' cent changes problem lies within the solution of ' n ' cent problem solution, that means ' k-1 ' coins are available for ' n-c ' cents. So, take the solution for the ' n-c ' cent changing problem and make sure this should be lesser then ' k- 1 ' coins then figure out the solution for ' n ' cent problem.
While dealing with ' n ' cent problem and try to get an optimal solution by taking minimum no. of coins. Always provide the biggest denomination coin until the rest amount became zero. So, if there is the biggest denomination present at first then by applying greedy methodology changes in quarter, dime, nickel and pennies are:
Q = ⌊ n /25 ⌋ quarter that gives nQ = n mod 25 cent for change.
D = ⌊ nQ /10 ⌋ dimes that gives nD = nQ mod 10 cent for change.
K = ⌊ nD /10 ⌋ dimes that gives nK = nD mod 5 cent for change.
P = nK pennies
By seeing the greedy solution, there are few possibilities like:
- If n = 0; then zero coins in optimal solution.
- If n > 0; then get the biggest denomination with value = ' n ' , suppose it’s ' c ' .
- Now, take a coin and apply process in recursive manner for ' n-c ' cent.
To get the optimal solution through greedy algorithm, one must hold the coin ' c ' with value greater than ' n ' .
There are four cases needs to be considered here:
- If (1= n < 5) then c = 1, this must hold the greedy choice and contains pennies.
- If (5 = n = 10) then c = 5, by supposition, in this it must hold pennies and change 5 pennies to one nickel because this solution is not holding any nickel.
- If (10 = n = 25) then c = 10, by supposition, it must hold a nickel and pennies but not a dime. So, add a dime in the solution.
- If (25 = n ) then c = 25, by supposition, it holds a dime, pennies, and nickel but not quarter. If it has three dimes change it with a quarter and nickel and if has at least two dime then few subset of nickel, dime and pennies add nearly 25 cents and change these coins with a quarter to get a solution.
Here, for showing that it must contains an optimal solution with greedy choices and these choices will further helpful to get the solution of sub problem. Therefore, this greedy approach provides an optimal outcome for the given problem.
For an algorithm that selected a coin and recurses sub problem one by one, the running complexity will be θ ( k ), where ' k ' is the coins used for optimal solution. Now ( k = n), so, running time will be O ( n ) and there is only four type of coins. So, it requires O (1) constant time.
(b)
To show that for given denomination ( c0, c1, c2, ........., ck ), greedy algorithm always gives an optimal solution where ( c > 1) and (1 = k ).
(b)

Explanation of Solution
Take denomination c0, c1, c2, ........., ck and changes amount ' n ' . By taking this, it is clear that
Only few coins are required in changing coin to give an optimal solution. Apply greedy algorithm, take biggest denomination coin first means take a denomination ci (not ck ), where ( k>i ).
- To make sure that solution should be optimal take any denomination ( ci ) no. of coin that must be lesser then ' c ' .
- Take coins of ' ci ' denomination having ( x0, x1, ...., xk ) optimal solution and prove that for every ( k >i ), denomination ( c >xi ).
- And for few j , ( c = xi ) and change denomination coins cj with cj+1coin .
- Therefore, xj reduced by c, xj +1 increased by 1 and total no. of coins reduced by c -1.
This is contradicting about ( x0, x1,....., xk ) being a solution. So, that optimal solution should have denomination ' c ' greater than xi for any ' ci ' denomination.
Thus the only solution of the given problem is xk = n/ck ; for ( k>i ), xi = n mod ci +1/ ci . Therefore this is proved that greedy algorithm with ( c0, c1, c2, ........., ck ) denomination always gives an optimal solution.
(c)
To prove that greedy algorithm does not provides the optimal solution all the time.
(c)

Explanation of Solution
Take the denomination set as {1, 3, 4} and the value of ' n =6’.
Then greedy solution will give two one cent coin {1, 1} and a four-cent coin {4} like {1, 1, 4} but two coins as {3, 3} is optimal solution.
So, for the set {1, 3, 4}, optimal solution will not present by using greedy algorithm.
In the other example where denomination set contains value {1, 10, 25}, for ' n = 30’; greedy algorithm provide a quarter and five pennies {25, 1, 1, 1, 1, 1} but the optimal solution should be three dime like {10, 10, 10}.
Thus, greedy algorithm will not provide an optimal solution always.
(d)
To show that the time complexity of the algorithm is O ( nk ), where ' k ' is the total no. of coins.
(d)

Explanation of Solution
While applying the dynamic programming, take the demonstration ' d ' define as d1 , d2 , d3 ,...., dk and to make changes for ' i ' cent, no. of coins required min( c [ i ]). So, the minimum coins required can be calculated as:
c [ i ] = 1 + min{ c [ i-dj ]}, if ( i > 0) and (1 = j = k ),
c [ i ] = 0, if ( i = 0)
This formula should be implemented as,
MakeChange ( d,n )
- For ( i ← 1) to n
- Do c [ i ] ← 8
- For ( j ← 1) to k
- Do if ( dj = i )
- Then if c [ i ] > c [ i-dj ] + 1
- Then c [ i ] ← c [ i - dj ] + 1
- denom[ i ] ← dj
- Return demon and ' c '
By seeing the algorithmic procedure of MakeChange( d,n ), it is showing that it requires two loops up to ' n ' times as outer loop and ' k ' times as inner loop.
Thus, the complexity of the implementation will be O ( nk ).
Want to see more full solutions like this?
Chapter 16 Solutions
Introduction to Algorithms
- What is a functional decomposition diagram? what is a good example of a high level task being broken down into tasks in at least two lower levels (three levels in all).arrow_forwardWhat are the advantages to using a Sytems Analysis and Design model like the SDLC vs. other approaches?arrow_forward3. Problem Description: Define the Circle2D class that contains: Two double data fields named x and y that specify the center of the circle with get methods. • A data field radius with a get method. • A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius. • A constructor that creates a circle with the specified x, y, and radius. • A method getArea() that returns the area of the circle. • A method getPerimeter() that returns the perimeter of the circle. • • • A method contains(double x, double y) that returns true if the specified point (x, y) is inside this circle. See Figure (a). A method contains(Circle2D circle) that returns true if the specified circle is inside this circle. See Figure (b). A method overlaps (Circle2D circle) that returns true if the specified circle overlaps with this circle. See the figure below. р O со (a) (b) (c)< Figure (a) A point is inside the circle. (b) A circle is inside another circle. (c) A circle overlaps another…arrow_forward
- 1. Explain in detail with examples each of the following fundamental security design principles: economy of mechanism, fail-safe default, complete mediation, open design, separation of privilege, least privilege, least common mechanism, psychological acceptability, isolation, encapsulation, modularity, layering, and least astonishment.arrow_forwardSecurity in general means the protection of an asset. In the context of computer and network security, explore and explain what assets must be protected within an online university. What the threats are to the security of these assets, and what countermeasures are available to mitigate and protect the organization from such threats. For each of the assets you identify, assign an impact level (low, moderate, or high) for the loss of confidentiality, availability, and integrity. Justify your answers.arrow_forwardPlease include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forward
- Object-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forwardWhen using functions in python, it allows us tto create procedural abstractioons in our programs. What are 5 major benefits of using a procedural abstraction in python?arrow_forward
- Find the error, assume data is a string and all variables have been declared. for ch in data: if ch.isupper: num_upper = num_upper + 1 if ch.islower: num_lower = num_lower + 1 if ch.isdigit: num_digits = num_digits + 1 if ch.isspace: num_space = num_space + 1arrow_forwardFind the Error: date_string = input('Enter a date in the format mm/dd/yyyy: ') date_list = date_string.split('-') month_num = int(date_list[0]) day = date_list[1] year = date_list[2] month_name = month_list[month_num - 1] long_date = month_name + ' ' + day + ', ' + year print(long_date)arrow_forwardFind the Error: full_name = input ('Enter your full name: ') name = split(full_name) for string in name: print(string[0].upper(), sep='', end='') print('.', sep=' ', end='')arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningOperations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks Cole
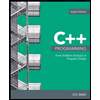
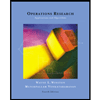