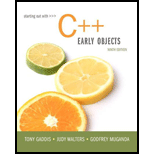
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 15, Problem 20RQE
Program Plan Intro
- Start a program.
- Declare a class named “Sorter”.
- Declare a required member variables and pure virtual function.
- In public, define the member function “set_Array()”.
- Inside the function set the array and size of an array.
- Define the “sort” function.
- Inside the function, call the “sort()” function with an argument size.
- Define the “sort” function.
- If the size is less than 1, the condition will ended.
- Find the position of largest value in array and put it at the end of the array.
- Use the “compare ()” function for sorting the given array.
- Swap a pair of array elements.
- Decrement the size by 1.
- Define the derived class “Incr_Sorter” from the class “Sorter”.
- In private, define the pure virtual function “compare()”.
- If the “x” value is greater than “y” value return true.
- In private, define the pure virtual function “compare()”.
- Define the derived class “Decr_Sorter” from the class “Sorter”.
- In private, define the pure virtual function “compare()”.
- If the “x” value is less than “y” value return true.
- In private, define the pure virtual function “compare()”.
- Inside the “main” function,
- Create the objects for the classes.
- Declare and initialize an array of 5 values.
- Call the “Incr_Sorter” function.
- Call the “print_Array()” function for displaying the output.
- Call the “Decr_Sorter” function.
- Call the “print_Array()” function for displaying the output.
- Define the “print_Array()” function.
- Display the array.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Modular Program Structure. Analysis of Structured Programming Examples. Ways to Reduce Coupling.
Based on the given problem, create an algorithm and a block diagram, and write the program code:
Function: y=xsinx
Interval: [0,π]
Requirements:
Create a graph of the function.
Show the coordinates (x and y).
Choose your own scale and show it in the block diagram.
Create a block diagram based on the algorithm.
Write the program code in Python.
Requirements:
Each step in the block diagram must be clearly shown.
The graph of the function must be drawn and saved (in PNG format).
Write the code in a modular way (functions and the main part should be separate).
Please explain and describe the results in detail.
Based on the given problem, create an algorithm and a block diagram, and write the program code:
Function: y=xsinx
Interval: [0,π]
Requirements:
Create a graph of the function.
Show the coordinates (x and y).
Choose your own scale and show it in the block diagram.
Create a block diagram based on the algorithm.
Write the program code in Python.
Requirements:
Each step in the block diagram must be clearly shown.
The graph of the function must be drawn and saved (in PNG format).
Write the code in a modular way (functions and the main part should be separate).
Please explain and describe the results in detail.
Based on the given problem, create an algorithm and a block diagram, and write the program code:
Function: y=xsinx
Interval: [0,π]
Requirements:
Create a graph of the function.
Show the coordinates (x and y).
Choose your own scale and show it in the block diagram.
Create a block diagram based on the algorithm.
Write the program code in Python.
Requirements:
Each step in the block diagram must be clearly shown.
The graph of the function must be drawn and saved (in PNG format).
Write the code in a modular way (functions and the main part should be separate).
Please explain and describe the results in detail.
Chapter 15 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 15.3 - Prob. 15.1CPCh. 15.3 - Prob. 15.2CPCh. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - How can you tell from looking at a class...Ch. 15.3 - What makes an abstract class different from other...Ch. 15.3 - Examine the following classes. The table lists the...Ch. 15 - A class that cannot be instantiated is a(n) _____...
Ch. 15 - A member function of a class that is not...Ch. 15 - A class with at least one pure virtual member...Ch. 15 - In order to use dynamic binding, a member function...Ch. 15 - Static binding takes place at _____ time.Ch. 15 - Prob. 6RQECh. 15 - Prob. 7RQECh. 15 - Prob. 8RQECh. 15 - The is-a relation between classes is best...Ch. 15 - The has-a relation between classes is best...Ch. 15 - If every C1 class object can be used as a C2 class...Ch. 15 - A collection of abstract classes defining an...Ch. 15 - The keyword _____ prevents a virtual member...Ch. 15 - To have the compiler check that a virtual member...Ch. 15 - C++ Language Elements Suppose that the classes Dog...Ch. 15 - Will the statement pAnimal = new Cat; compile?Ch. 15 - Will the statement pCreature = new Dog ; compile?Ch. 15 - Will the statement pCat = new Animal; compile?Ch. 15 - Rewrite the following two statements to get them...Ch. 15 - Prob. 20RQECh. 15 - Find all errors in the following fragment of code,...Ch. 15 - Soft Skills 22. Suppose that you need to have a...Ch. 15 - Prob. 1PCCh. 15 - Prob. 2PCCh. 15 - Sequence Sum A sequence of integers such as 1, 3,...Ch. 15 - Prob. 4PCCh. 15 - File Filter A file filter reads an input file,...Ch. 15 - Prob. 6PCCh. 15 - Bumper Shapes Write a program that creates two...Ch. 15 - Bow Tie In Tying It All Together, we defined a...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Question: Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forward23:12 Chegg content://org.teleg + 5G 5G 80% New question A feed of 60 mol% methanol in water at 1 atm is to be separated by dislation into a liquid distilate containing 98 mol% methanol and a bottom containing 96 mol% water. Enthalpy and equilibrium data for the mixture at 1 atm are given in Table Q2 below. Ask an expert (a) Devise a procedure, using the enthalpy-concentration diagram, to determine the minimum number of equilibrium trays for the condition of total reflux and the required separation. Show individual equilibrium trays using the the lines. Comment on why the value is Independent of the food condition. Recent My stuff Mol% MeOH, Saturated vapour Table Q2 Methanol-water vapour liquid equilibrium and enthalpy data for 1 atm Enthalpy above C˚C Equilibrium dala Mol% MeOH in Saturated liquid TC kJ mol T. "Chk kot) Liquid T, "C 0.0 100.0 48.195 100.0 7.536 0.0 0.0 100.0 5.0 90.9 47,730 928 7,141 2.0 13.4 96.4 Perks 10.0 97.7 47,311 87.7 8,862 4.0 23.0 93.5 16.0 96.2 46,892 84.4…arrow_forwardYou are working with a database table that contains customer data. The table includes columns about customer location such as city, state, and country. You want to retrieve the first 3 letters of each country name. You decide to use the SUBSTR function to retrieve the first 3 letters of each country name, and use the AS command to store the result in a new column called new_country. You write the SQL query below. Add a statement to your SQL query that will retrieve the first 3 letters of each country name and store the result in a new column as new_country.arrow_forward
- We are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7). A code breaker manages to factories 247 = 13 x 19 Determine Alice's secret key. To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.arrow_forwardConsider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.arrow_forwardPllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forward
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
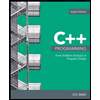
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
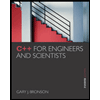
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
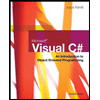
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
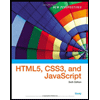
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
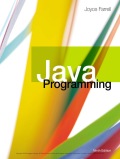
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT