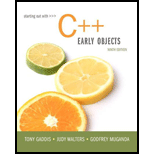
Concept explainers
Soft Skills
22. Suppose that you need to have a class that can sort an array in ascending order or descending order upon request. If an array is already sorted in ascending or descending order, you can easily sort it the other way by reversing it. Now suppose you have two different classes that encapsulate arrays. One provides a member function to reverse its array, while the other provides a member function to sort its array. Can you use multiple inheritance to obtain a quick solution to your problem? Should you? Write a couple of paragraphs explaining whether using multiple inheritance will or will not work to solve this problem, and, if it can, whether this is a good way to solve the problem.

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Starting Out with C++: Early Objects (9th Edition)
Additional Engineering Textbook Solutions
Concepts Of Programming Languages
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Database Concepts (8th Edition)
Starting Out With Visual Basic (8th Edition)
- PROGRAMMING LANGUAGE: C++ Write a program that has a base class named FlightCrew. This class shouldhave three data members: an integer to store the ID of the crew member, aninteger to store the number of years of service and another integer to storethe total salary of the member. Provide a parameterized constructor in theclass to set the values of the data members.Derive a class Pilot from FlightCrew to contain two additional data members,an integer to store the number of hours of flight and a boolean to storewhether the Pilot has military experience or not. Provide a paramete rizedconstructor in the Pilot class. Provide a function bonus() in the class wherethe bonus of a pilot is his number of flight hours times the 10% of his salary.Likewise, provide a function isEligible() in the class to find out if the pilot iseligible for promotion or not. A pilot is eligible for promotion to the nextrank if he has at least 5 years of experience and the number of total flighthours is greater…arrow_forwardclassarrow_forwardobjective of the project: Implement a class address. An address has a house number street optional apartment number city state postal code. All member variables should be private and the member functions should be public. Implement two constructors: one with an apartment number one without an appartment number. Implement a print function that prints the address with the street on one line and the city, state, and postal code on the next line. Implement a member function comesBefore that tests whether one address comes before another when the addresses are compared by postal code. Returns false if both zipcodes are equal. Use the provided main.cpp to start with. The code creates three instances of the Address class (three objects) to test your class. Each object will utilize a different constructor. You will need to add the class definition and implementation. The comesBefore function assumes one address comes before another based on zip code alone. The test will also return…arrow_forward
- write an oop using photos belowarrow_forwardDevelop class Polynomial . The internal representation of a Polynomial is an array of terms. Each term contains a coefficient and an exponent—e.g., the term 2x4has the coefficient 2 and the exponent 4. Develop a complete class containing proper constructor and destructor functions as well as set and get functions. The class should also provide the following overloaded operator capabilities: "Overload the subtraction operator ( - ) to subtract two Polynomial s."arrow_forwardThe Critter class is a base class with some basic functionality for running simulations of different kinds of critters. Critters can move, act, and remember their history. A typical simulation contains a number of critters of different types. In each step of the simulation, the act member function will be called on each critter. Define a class Sloth derived from Critter that simulates a sloth. Sloths alternate between eating and sleeping. Add the word "eat" or "sleep" to the history each time the act function is called. The implementation of the Critter class is not shown. Complete the following file: sloth_Tester.cpp #include <iostream>using namespace std; #include "critter.h" /**A sloth eats and sleeps.*/class Sloth : public Critter{public:Sloth();. . .private:. . .}; Sloth::Sloth(){. . .} void Sloth::act(){if (...) {add_history("eat");...}else{add_history("sleep");... }} int main(){Sloth sloth;sloth.act();cout << sloth.get_history() << endl;cout <<…arrow_forward
- •Person Class: Person class has attributes: String name, address and int age. Write setperson() function to set values and getPerson() to Print attributes. Also write appropriate constructors.•Employee Class: Write another class Employee having attributes department and salary of type string and double. Write methods setEmployee(), getEmployee() and appropriate constructors for Employee class.•Student Class:•Write a class Student having attributes registration number and GPA of type string and float. Also write setStudent(), getStudent() methods and required constructors. Use the concept of inheritance to achieve the above functionality. Write a main() function to display the information of employee and student.• Note: Call the constructors/methods of parent class in child class where required in java codearrow_forwardCreate a class of Person having private data members. Make all constructors along with setter getter. Also make a print function that can print all the information of that person.Class Person{Private:char* name;int age;Job job;};Class Job{Private:int id;char * role;int salary;};arrow_forward1.Use inheritance and classes to represent a deck playing cards. Create a Card class that stores the suit (e.g., Clubs, Diamonds, Hearts, Spades) and name (e.g., Ace, 2, 10, Jack) of each card along with appropriate accessors, constructors, and mutators.2.Next, create a Deck class that stores an ArrayList of Card objects. The default constructor should create objects that represent the standard 52 cards and store them in the ArrayList. The Deck class should have methods to do the following: Print every card in the deck. Shuffle the cards in the deck. You can implement this by randomly swappingevery card in the deck. Add a new card to the deck. This method should take a Card object as a parameter and add it to the ArrayList. Remove a card from the deck. This removes the first card stored in the ArrayList and returns it. Sort the cards in the deck ordered by name. 3.Next, create a Hand class that represents the cards in a hand. Hand should be derived from Deck. This is…arrow_forward
- •Person Class: Person class has attributes: String name, address and int age. Write setperson() function to set values and getPerson() to Print attributes. Also write appropriate constructors. •Employee Class: Write another class Employee having attributes department and salary of type string and double. Write methods setEmployee(), getEmployee() and appropriate constructors for Employee class. •Student Class: •Write a class Student having attributes registration number and GPA of type string and float. Also write setStudent(), getStudent() methods and required constructors. Use the concept of inheritance to achieve the above functionality. Write a main() function to display the information of employee and student. • Note: Call the constructors/methods of parent class in child class where requiredarrow_forwardC++arrow_forwardNovice: How can i access a main class objects from outside the function? / Better Alternative? If i have 4 Student objects how can i print the information for the specific object given one parameter about the class, like the students Idnumber. What i came up with was making a checkId void function that takes the user input and runs an if else chain checking if the Id belongs to student 1-4 then printing the details of that classes object with the void function print. ideally i would want the if-else chain in the checkId function to call the print( student1-4) class object but im not sure how to properly do that. i dont think this is the best way to go about it, if you have any recommendations or alternatives please help me out.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
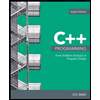
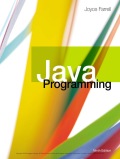
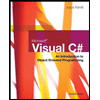