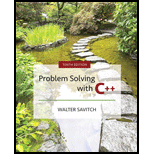
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 14.3, Problem 15STE
Explanation of Solution
Recursive function definition for “squares” function:
The recursive function definition for “squares” function is shown below:
//Function definition for "squares" function
int squares(int n)
{
/* If "n" is less than or equal to "1" */
if (n <= 1)
//Returns "1"
return 1;
//Otherwise
else
/* Recursively call the "squares" function with subtracting the value of "n" by "1" and then add and multiplied by "n" */
return (squares(n-1) + n * n);
}
Explanation:
The above function is used to compute the sum of the squares of numbers from “1” to “n”.
- In this function, first check the value of “n”. If the value of “n” is less than or equal to “1”, then returns “1”.
- Otherwise, recursively call the “squares” function with subtracting the value of “n” by “1” and then add and multiplied by “n”...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
What should the next three steps be in my machine based home security system after deployment and after the following current steps:
Enhancing Security & Privacy Measures
User Interface (UI) and Experience (UX) Improvement
Machine Learning Model Refinement & Continuous Improvement
I am creating a machine learning home based security system, have completed initial deployment and in the following phases of the project: Expanding device compatibility and integration, preparing for cloud integration, and implementing system reduncancy and disaster recovery. What should the next three phases be?
Hands-On Assignments Part II
Assignment 1-5: Querying the DoGood Donor Database
Review the DoGood Donor data by writing and running SQL statements to perform the following
tasks:
1. List each donor who has made a pledge and indicated a single lump sum payment. Include
first name, last name, pledge date, and pledge amount.
2. List each donor who has made a pledge and indicated monthly payments over one year.
Include first name, last name, pledge date, and pledge amount. Also, display the monthly
payment amount. (Equal monthly payments are made for all pledges paid in monthly
payments.)
3. Display an unduplicated list of projects (ID and name) that have pledges committed. Don't
display all projects defined; list only those that have pledges assigned.
4. Display the number of pledges made by each donor. Include the donor ID, first name, last
name, and number of pledges.
5. Display all pledges made before March 8, 2012. Include all column data from the
DD PLEDGE table.
Chapter 14 Solutions
Problem Solving with C++ (10th Edition)
Ch. 14.1 - Prob. 1STECh. 14.1 - Prob. 2STECh. 14.1 - Prob. 3STECh. 14.1 - Prob. 4STECh. 14.1 - Prob. 5STECh. 14.1 - If your program produces an error message that...Ch. 14.1 - Write an iterative version of the function cheers...Ch. 14.1 - Write an iterative version of the function defined...Ch. 14.1 - Prob. 9STECh. 14.1 - Trace the recursive solution you made to Self-Test...
Ch. 14.1 - Trace the recursive solution you made to Self-Test...Ch. 14.2 - What is the output of the following program?...Ch. 14.2 - Prob. 13STECh. 14.2 - Redefine the function power so that it also works...Ch. 14.3 - Prob. 15STECh. 14.3 - Write an iterative version of the one-argument...Ch. 14 - Prob. 1PCh. 14 - Prob. 2PCh. 14 - Write a recursive version of the search function...Ch. 14 - Prob. 4PCh. 14 - Prob. 5PCh. 14 - The formula for computing the number of ways of...Ch. 14 - Write a recursive function that has an argument...Ch. 14 - Prob. 3PPCh. 14 - Prob. 4PPCh. 14 - Prob. 5PPCh. 14 - The game of Jump It consists of a board with n...Ch. 14 - Prob. 7PPCh. 14 - Prob. 8PP
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a FancyCar class to support basic operations such as drive, add gas, honk horn, and start engine. FancyCar.java is provided with method stubs. Follow each step to gradually complete all methods. Note: This program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress. The main() method includes basic method calls. Add statements in main() as methods are completed to support development mode testing. Step 0. Declare private fields for miles driven as shown on the odometer (int), gallons of gas in tank (double), miles per gallon or MPG (double), driving capacity (double), and car model (String). Note the provided final variable indicates the gas tank capacity of 14.0 gallons. Step 1 (2 pts). 1) Complete the default constructor by initializing the odometer to five miles, tank is full of gas, miles per gallon is 24.0, and the model is "Old Clunker". 2)…arrow_forwardFind the error: daily_sales = [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0] days_of_week = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] for i in range(7): daily_sales[i] = float(input('Enter the sales for ' \ + day_of_week[i] + ': ')arrow_forwardFind the error: daily_sales = [0.0, 0,0, 0.0, 0.0, 0.0, 0.0, 0.0] days_of_week = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] for i in range(7): daily_sales[i] = float(input('Enter the sales for ' \ + days_of_week[i] + ': ')arrow_forward
- Find the error: daily_sales = [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0] days_of_week = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] for i in range(6): daily_sales[i] = float(input('Enter the sales for ' \ + days_of_week[i] + ': '))arrow_forwardWhat are the steps you will follow in order to check the database and fix any problems with it and normalize it? Give two references with your answer.arrow_forwardWhat are the steps you will follow in order to check the database and fix any problems with it? Have in mind that you SHOULD normalize it as well. Consider that the database offline is not allowed since people are connected to it and personal data might be bridged and not secured. Provide three refernces with you answer.arrow_forward
- Should software manufacturers should be tolerant of the practice of software piracy in third-world countries to allow these countries an opportunity to move more quickly into the information age? Why or why not?arrow_forwardI would like to know about the features of Advanced Threat Protection (ATP), AMD-V, and domain name space (DNS).arrow_forwardPlease show the code for the Tikz figurearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
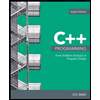
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
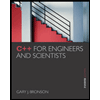
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
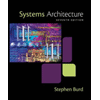
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning