STARTING OUT WITH C++ MPL
9th Edition
ISBN: 9780136673989
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 15, Problem 2PC
Program Plan Intro
Analysis of Quicksort
Program Plan:
- Include the required header files to the program.
- Define “AbstractSort” class.
- In public, declare the pure virtual function.
- In the “get_comparison” function return the total number of comparison.
- In protected, declare the “compare” function.
- In the “reset_comparison” function reset the comparison value to “0”.
- In private, declare the required variable.
- In public, declare the pure virtual function.
- Define the “compare” function outside the class definition.
- Inside the function, increment the comparison count and return the number of comparison.
- Define the derived class “Quicksort”.
- In public, declare the “sort” function.
- In private, declare the “quick” function and “partition” function.
- Define the “quick” function.
- If the least number is greater than the highest number, return the value to the function.
- Call the “partition”, quick” functions.
- Define the “partition” function.
- Set the pivot value.
- Declare and set the “front” variable.
- If the “front” value is less than “u”, swap the values and increment the pivot variable.
- Increment the “front” variable.
- Define the “main()” function.
- Declare and initialize the required variables.
- Get the array value from the user.
- Check the array value with array index.
- If the array value is greater than index value exits the program.
- Initialize the random number generator and generate the random numbers.
- Create the object for the class “Quicksort”.
- Call the “sort” function.
- Display the result.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Don't use vector array .using c++ language
Course: Data Structure and Algorithms
Language: C++
Question is well explained
Question #2Implement a class for Circular Doubly Linked List (with a dummy header node) which stores integers in unsorted order. Your class definitions should look like as shown below:
class CDLinkedList;class DNode {friend class CDLinkedList;private int data;private DNode next;private DNode prev;};class CDLinkedList
{private:DNode head; // Dummy header nodepublic CDLinkedList(); // Default constructorpublic bool insert (int val); public bool removeSecondLastValue (); public void findMiddleValue(); public void display(); };
python assignment
Matrix class
Implement a matrix class (in matrix.py).
a) The initializer should take a list of lists as an argument, where each outer list is a row, and each value in an inner list is a value in the corresponding row.
b) Implement the __str__ method to nicely format the string representation of the matrix: one line per row, two characters per number (%2d) and a space between numbers. For example:
m = Matrix([[1,0,0],[0,1,0],[0,0,1]])
print(m)> 1 0 0> 0 1 0> 0 0 1
c) Implement a method scale(factor) that returns a new matrix where each value is multiplied by scale. For example:
m = Matrix([[1,2,3],[4,5,6],[7,8,9]])n = m.scale(2)print(n)> 2 4 6> 8 10 12>14 16 18print(m)> 1 2 3> 4 5 6> 7 8 9
d) Implement a method transpose() that returns a new matrix that has been transposed. Transposing flips a matrix over its diagonal: it switches rows and columns.
m = Matrix([[1,2,3],[4,5,6],[7,8,9]])print(m)> 1 2 3> 4 5 6> 7 8…
Chapter 15 Solutions
STARTING OUT WITH C++ MPL
Ch. 15.3 - Prob. 15.1CPCh. 15.3 - Prob. 15.2CPCh. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - How can you tell from looking at a class...Ch. 15.3 - What makes an abstract class different from other...Ch. 15.3 - Examine the following classes. The table lists the...Ch. 15 - A class that cannot be instantiated is a(n) _____...
Ch. 15 - A member function of a class that is not...Ch. 15 - A class with at least one pure virtual member...Ch. 15 - In order to use dynamic binding, a member function...Ch. 15 - Static binding takes place at _____ time.Ch. 15 - Prob. 6RQECh. 15 - Prob. 7RQECh. 15 - Prob. 8RQECh. 15 - The is-a relation between classes is best...Ch. 15 - The has-a relation between classes is best...Ch. 15 - If every C1 class object can be used as a C2 class...Ch. 15 - A collection of abstract classes defining an...Ch. 15 - The keyword _____ prevents a virtual member...Ch. 15 - To have the compiler check that a virtual member...Ch. 15 - C++ Language Elements Suppose that the classes Dog...Ch. 15 - Will the statement pAnimal = new Cat; compile?Ch. 15 - Will the statement pCreature = new Dog ; compile?Ch. 15 - Will the statement pCat = new Animal; compile?Ch. 15 - Rewrite the following two statements to get them...Ch. 15 - Prob. 20RQECh. 15 - Find all errors in the following fragment of code,...Ch. 15 - Soft Skills 22. Suppose that you need to have a...Ch. 15 - Prob. 1PCCh. 15 - Prob. 2PCCh. 15 - Sequence Sum A sequence of integers such as 1, 3,...Ch. 15 - Prob. 4PCCh. 15 - File Filter A file filter reads an input file,...Ch. 15 - Prob. 6PCCh. 15 - Bumper Shapes Write a program that creates two...Ch. 15 - Bow Tie In Tying It All Together, we defined a...
Knowledge Booster
Similar questions
- Y3 Using C++ To test your understanding of recursion, you are charged with creating a recursive, singly linked list. You will need to make the class yourself for this data structure. This repository already contains the List ADT and a driver that will create a TUI (terminal user interface) program that uses your data structure to generate a recursive art animation. Submissions that do not compile will receive a zero. You can work on this by yourself or with one other person. LinkedList This class will represent the data structure. Since we want this data structure to be generic, you need to make it a class template. Create two files for this class: a header file (LinkedList.hpp) and an implementation file (LinkedList.tpp). Place these two files in the src directory. It needs to inherit from the List abstract class using the public access specifier. The following are implementation notes for the class. The majority of methods need to be recursive and optimized via tail recursion…arrow_forwardProgramming Language: C++ I need the codes for arrayListType.h, main.cpp, myString.cpp, myString.harrow_forwardUse the array based list headers for queue. Call your function to test its functionality. Here are the headers: #ifndef H_arrayListType #define H_arrayListType #include <iostream> using namespace std; template <class elemType> class arrayListType { public: const arrayListType<elemType>& operator=(const arrayListType<elemType>&); //Overloads the assignment operator bool isEmpty() const; //Function to determine whether the list is empty //Postcondition: Returns true if the list is empty; // otherwise, returns false. bool isFull() const; //Function to determine whether the list is full //Postcondition: Returns true if the list is full; // otherwise, returns false. int listSize() const; //Function to determine the number of elements in //the list. //Postcondition: Returns the value of length. int maxListSize() const; //Function to determine the maximum size of the list //Postcondition: Returns the value of maxSize. void print() const; //Function to…arrow_forward
- JAVA programming language DescriptionYour job is to write your own array list (growable array) that stores elements that are objects of a parentclass or any of its child classes but does not allow any other type of objects to be stored. You will createa general data class and two specific data classes that inherit from that class. You will then write thearray list to hold any objects of the general data class. Finally, you will test your array list using JUnittests to prove that it does what it should.DetailsYour array list must be named DataList. Start with an array with room for 10 elements. Keep all elementscontiguous (no blank places) at the low end of the indices. When the array is full, grow it by doubling thesize. (Do not use any built-in methods to copy the array elements. Write your own code to do this.) Youdo not have to shrink the array when elements are removed. The array list must have the followingpublic methods (listed in UML style where GenClass is your general data…arrow_forwardc++arrow_forwardc++arrow_forward
- JAVA CODE Learning Objectives: Detailed understanding of the linked list and its implementation. Practice with inorder sorting. Practice with use of Java exceptions. Practice use of generics. You have been provided with java code for SomeList<T> class. This code is for a general linked list implementation where the elements are not ordered. For this assignment you will modify the code provided to create a SortedList<T> class that will maintain elements in a linked list in ascending order and allow the removal of objects from both the front and back. You will be required to add methods for inserting an object in order (InsertInorder) and removing an object from the front or back. You will write a test program, ListTest, that inserts 25 random integers, between 0 and 100, into the linked list resulting in an in-order list. Your code to remove an object must include the exception NoSuchElementException. Demonstrate your code by displaying the ordered linked list and…arrow_forwardThis chapter describes the array implementation of queues that use a special array slot, called the reserved slot, to distinguish between an empty and a full queue. Write the definition of the class and the definitions of the function members of this queue design. Also, write a program (in main.cpp) to test various operations on a queue. //Header file QueueAsArray #ifndef H_QueueAsArray #define H_QueueAsArray #include <iostream> #include <cassert> using namespace std; template<class Type> class queueType { public: const queueType<Type>& operator=(const queueType<Type>&); // overload the assignment operator void initializeQueue(); int isEmptyQueue() const; int isFullQueue() const; Type front() const; Type back() const; void addQueue(Type queueElement); void deleteQueue(); queueType(int queueSize = 100); queueType(const queueType<Type>& otherQueue); // copy constructor…arrow_forwardDouble linked list in Java Double linked list Textbook reference Data structures and algorithms Micheal Goodricharrow_forward
- C++ A queue is essentially a waiting list. It’s a sequence of elements with a front and a back. Elements can only be added to the back of the queue and they can only be removed from the front of the queue. Elements are kept in order so that the first element to enter the queue is the first one to leave it.arrow_forwardUnordered Sets |As explained in this chapter, a set is a collection of distinct elements of the same type. Design the class unorderedSetType, derived from the class unorderedArrayListType, to manipulate sets. Note that you need to redefine only the functions insertAt, insertEnd, and replaceAt. If the item to be inserted is already in the list, the functions insertAt and insertEnd output an appropriate message, such as 13 is already in the set. Similarly, if the item to be replaced is already in the list, the function replaceAt outputs an appropriate message. Also, write a program to test your class.arrow_forwardQUESTION: NOTE: This assignment is needed to be done in OOP(c++/java), the assignment is a part of course named data structures and algorithm. A singly linked circular list is a linked list where the last node in the list points to the first node in the list. A circular list does not contain NULL pointers. A good example of an application where circular linked list should be used is a items in the shopping cart In online shopping cart, the system must maintain a list of items and must calculate total bill by adding amount of all the items in the cart, Implement the above scenario using Circular Link List. Do Following: First create a class Item having id, name, price and quantity provide appropriate methods and then Create Cart/List class which holds an items object to represent total items in cart and next pointer Implement the method to add items in the array, remove an item and display all items. Now in the main do the following Insert Items in list Display all items. Traverse…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
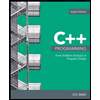
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning