Concept explainers
Soft Skills
22. Suppose that you need to have a class that can sort an array in ascending order or descending order upon request. If an array is already sorted in ascending or descending order, you can easily sort it the other way by reversing it. Now suppose you have two different classes that encapsulate arrays. One provides a member function to reverse its array, while the other provides a member function to sort its array. Can you use multiple inheritance to obtain a quick solution to your problem? Should you? Write a couple of paragraphs explaining whether using multiple inheritance will or will not work to solve this problem, and, if it can, whether this is a good way to solve the problem.

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
STARTING OUT WITH C++ MPL
Additional Engineering Textbook Solutions
Starting Out With Visual Basic (7th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
C How to Program (8th Edition)
Starting Out with Programming Logic and Design (4th Edition)
Modern Database Management (12th Edition)
Computer Science: An Overview (12th Edition)
- write an oop using photos belowarrow_forwardDevelop class Polynomial . The internal representation of a Polynomial is an array of terms. Each term contains a coefficient and an exponent—e.g., the term 2x4has the coefficient 2 and the exponent 4. Develop a complete class containing proper constructor and destructor functions as well as set and get functions. The class should also provide the following overloaded operator capabilities: "Overload the subtraction operator ( - ) to subtract two Polynomial s."arrow_forward•Person Class: Person class has attributes: String name, address and int age. Write setperson() function to set values and getPerson() to Print attributes. Also write appropriate constructors.•Employee Class: Write another class Employee having attributes department and salary of type string and double. Write methods setEmployee(), getEmployee() and appropriate constructors for Employee class.•Student Class:•Write a class Student having attributes registration number and GPA of type string and float. Also write setStudent(), getStudent() methods and required constructors. Use the concept of inheritance to achieve the above functionality. Write a main() function to display the information of employee and student.• Note: Call the constructors/methods of parent class in child class where required in java codearrow_forward
- Create a class of Person having private data members. Make all constructors along with setter getter. Also make a print function that can print all the information of that person.Class Person{Private:char* name;int age;Job job;};Class Job{Private:int id;char * role;int salary;};arrow_forward•Person Class: Person class has attributes: String name, address and int age. Write setperson() function to set values and getPerson() to Print attributes. Also write appropriate constructors. •Employee Class: Write another class Employee having attributes department and salary of type string and double. Write methods setEmployee(), getEmployee() and appropriate constructors for Employee class. •Student Class: •Write a class Student having attributes registration number and GPA of type string and float. Also write setStudent(), getStudent() methods and required constructors. Use the concept of inheritance to achieve the above functionality. Write a main() function to display the information of employee and student. • Note: Call the constructors/methods of parent class in child class where requiredarrow_forwardPlease solution in c++arrow_forward
- C++arrow_forwardNovice: How can i access a main class objects from outside the function? / Better Alternative? If i have 4 Student objects how can i print the information for the specific object given one parameter about the class, like the students Idnumber. What i came up with was making a checkId void function that takes the user input and runs an if else chain checking if the Id belongs to student 1-4 then printing the details of that classes object with the void function print. ideally i would want the if-else chain in the checkId function to call the print( student1-4) class object but im not sure how to properly do that. i dont think this is the best way to go about it, if you have any recommendations or alternatives please help me out.arrow_forward18. If a class is declared as final, the incorrect statement is (). A. Indicates that this class is final B. Indicates that this class is a root class C. Methods in this class cannot be overridden D. Variables in this class cannot be hidden 19. In the following description of polymorphism, the incorrect one is (). A. Polymorphism refers to "one definition, multiple implementations" B. There are three types of polymorphism: dynamic polymorphism, static polymorphism, and default polymorphism C. Polymorphism is not used to speed up code D. Polymorphism is one of the core characteristics of OOP 20. In Java, two interfaces B and C have been defined. To define a class that implements these two interfaces, the correct statement is ( ). A. interface A extends B,C B. interface A implements B,C C. class A implements B,C D. class A extends B,C 21. Given the following java code, the correct description of the usage of "super" is (). class Student extends Person{ public Student() { super(); } 3 D…arrow_forward
- need help with c++...Please explain the code and the question TOO.arrow_forwardThe base class Pet has attributes name and age. The derived class Dog inherits attributes from the base class Pet class and includes a breed attribute. Complete the program to: Create a generic pet, and print the pet's information using print_info(). Create a Dog pet, use print_info() to print the dog's information, and add a statement to print the dog's breed attribute. Ex: If the input is: Dobby 2 Kreacher 3 German Schnauzer the output is: Pet Information: Name: Dobby Age: 2 Pet Information: Name: Kreacher Age: 3 Breed: German Schnauzer Here is the original code: class Pet:def __init__(self):self.name = ''self.age = 0 def print_info(self):print('Pet Information:')print(' Name:', self.name)print(' Age:', self.age) class Dog(Pet):def __init__(self):Pet.__init__(self)self.breed = '' my_pet = Pet()my_dog = Dog() pet_name = input()pet_age = int(input())dog_name = input()dog_age = int(input())dog_breed = input() # TODO: Create generic pet (using pet_name, pet_age) and then call…arrow_forwardWrite a base class (Person) that has the following data members: String name, long NID, int age. Your class (Person) should have setters and getters for all data members. Derive a class (Student) from class (Person). Class student should have the following private data members: Int id, double GPA, string major. Class (Student) should have setters and getters for its private data members. In your main function, create an object of class (Student) and set all of its data members.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
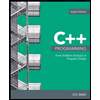
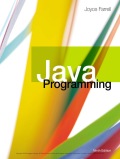
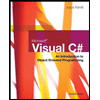