HELP CHAT GPT GAVE ME WRONG ANSWER Consider the following implementation of a container that will be used in a concurrent environment. The container is supposed to be used like an indexed array, but provide thread-safe access to elements. struct concurrent_container { // Assume it’s called for any new instance soon before it’s ever used void concurrent_container() { init_mutex(&lock); } ~concurrent_container() { destroy_mutex(&lock); } // Returns element by its index. int get(int index) { lock.acquire(); if (index < 0 || index >= size) { return -1; } int result = data[index]; lock.release(); return result; } // Sets element by its index. void set(int index, int value) { lock.acquire(); if (index < 0 || index >= size) { resize(size); } data[index] = value; lock.release(); } // Extend maximum capacity of the container so we can add more elements void resize(int n) { lock.acquire(); int* new_data = (int*) malloc(n * sizeof(int)); if (new_data == nullptr) { lock.release(); return; } // Ensure we store 0 for elements that have never been set for (int i = 0; i < size; i++) { new_data[i] = 0; } free(data); data = new_data; size = n; lock.release(); } // Assume it’s only called when the container object is destroyed and no one is going to use it anymore void destroy() { free(data); data = nullptr; size = 0; destroy_mutex(&lock); } // Assume nobody (except the member methods) is ever going to access these fields directly int* data; int size; mutex lock;}; Which of the following problems do you think are present in the implementation? You may select multiple options. Pick ONE OR MORE options: The implementation can cause a deadlock. The implementation can cause a resource leak. The implementation doesn’t prevent multiple threads from accessing data at the same time. The implementation can cause a memory corruption. The implementation can result in unaligned memory access, which is forbidden on some platforms. The implementation doesn’t do proper handling of invalid input. The implementation is not protected from integer overflow errors. The implementation is actually correct and thread-safe.
HELP CHAT GPT GAVE ME WRONG ANSWER
Consider the following implementation of a container that will be used in a concurrent environment. The container is supposed to be used like an indexed array, but provide thread-safe access to elements.
struct concurrent_container {
// Assume it’s called for any new instance soon before it’s ever used
void concurrent_container() {
init_mutex(&lock);
}
~concurrent_container() {
destroy_mutex(&lock);
}
// Returns element by its index.
int get(int index) {
lock.acquire();
if (index < 0 || index >= size) {
return -1;
}
int result = data[index];
lock.release();
return result;
}
// Sets element by its index.
void set(int index, int value) {
lock.acquire();
if (index < 0 || index >= size) {
resize(size);
}
data[index] = value;
lock.release();
}
// Extend maximum capacity of the container so we can add more elements
void resize(int n) {
lock.acquire();
int* new_data = (int*) malloc(n * sizeof(int));
if (new_data == nullptr) {
lock.release();
return;
}
// Ensure we store 0 for elements that have never been set
for (int i = 0; i < size; i++) {
new_data[i] = 0;
}
free(data);
data = new_data;
size = n;
lock.release();
}
// Assume it’s only called when the container object is destroyed and no one is going to use it anymore
void destroy() {
free(data);
data = nullptr;
size = 0;
destroy_mutex(&lock);
}
// Assume nobody (except the member methods) is ever going to access these fields directly
int* data;
int size;
mutex lock;
};
Which of the following problems do you think are present in the implementation? You may select multiple options.
Pick ONE OR MORE options:
- The implementation can cause a deadlock.
- The implementation can cause a resource leak.
- The implementation doesn’t prevent multiple threads from accessing data at the same time.
- The implementation can cause a memory corruption.
- The implementation can result in unaligned memory access, which is forbidden on some platforms.
- The implementation doesn’t do proper handling of invalid input.
- The implementation is not protected from integer overflow errors.
- The implementation is actually correct and thread-safe.

Step by step
Solved in 2 steps

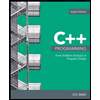
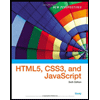
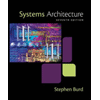
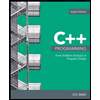
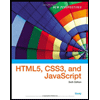
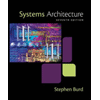
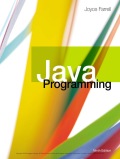
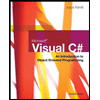
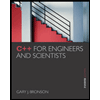